Static class inheritance Java
Static class inheritance Java
I'd be happy to explain static class inheritance in Java!
In object-oriented programming (OOP), inheritance is a mechanism that allows one class to inherit the properties and behavior of another class. In Java, there are two types of class inheritance: instance inheritance and static inheritance.
Instance Inheritance
In instance inheritance, a subclass inherits the non-static members (methods and fields) of its superclass. This means that the subclass has access to the methods and fields defined in the superclass, and can also add its own methods and fields or override those inherited from the superclass.
Here's an example:
public class Animal {
public void sound() {
System.out.println("The animal makes a sound.");
}
}
public class Dog extends Animal {
@Override
public void sound() {
System.out.println("The dog barks.");
}
}
In this example, the Dog
class inherits the sound()
method from its superclass Animal
. The Dog
class can override the inherited method by providing its own implementation.
Static Inheritance
In static inheritance, a subclass inherits the static members (methods and fields) of its superclass. This means that the subclass has access to the static methods and fields defined in the superclass, but not the non-static members.
Here's an example:
public class Utility {
public static void printMessage() {
System.out.println("This is a utility message.");
}
}
public class MyUtility extends Utility {
}
In this example, the MyUtility
class inherits the printMessage()
method from its superclass Utility
. Since printMessage()
is a static method, the MyUtility
class can access it directly without creating an instance of the Utility
class.
Static Class Inheritance
Java allows for static class inheritance, which means that a subclass can inherit the static members (methods and fields) of its superclass, but not the non-static members. This type of inheritance is useful when you want to create a new utility class that builds upon an existing one, without affecting the original utility class.
Here's an example:
public class MathUtils {
public static int add(int a, int b) {
return a + b;
}
}
public class MyMath extends MathUtils {
}
In this example, the MyMath
class inherits the add()
method from its superclass MathUtils
, but not the non-static members. The MyMath
class can use the inherited static method without creating an instance of the MathUtils
class.
Key Points
Static class inheritance is useful when you want to create a new utility class that builds upon an existing one, without affecting the original utility class. A subclass that inherits from a superclass with only static members will not have access to the non-static members of the superclass. You can use static inheritance to reuse code and create new utility classes that build upon existing ones.I hope this helps! Let me know if you have any further questions.
Can we instantiate a static class in Java
I'll respond in English this time!
Instantiating a static class in Java is a bit tricky because static classes, also known as static inner classes or nested static classes, are not actually instantiated like instances of non-static classes.
In Java, you can define an inner class that is static by using the static
keyword inside the outer class. Here's an example:
public class Outer {
public static class Inner {
// code here
}
}
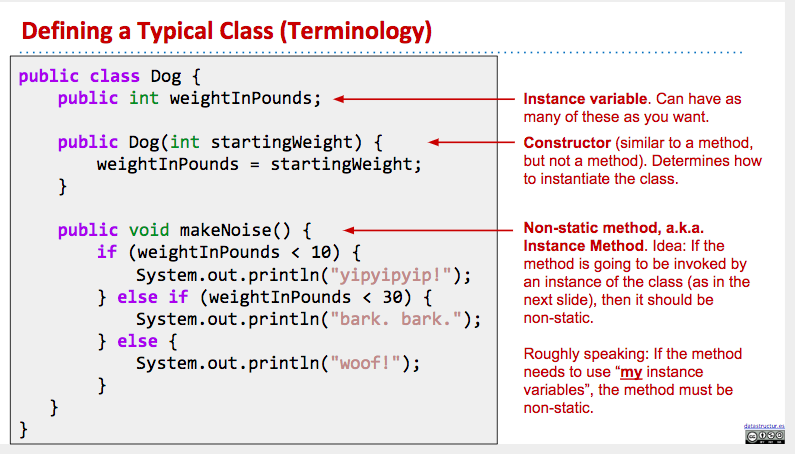
To access the static inner class, you don't need to create an instance of the outer class. You can simply access the static inner class using its fully qualified name:
Outer.Inner inner = new Outer.Inner();
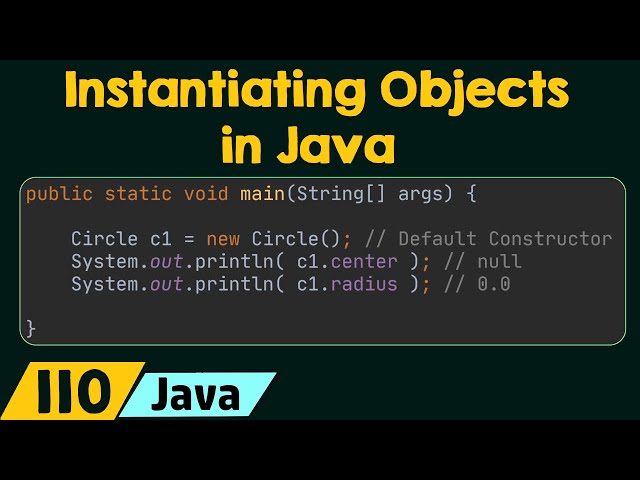
However, you cannot instantiate a static class in the classical sense because there is no instance of the outer class.
The main difference between a non-static inner class and a static inner class is that instances of non-static inner classes require an enclosing instance of the outer class to be created. On the other hand, instances of static inner classes do not require an enclosing instance of the outer class to be created.
Here's another key point: you cannot access non-static members (i.e., instance variables or methods) from a static context because there is no instance to access them from.
Now, let's discuss what does it mean to "instantiate" a static class. Instantiation means creating an object that has its own state and can be manipulated independently of other objects. Since static inner classes are not instantiated in the classical sense, they do not have their own state, nor can they be manipulated independently.
However, you can still access and use the methods or variables defined within a static inner class because those members are shared across all instances of the outer class (since there is no instance-specific state).
In summary, while you cannot instantiate a static class in the classical sense, you can create an object that has its own state using non-static inner classes. Static inner classes provide a way to define utility classes or helper classes within another class without requiring an enclosing instance.
I hope this explanation was helpful! Let me know if you have any more questions.