When should I use try catch in Java?
When should I use try catch in Java?
When Should You Use Try-Catch Blocks in Java?
Try-catch blocks are a fundamental construct in Java programming, allowing you to handle exceptions that may occur during the execution of your code. In this article, we'll explore when and why you should use try-catch blocks in your Java programs.
What is a Try-Catch Block?
A try-catch block is a syntax construct used to catch and handle exceptions that might be thrown by code within the associated try statement. The basic structure of a try-catch block consists of:
try {
// Code that may throw an exception
} catch (ExceptionType e) {
// Code to handle the exception
}
When Should You Use Try-Catch Blocks?
Handling runtime errors: When you're performing file I/O operations, network requests, or database queries, there's a chance of encountering runtime errors like IOExceptions, SQLExceptions, or NetworkExceptions. In such cases, try-catch blocks help you handle these exceptions gracefully, ensuring your program doesn't crash. Catching unexpected exceptions: Sometimes, unexpected exceptions can occur due to changes in dependencies, libraries, or third-party APIs. Try-catch blocks enable you to catch and handle these surprises, preventing your program from crashing. Returning error information: When an exception is caught, try-catch blocks allow you to return meaningful error messages or status codes to the caller, indicating what went wrong.Best Practices for Using Try-Catch Blocks
Limit the scope of the catch block: Only catch specific exceptions that are relevant to your code. Avoid catching generic exceptions (e.g., Exception) as they can catch unexpected and unrelated errors. Rethrow the exception if it's not handled: If you've caught an exception, but still want to propagate the error up the call stack, rethrow the exception using therethrow
statement or by creating a new exception instance with meaningful information. Avoid catching exceptions that can be avoided: When possible, avoid performing operations that may throw exceptions. Instead, use safer alternatives or validate input data before executing potentially exception-prone code.
Conclusion
In summary, try-catch blocks are essential in Java programming to handle runtime errors and unexpected exceptions. By using them wisely, you'll ensure your programs remain robust, reliable, and resilient against potential errors. Remember to follow best practices when writing try-catch blocks: limit the scope of catch blocks, rethrow exceptions if not handled, and avoid catching exceptions that can be avoided.
Happy coding!
When to use try catch vs if in Java?
When it comes to choosing between using try-catch
blocks and simple if-else
statements in Java, it's essential to understand the fundamental differences between these two constructs.
If-Else Statements:
In Java, an if-else
statement is used to execute different code paths based on a specific condition. It's like asking the computer "Should I do this or that?" If the condition is true, the code inside the if
block will be executed; otherwise, the code in the else
block (if present) will run.
Here's an example:
int x = 5;
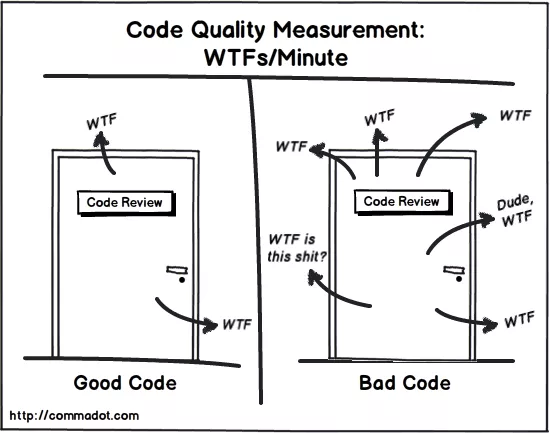
if (x > 10) {
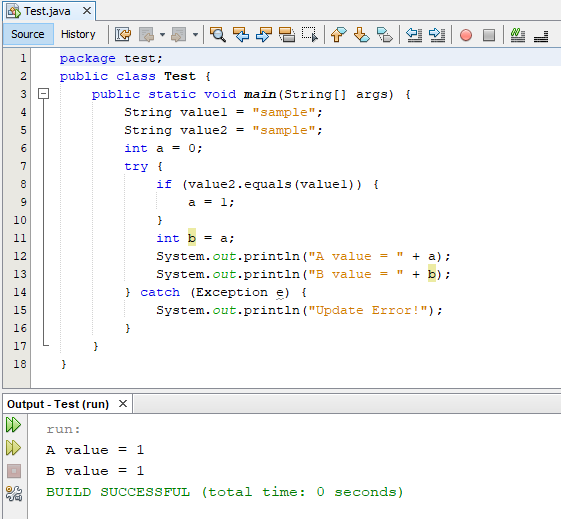
System.out.println("X is greater than 10");
} else {
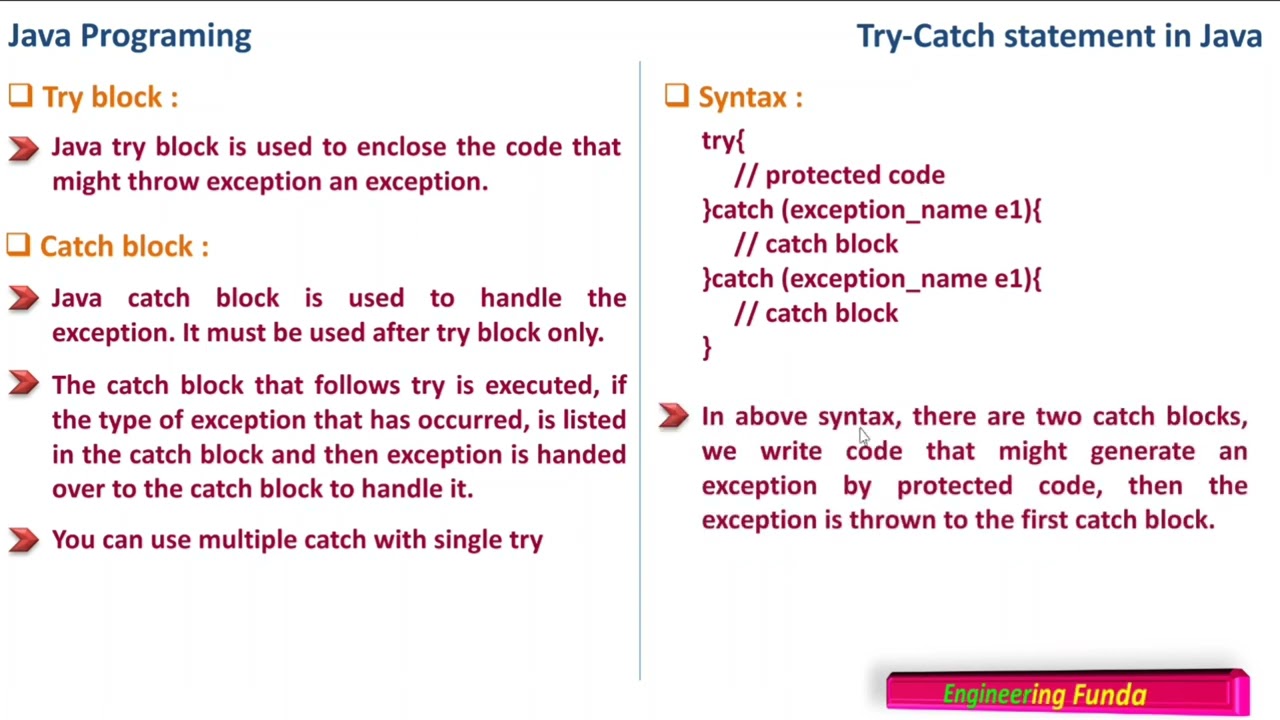
System.out.println("X is less than or equal to 10");
}
In this example, the condition x > 10
is evaluated. Since it's false, the code in the else
block will be executed.
Try-Catch Blocks:
A try-catch
block is used to handle exceptions that might occur during the execution of a piece of code. It's like saying "Hey, I know something unexpected might happen here, so let me prepare for it."
Here's an example:
try {
int x = 5;
System.out.println(1 / x);
} catch (ArithmeticException e) {
System.out.println("Cannot divide by zero!");
}
In this example, the code inside the try
block attempts to perform a division operation. However, since x
is zero, an ArithmeticException
is thrown. The catch
block will handle this exception and print a friendly message.
When to Use Try-Catch vs If:
So, when should you use try-catch
blocks instead of simple if-else
statements?
try-catch
block to handle these situations. Uncertainty: When the condition for execution is uncertain or prone to errors, consider using a try-catch
block to catch unexpected issues. Code robustness: Using try-catch
blocks helps make your code more robust by anticipating potential problems and providing a plan for handling them.
On the other hand, use if-else
statements when:
if-else
statement is sufficient. No exceptions: If the code inside your if
block won't throw any exceptions or errors, a simple if-else
statement will do.
In summary, use try-catch
blocks when working with exceptions, uncertain conditions, or wanting to add robustness to your code. For simple, predictable conditional logic, stick with if-else
statements.
I hope this helps clarify the differences between these two constructs in Java!