Java concurrency tutorial for beginners
Java concurrency tutorial for beginners
I'd be happy to provide a comprehensive introduction to Java concurrency for beginners! Here's a detailed guide to get you started:
What is Concurrency?
Concurrency refers to the ability of multiple threads or processes to execute concurrently, improving system performance and responsiveness. In Java, concurrency is achieved using threads, which are lightweight and efficient.
Why Use Concurrency in Java?
Concurrent programming enables your program to:
Improve Performance: By executing tasks simultaneously, you can speed up overall execution time. Enhance Responsiveness: Concurrency allows your program to handle multiple requests or events concurrently, making it more responsive. Simplify Code: With concurrency, you can divide complex tasks into smaller, manageable parts, simplifying code and reducing complexity.Basic Concepts
Thread: A lightweight process that executes a specific task. Java uses thejava.lang.Thread
class to manage threads. Runnable: An interface that defines the run() method, which is called when a thread is started.
Concurrency Models
Java Concurrency APIs
java.lang.Thread: The core API for working with threads. java.util.concurrent: A package containing classes for concurrency management, such asExecutor
, ExecutorService
, and Futures
. java.util.concurrrent.atomic: A package providing atomic operations for updating shared variables.
Best Practices
Use Executors Wisely: Only create executors when necessary, to avoid overhead. Minimize Lock Contention: Use locking mechanisms wisely to avoid performance bottlenecks. Test Concurrency Carefully: Test your concurrency code thoroughly to catch any potential issues or deadlocks.Concurrency Challenges
Synchronization: Coordinating access to shared resources and ensuring thread safety. Communication: Sharing data between threads and handling inter-thread dependencies. Resource Management: Managing system resources, such as CPU, memory, and I/O devices, effectively.Conclusion
Java concurrency is a powerful tool for building efficient, scalable, and responsive applications. By understanding the basics of concurrency, Java's concurrency APIs, best practices, and challenges, you'll be well-equipped to tackle complex concurrency problems in your programming journey!
How to do concurrency in Java?
Concurrency in Java!
To achieve concurrency in Java, you can use various techniques and tools provided by the language itself. Here are some ways:
1. Threads: The most straightforward way is to create multiple threads that execute concurrently. You can use the Thread
class or implement a custom thread class. Each thread runs independently, sharing the same memory space.
public class ConcurrencyExample {
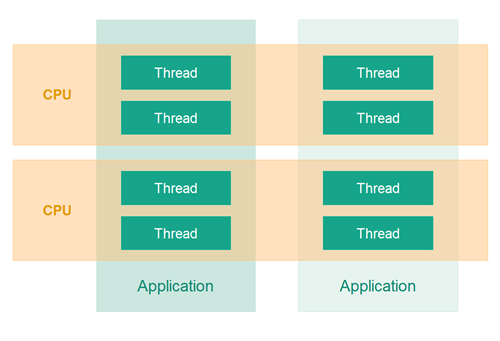
public static void main(String[] args) {
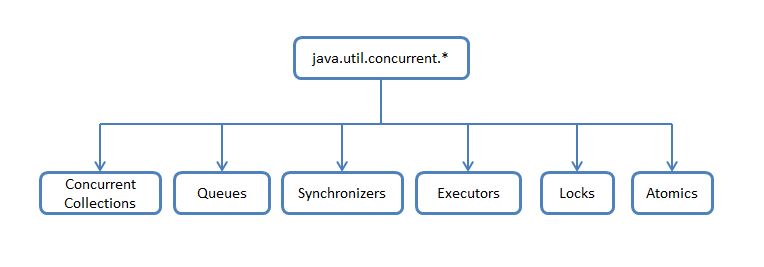
Thread t1 = new MyThread("Thread 1");
Thread t2 = new MyThread("Thread 2");
t1.start();
t2.start();
// wait for both threads to finish
try {
t1.join();
t2.join();
} catch (InterruptedException e) {
System.out.println("Interrupted!");
}
}
static class MyThread extends Thread {
public MyThread(String name) {
super(name);
}
@Override
public void run() {
for (int i = 0; i < 5; i++) {
System.out.println(getName() + ": " + i);
}
}
}
}
2. Executor Framework: This is a higher-level abstraction that simplifies thread creation and management. You can use an ExecutorService
to create, execute, and manage threads.
public class ConcurrencyExample {
public static void main(String[] args) {
// create a single-threaded executor service
ExecutorService executor = Executors.newSingleThreadExecutor();
// submit tasks for execution
Future<?> future1 = executor.submit(() -> {
for (int i = 0; i < 5; i++) {
System.out.println("Task 1: " + i);
}
});
Future<?> future2 = executor.submit(() -> {
for (int i = 0; i < 5; i++) {
System.out.println("Task 2: " + i);
}
});
// shut down the executor
executor.shutdown();
}
}
3. Java Concurrency Utilities (JCUP): This is a set of libraries that provide classes for executing tasks concurrently.
public class ConcurrencyExample {
public static void main(String[] args) {
// create a single-threaded executor service
ExecutorService executor = Executors.newSingleThreadExecutor();
// submit tasks for execution
executor.submit(new Callable() {
@Override
public Object call() {
for (int i = 0; i < 5; i++) {
System.out.println("Task: " + i);
}
return null;
}
});
// shut down the executor
executor.shutdown();
}
}
4. Futures: You can use Future
objects to execute tasks asynchronously and then retrieve the results.
public class ConcurrencyExample {
public static void main(String[] args) {
// create a single-threaded executor service
ExecutorService executor = Executors.newSingleThreadExecutor();
// submit tasks for execution
Future future = executor.submit(() -> "Hello, World!");
try {
System.out.println(future.get()); // prints: Hello, World!
} catch (InterruptedException e) {
System.out.println("Interrupted!");
}
// shut down the executor
executor.shutdown();
}
}
Remember to handle exceptions and thread interruptions properly, especially in production environments.
These are just a few examples of how you can achieve concurrency in Java. The choice of approach depends on your specific use case and requirements.