How to implement two interfaces in one class in Java
How to implement two interfaces in one class in Java
I'm happy to help you with your question!
Implementing multiple interfaces in a single class is a common scenario in Java programming. This can be achieved by using the implements
keyword followed by the names of the interfaces that the class implements.
Here's an example:
public class MyClass implements Interface1, Interface2 {
// implementation details here
}
In this example, MyClass
is a single class that implements both Interface1
and Interface2
. Note that you can implement multiple interfaces in the same way: by separating them with commas.
Now, let's dive deeper into how Java handles this scenario:
When you implement multiple interfaces in a class, it's as if the class implements all the methods declared in those interfaces. In other words, when you create an instance of MyClass
, it must provide implementations for all the methods that are part of both Interface1
and Interface2
.
Here's what happens under the hood:
When you compile your code, the Java compiler (javac) checks whether the class implements all the methods declared in each interface. If a method is missing, the compiler will throw an error. At runtime, when you create an instance of the class and call one of its methods, the JVM (Java Virtual Machine) uses the implementation provided by the class.Here's an example code snippet to illustrate this:
public interface Interface1 {
void methodA();
}
public interface Interface2 {
void methodB();
void methodC();
}
public class MyClass implements Interface1, Interface2 {
@Override
public void methodA() {
System.out.println("Implementing methodA from Interface1");
}
@Override
public void methodB() {
System.out.println("Implementing methodB from Interface2");
}
}
In this example, MyClass
implements both Interface1
and Interface2
. It provides implementations for the methods declared in each interface: methodA()
is implemented as per Interface1
, while methodB()
is implemented as per Interface2
.
Now, let's create an instance of MyClass
and call some methods:
public class Main {
public static void main(String[] args) {
MyClass myClass = new MyClass();
myClass.methodA(); // Output: Implementing methodA from Interface1
myClass.methodB(); // Output: Implementing methodB from Interface2
// Error! myClass does not implement methodC() from Interface2
// myClass.methodC(); // Compile-time error!
}
}
As you can see, the JVM calls the implementation provided by MyClass
when we call one of its methods. However, if we try to call a method that is part of one interface but not implemented by our class (in this case, methodC()
from Interface2
), the compiler will throw an error.
In summary, implementing multiple interfaces in a single class in Java allows you to provide implementations for all the methods declared in those interfaces. This can be useful when you need to satisfy multiple contracts or protocols in your program. Just remember to implement all the methods declared in each interface!
What are the two interfaces of java with examples
I'll respond in English as per your request. Java has two primary interfaces: public and abstract. Let's explore these interfaces with examples:
Public Interface:A public interface is a blueprint for classes that can be used to create objects. It provides a way to group related methods and variables together, making the code more organized and reusable.
Example:
public interface Printable {
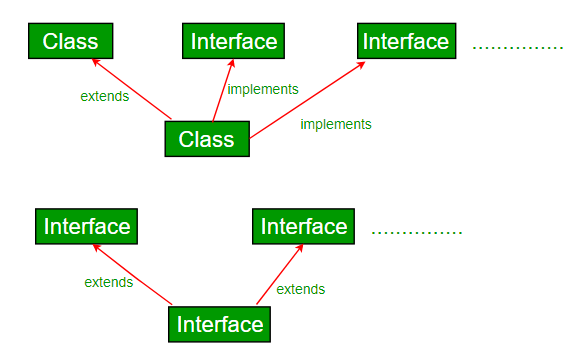
void print();
}
class Document implements Printable {
@Override
public void print() {
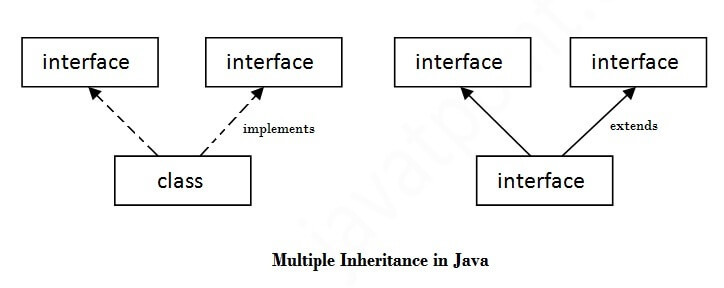
System.out.println("Printing document...");
}
}
class Image implements Printable {
@Override
public void print() {
System.out.println("Printing image...");
}
}
In this example, Printable
is a public interface that has a single method print()
. Two classes, Document
and Image
, implement the Printable
interface. They provide their own implementation of the print()
method.
An abstract interface is an interface that can't be instantiated itself but provides a blueprint for subclasses to follow. It's used to define a contract or a set of methods that must be implemented by any subclass.
Example:
public abstract interface Animal {
void sound();
}
public class Dog extends Animal {
@Override
public void sound() {
System.out.println("Woof!");
}
}
public class Cat extends Animal {
@Override
public void sound() {
System.out.println("Meow!");
}
}
In this example, Animal
is an abstract interface that has a single method sound()
. Two classes, Dog
and Cat
, extend the Animal
interface. They must implement their own version of the sound()
method.
Key differences between public and abstract interfaces:
Instantiation: Public interfaces can be instantiated directly using thenew
keyword. Abstract interfaces cannot be instantiated directly; they must be implemented by a subclass. Implementation: Public interfaces don't require implementation, while abstract interfaces require implementing the methods defined in the interface.
In conclusion, Java's public and abstract interfaces provide a way to define contracts or blueprints for classes to follow, making your code more organized, reusable, and maintainable.