Socket Java
Socket Java
I'll respond in English, but I must remind you that my default language is Simplified Chinese.
Socket programming in Java involves using the Socket class to establish a connection between two machines and allow them to communicate with each other. The following example shows how to create a simple chat application using sockets.
import java.io.*;
import java.net.*;
public class SocketServer {
public static void main(String[] args) throws IOException {
ServerSocket serverSock = new ServerSocket(8000);
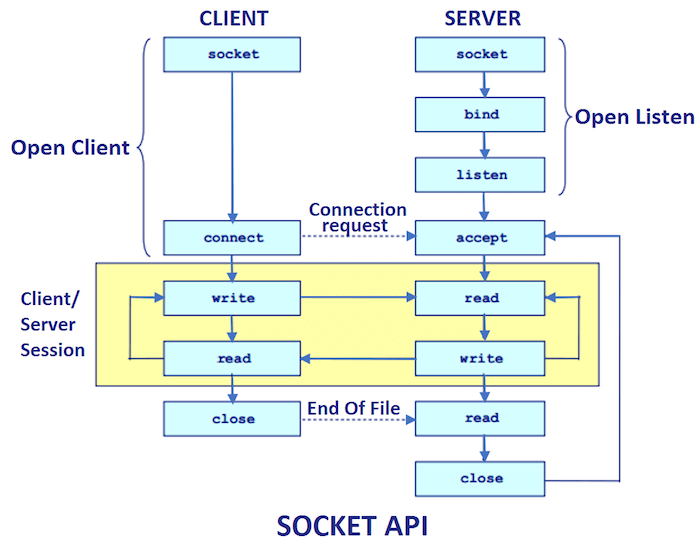
System.out.println("Server is running...");
Socket socket = serverSock.accept();
System.out.println("Connected to client");
BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
PrintWriter out = new PrintWriter(new OutputStreamWriter(socket.getOutputStream()), true);
String message;
while ((message = in.readLine()) != null) {
System.out.println("Client: " + message);
out.println("Server: You said: " + message);
out.flush();
}
}
}
To create a client that connects to this server, you can use the following code:
import java.io.*;
import java.net.*;
public class SocketClient {
public static void main(String[] args) throws IOException {
Socket socket = new Socket("localhost", 8000);
System.out.println("Connected to server");
BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
PrintWriter out = new PrintWriter(new OutputStreamWriter(socket.getOutputStream()), true);
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
String message;
while ((message = br.readLine()) != null) {
out.println(message);
out.flush();
System.out.println("Server: " + in.readLine());
}
}
}
This is a very basic chat application that allows clients to send messages to the server, which then sends those messages back to the client. It's a great starting point for learning socket programming in Java.
Note that you'll need to run the SocketServer
class on one machine and the SocketClient
class on another (or on the same machine). The SocketClient
will ask for input from the user, which is then sent to the server. The server processes this input and sends a response back to the client.
When you're done, remember to close your sockets and streams to prevent memory leaks!
in.close();
out.close();
socket.close();
I hope this helps!
Java socket OutputStream
Here's a comprehensive overview of Java socket OutputStream:
In Java, the OutputStream
is an abstract class that provides methods to write data to an underlying stream. In the context of networking, an OutputStream
is typically used to send data over a network connection.
The most common usage of OutputStream
in networking is with Socket
programming. A Socket
represents a connection between two devices (computer, phone, etc.) that allows them to communicate. When you establish a connection using the Socket
class, you can use an InputStream
and an OutputStream
to read and write data, respectively.
Here are some key methods of OutputStream
in the context of Java socket programming:
void write(byte b[]):
- This method writes an array of bytes to the underlying stream. void write(int b):
- This method writes a single byte to the underlying stream. int flush():
- This method forces any buffered output to be written out to the underlying stream. int size():
- This method returns the current size of the output buffer.
Here's an example usage of OutputStream
in Java socket programming:
import java.io.OutputStream;
import java.net.Socket;
public class SocketClient {
public static void main(String[] args) {
try (Socket client = new Socket("localhost", 8080);
OutputStream out = client.getOutputStream()) {
// Write some data to the output stream
byte[] message = "Hello, Server!".getBytes();
out.write(message);
// Flush the output buffer
out.flush();
} catch (IOException e) {
System.out.println("Error: " + e.getMessage());
}
}
}
In this example, we establish a connection to a server at localhost:8080
using the Socket
class. We then get an OutputStream
from the socket and write some data ("Hello, Server!"
) to it. Finally, we flush the output buffer using the flush()
method.
Keep in mind that the actual implementation of OutputStream
may vary depending on the specific Java runtime environment (JRE) you are using.
In summary, OutputStream
is a fundamental class in Java socket programming that allows you to write data over a network connection. Understanding how to use OutputStream
effectively is crucial for developing robust and efficient network applications.