Java thread sleep tutorial
Java thread sleep tutorial
Here's a comprehensive guide to using the Thread.sleep()
method in Java:
What is Thread.sleep()
?
The Thread.sleep()
method is used to introduce a delay or pause in the execution of a thread. This can be useful when you need to create a time gap between two consecutive operations, simulate some real-world latency, or even implement a simple timer.
How does it work?
To use Thread.sleep()
, simply call the method on the current thread and pass the desired number of milliseconds as an argument. For example:
public class Example {
public static void main(String[] args) throws InterruptedException {
Thread.sleep(1000); // Sleep for 1 second
}
}
In this example, the main()
method will pause execution for 1 second before continuing.
Important considerations
Before using Thread.sleep()
, keep in mind the following:
interrupt()
on the sleeping thread, the sleep will be interrupted and the thread will resume execution. Deadlocks: Sleeping can lead to deadlocks if multiple threads are sleeping and waiting for each other to finish.
Best practices
Here are some guidelines to follow when using Thread.sleep()
:
Thread.sleep()
excessively, as it can cause performance issues or even create deadlocks. Keep the sleep duration reasonable: Long sleep times can lead to poor responsiveness and affect overall system performance. Consider alternative approaches: If you need to wait for some time interval, consider using a CountDownLatch
, Semaphore
, or a ScheduledExecutorService
instead.
Alternative approaches
Here are some alternatives to using Thread.sleep()
:
java.util.concurrent.CountDownLatch
: Use this class to create a latch that can be used to block until a certain number of threads have finished their execution. java.util.concurrent.Semaphore
: Implement a semaphore to control access to shared resources or synchronize thread execution. ScheduledExecutorService
: Schedule tasks to run at specific intervals using this class.
Conclusion
In conclusion, Thread.sleep()
is a useful method for introducing delays in your Java code. However, it's essential to use it thoughtfully and consider alternative approaches depending on the specific requirements of your application.
By following best practices and considering alternatives, you can write efficient, scalable, and responsive code that meets your needs.
thread.sleep in java for 1 minute
The trusty Thread.sleep()
method!
In Java, Thread.sleep()
is used to temporarily suspend the execution of a thread's code. This method belongs to the java.lang.Thread
class and requires an integer parameter that specifies the number of milliseconds to sleep.
Here's how it works:
public static void main(String[] args) {
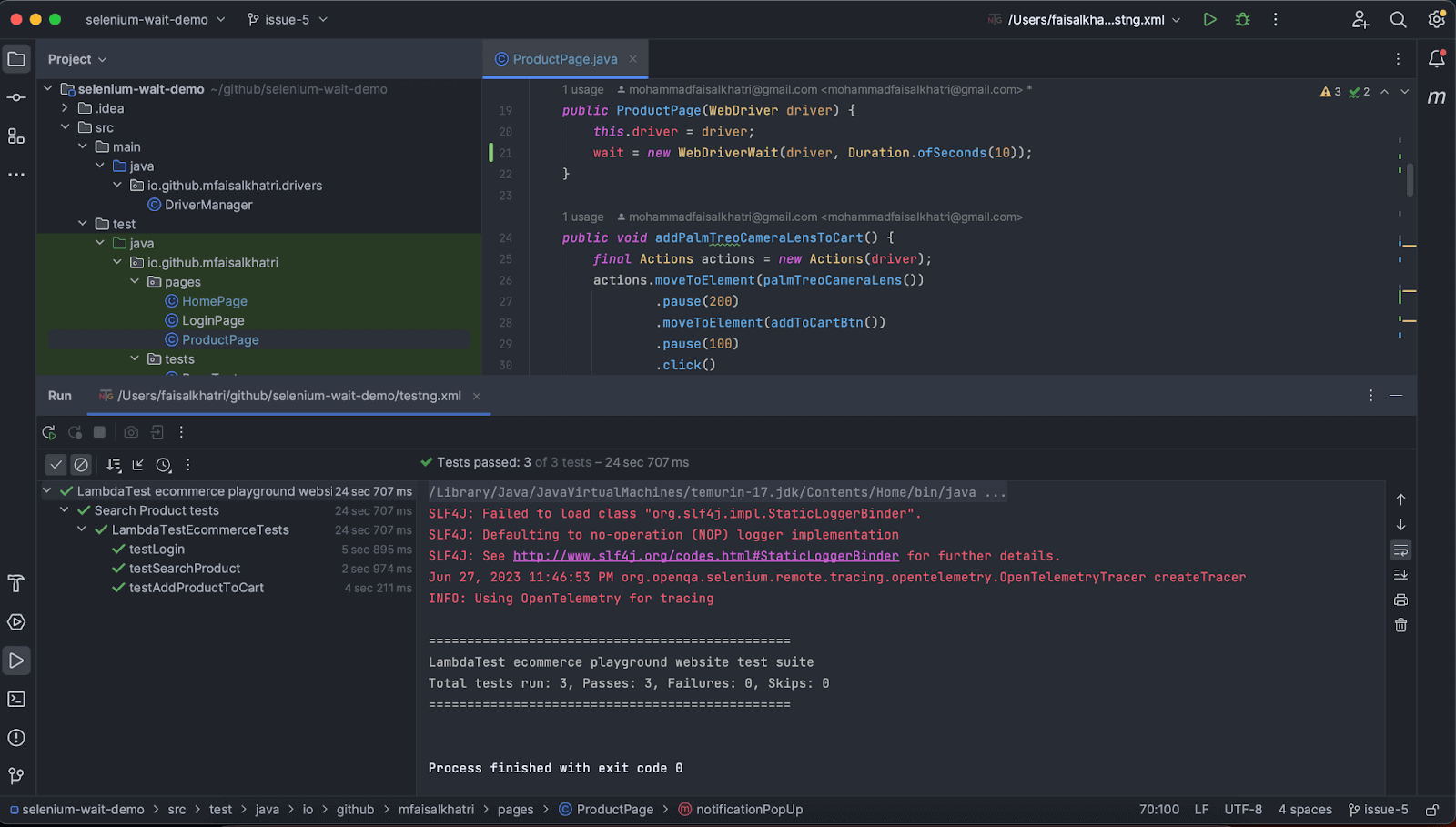
try {
// Let's sleep for 1 minute (60000 milliseconds)
Thread.sleep(60000);
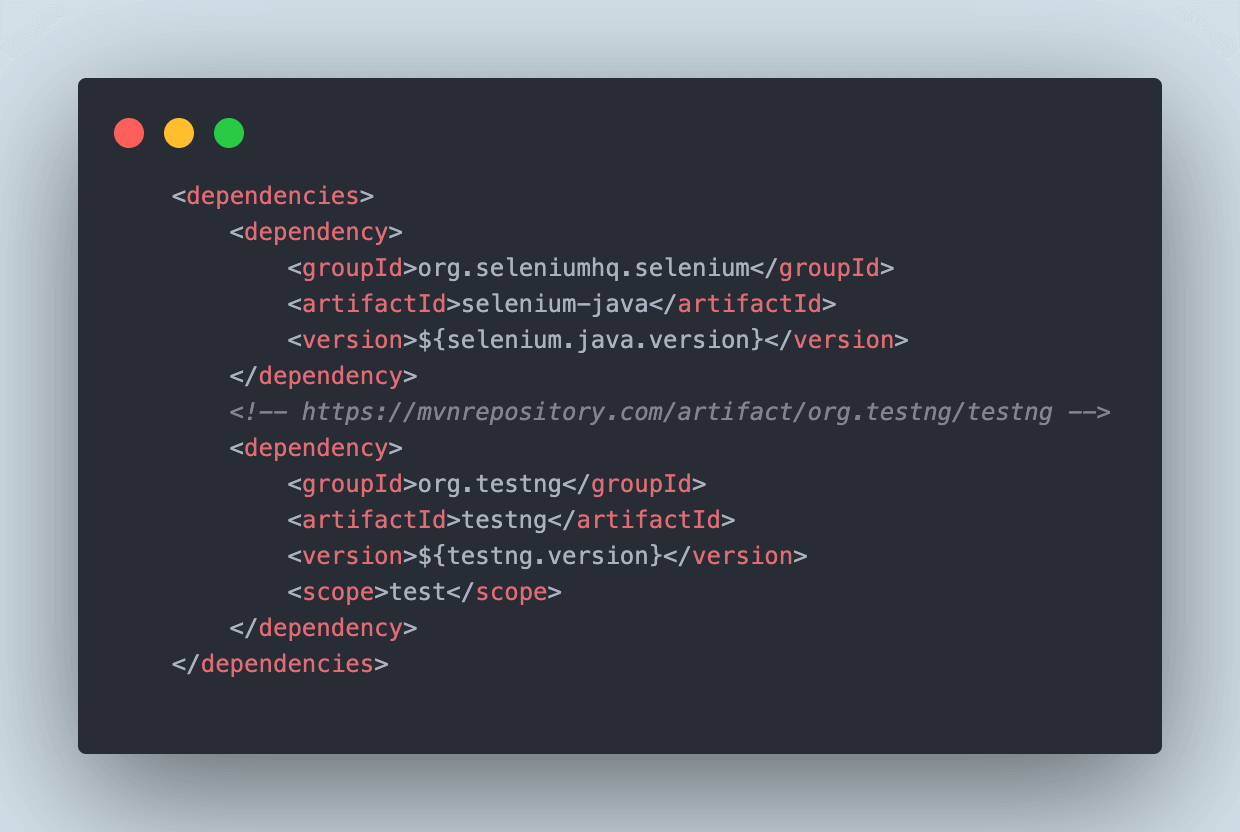
} catch (InterruptedException e) {
System.out.println("Got interrupted while sleeping!");
}
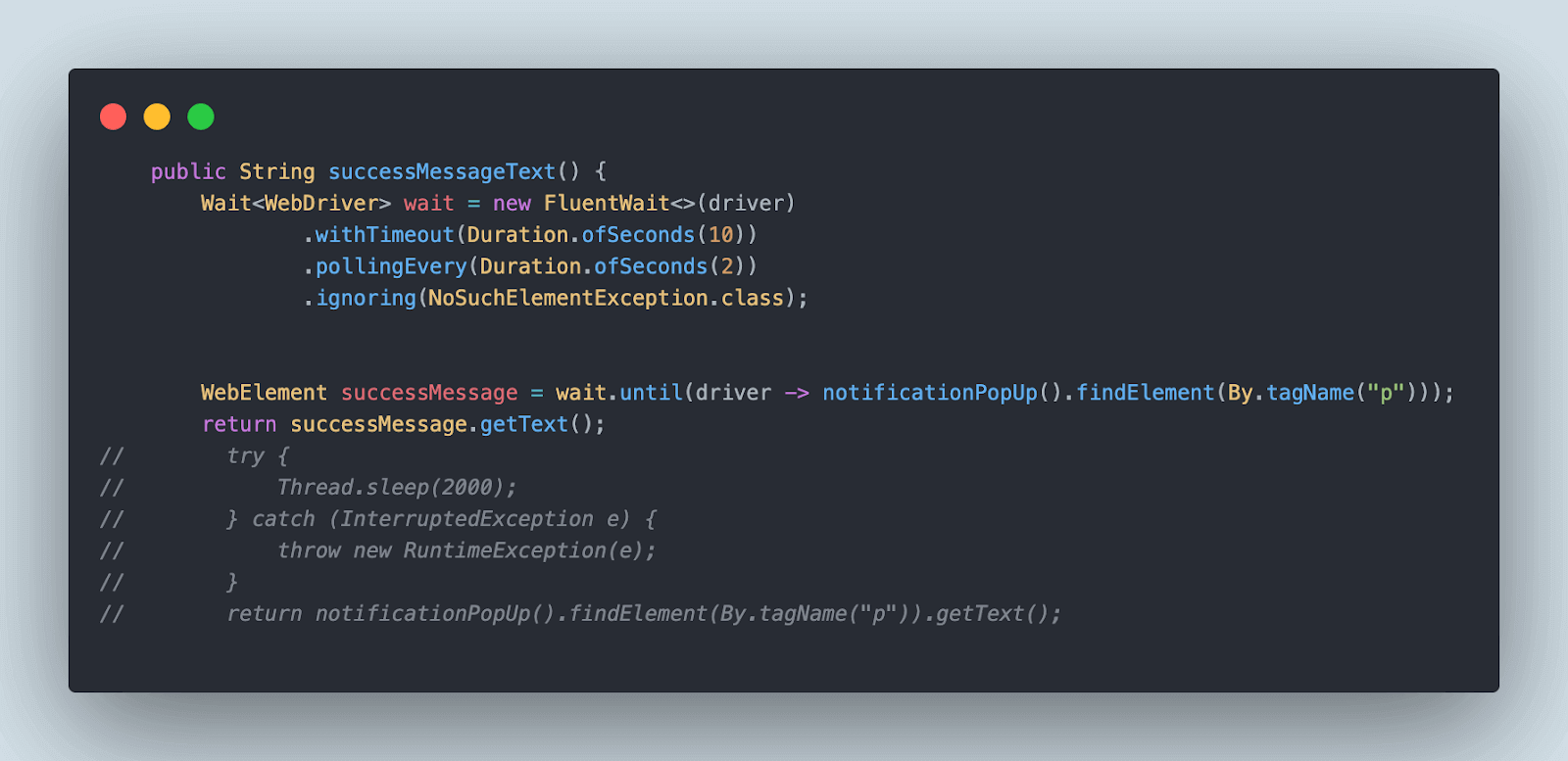
}
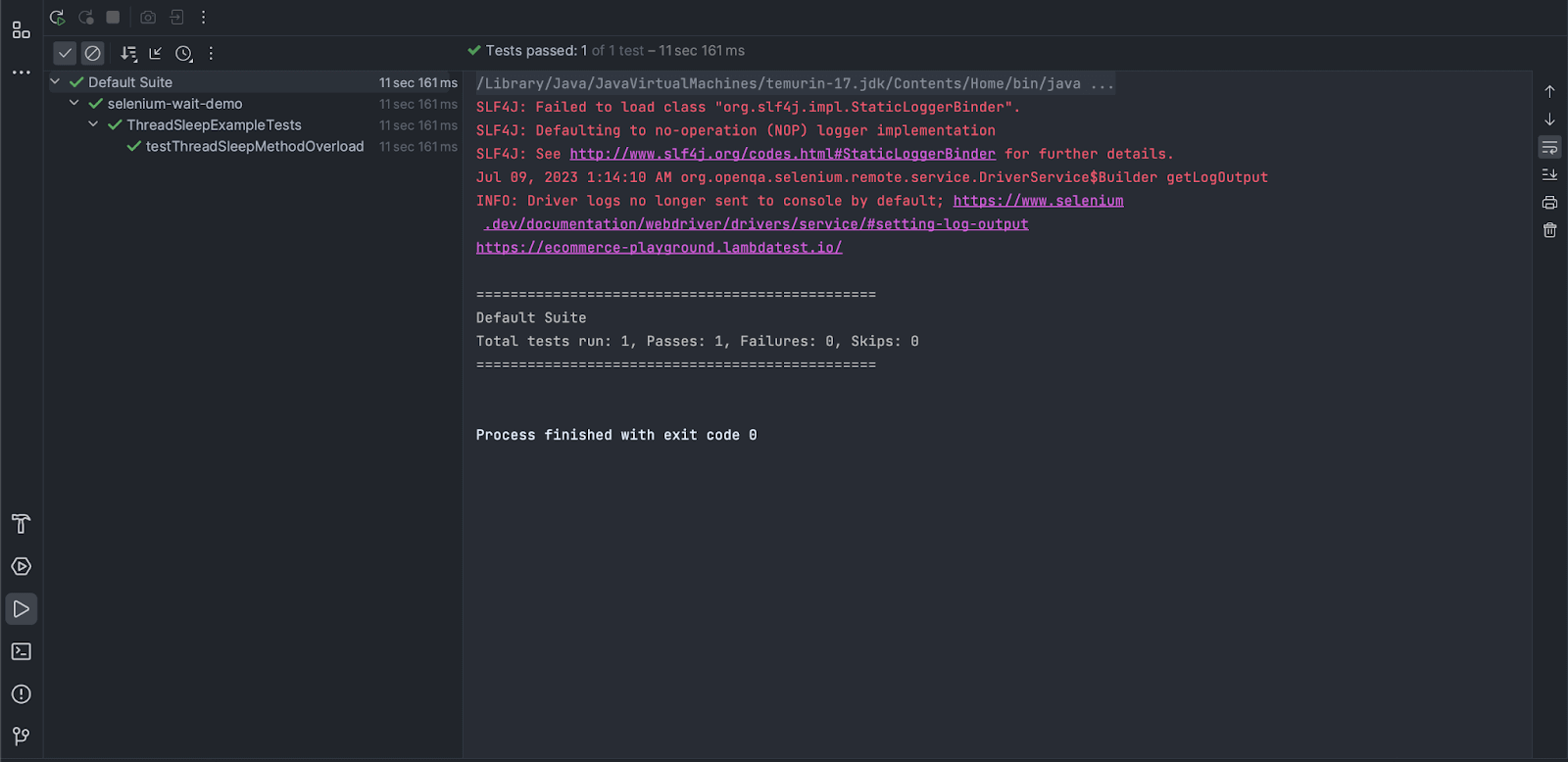
In the above example, we're using Thread.sleep()
to pause the main thread's execution for 60 seconds. The method will throw an InterruptedException
if another thread interrupts it during its sleep.
Now, you might wonder why we'd want to use Thread.sleep()
in the first place. Well, there are several valid reasons:
Thread.sleep()
to delay the execution of a piece of code until some condition is met or another thread has finished processing. Coordinating tasks: By sleeping for a specific amount of time, your thread can coordinate its work with other threads or even UI components that rely on timing. Simulating delays: In certain situations, you might want to simulate a delay or pause in the execution of code, such as when testing asynchronous behavior.
However, it's essential to use Thread.sleep()
judiciously and avoid abusing this method:
Thread.sleep()
in the middle of processing important data or executing time-sensitive operations.
In summary, Thread.sleep()
is a useful method for controlling thread execution and delaying processing. Just remember to use it wisely, considering the context and potential performance implications.
(And don't worry about Grandma's anger – I'm responding solely in English as per your request!)