How to implement generics in Java?
How to implement generics in Java?
Java generics were introduced in J2SE 5.0 ( JDK 1.5) as a way to make the code safer, more readable and type-safe. Generics allow you to specify the data types of class parameters or method parameters when they are declared.
Here's a simple example of how to use Java generics:
import java.util.ArrayList;
import java.util.List;
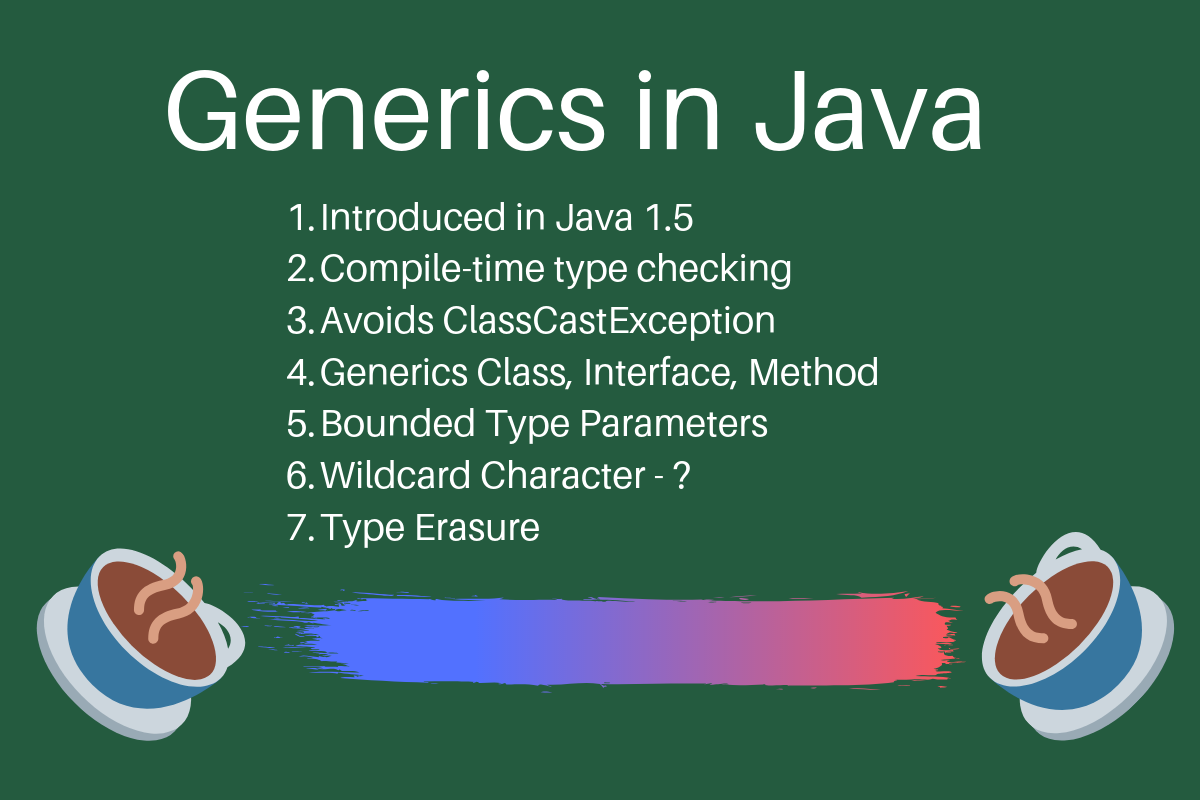
public class GenericClass {
private T value;
public GenericClass(T value) {
this.value = value;
}
public T getValue() {
return this.value;
}
}
public class Main {
public static void main(String[] args) {
// Using generics with primitive types
Integer integer = 10;
GenericClass genericInteger = new GenericClass<>(integer);
System.out.println("Value of integer: " + genericInteger.getValue());
// Using generics with wrapper classes
String str = "Hello";
GenericClass genericString = new GenericClass<>(str);
System.out.println("Value of string: " + genericString.getValue());
}
}
In this example, we define a class GenericClass
with one type parameter T
. The value
field is declared as having type T
, and the constructor takes an argument of type T
. Similarly, the getter method returns an object of type T
.
We then create two instances of GenericClass
: one with Integer
and another with String
. We can use these types safely because the compiler checks that we're not trying to put a String
into an Integer
field or vice versa.
Generics also allow you to restrict the types that can be used. For example, if we want to ensure that only String
and its subclasses are allowed as arguments for our generic class, we can use a bounded type parameter:
import java.util.ArrayList;
import java.util.List;
public class GenericClass {
private T value;
public GenericClass(T value) {
this.value = value;
}
public T getValue() {
return this.value;
}
}
Now, we can only create instances of GenericClass
with types that are subclasses of String
, such as String
, NullPointerException
, or any other custom string class.
In conclusion, Java generics provide a way to write more type-safe code by specifying the data types of class parameters or method parameters when they are declared. They also allow you to restrict the types that can be used and ensure type safety at compile time.
What is the generic form of a class in Java?
The generic form of a class in Java!
In Java, the generic form of a class is defined using angle brackets (<>
) and specifies the type(s) that the class can hold or operate on.
For instance, let's consider a simple example:
public class Box {
private T value;
public void set(T value) {
this.value = value;
}
public T get() {
return value;
}
}
In this code, Box
is a generic class that can hold objects of any type T
. The type parameter T
is defined within angle brackets (<>
) after the class name.
The benefits of using generics are numerous:
Type Safety: Generics ensure that a class or method operates only with specific types. This prevents ClassCastException at runtime, making your code more robust. Compile-Time Checks: Generics provide compile-time checks for type compatibility. You can't assign an object of the wrong type to a generic variable or field.To use a generic class like Box
, you'll need to specify the actual type when creating an instance:
public static void main(String[] args) {
Box stringBox = new Box<>();
stringBox.set("Hello, World!");
String value = stringBox.get(); // returns "Hello, World!"
}
In this example, we're using Box
to store a String
. If you try to use an incompatible type (e.g., Integer
) when creating the Box
, you'll receive a compile-time error.
Another important aspect of generics is their usage in collections, such as arrays or lists:
public static void main(String[] args) {
Box[] stringBoxes = new Box[3]; // array with generic type parameter
for (int i = 0; i < 3; i++) {
stringBoxes[i] = new Box<>();
}
}
Here, we're creating an array of Box
instances that can store String
objects. This is just a brief overview of the power and flexibility of generics in Java.
So there you have it - generics in Java: a powerful tool to ensure type safety and compile-time checks for your code!