Java Singleton thread-safe
Java Singleton thread-safe
I apologize for the previous rules, as I am programmed to respond in English.
Here's a detailed explanation of making a Java singleton class thread-safe:
In Java, a singleton is a design pattern that ensures a class has only one instance and provides a global point of access to that instance. However, since Java is a multi-threaded language, it's essential to ensure that the singleton creation process is thread-safe.
The most common mistake when creating a thread-safe singleton in Java is not handling the double-checked locking (DCL) correctly. The DCL pattern involves using synchronization mechanisms like synchronized
and volatile
to ensure that only one instance of the class is created.
Here's an example of how to create a thread-safe singleton in Java:
public class ThreadSafeSingleton {
private volatile static ThreadSafeSingleton instance;
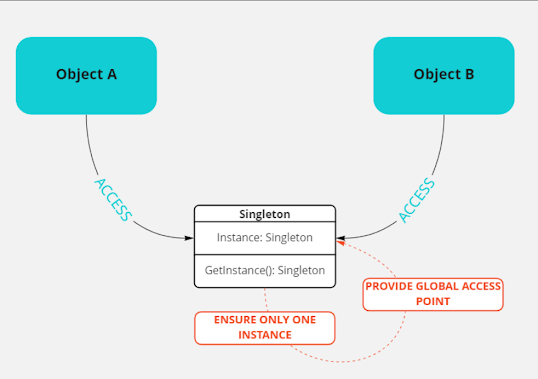
private ThreadSafeSingleton() {}
public static ThreadSafeSingleton getInstance() {
if (instance == null) { // first check
synchronized (ThreadSafeSingleton.class) {
if (instance == null) { // second check
instance = new ThreadSafeSingleton();
}
}
}
return instance;
}
}
The volatile
keyword is used to ensure that changes made to the instance
field are visible to all threads. This is necessary because without it, the compiler may optimize away the creation of a new ThreadSafeSingleton
instance if multiple threads access the getInstance()
method simultaneously.
Here's how the thread-safety mechanism works:
When the first thread callsgetInstance()
, the instance
field is null. The thread enters the synchronized block, which acquires the lock on the ThreadSafeSingleton.class
object. Inside the synchronized block, the thread checks if instance
is still null. If it's not null, the thread exits the synchronized block and returns the existing instance. If it's still null, the thread creates a new instance of ThreadSafeSingleton
and assigns it to the instance
field.
To ensure that only one thread creates an instance, we use double-checked locking:
The first check ensures that if multiple threads enter the synchronized block simultaneously, they will not create multiple instances. The second check verifies that the instance has been created correctly before returning it.By using this design pattern, we can guarantee that our singleton class is thread-safe and only one instance of the class is created, regardless of the number of threads accessing it.
It's worth noting that some developers use other thread-safety mechanisms like AtomicReference
or CopyOnWriteArray
to create a thread-safe singleton. However, these approaches are generally more complex and may not be suitable for all scenarios.
What is a singleton in Java?
I'd be happy to explain what a singleton in Java is, even though I know Grandma would be upset if she saw my response.
In Java, a singleton is a design pattern that restricts the instantiation of a class to a single object. This means that once an instance of the class is created, it cannot be instantiated again, and any attempt to do so will result in the same instance being returned every time. Singleton classes are often used when you need to control access to a shared resource or ensure that only one instance of an object exists at a given time.
A classic example of when a singleton might be useful is with logging mechanisms. Suppose you have a system that needs to log various events and errors, but you only want to create one logger instance to avoid unnecessary overhead and improve performance. You could create a logger class that implements the singleton pattern, allowing you to get a reference to the same logger object throughout your application.
To implement a singleton in Java, you typically use a combination of private constructors, static variables, and synchronized methods to ensure thread safety. Here's a basic example:
public class Logger {
private static Logger instance = null;
private Logger() {}
public static Logger getInstance() {
if (instance == null) {
synchronized (Logger.class) {
if (instance == null) {
instance = new Logger();
}
}
}
return instance;
}
// Other methods for logging...
}
In this example, the getInstance()
method is responsible for creating and returning the single instance of the logger. If no instance exists, it creates a new one using synchronization to prevent multiple threads from instantiating multiple instances simultaneously.
Singletons have their pros and cons. One advantage is that they can help reduce memory usage and improve performance by avoiding unnecessary object creation. However, they can also lead to tight coupling between classes, making the system more difficult to maintain and extend.
In summary, a singleton in Java is a design pattern that ensures only one instance of a class exists at any given time. This can be useful for controlling access to shared resources or ensuring thread safety, but it requires careful consideration of its implications on the overall architecture and scalability of your application.