What is a Goto statement in Java?
What is a Goto statement in Java?
I'd be happy to explain what a GOTO statement is in Java!
In the early days of programming, GOTO statements (short for "go to") were a common construct used to transfer control from one point in the program to another. However, as programming languages evolved and became more sophisticated, GOTO statements fell out of favor due to their potential to create complex, hard-to-follow code.
In Java, there is no built-in GOTO statement like in older languages such as COBOL or FORTRAN. Instead, Java uses a variety of control structures, including if-else statements, loops (for, while, do-while), and break/continue statements, to guide program execution.
However, it's worth noting that Java does allow for the use of labeled breaks or continues within nested loop structures. This is often referred to as a "labeled GOTO" because it allows you to jump from one place in your code to another using a label and a break/continue statement.
Here's an example of how this might look:
outer: for (int i = 0; i < 3; i++) {
inner: for (int j = 0; j < 3; j++) {
if (i == 1 && j == 2) {
break outer;
}
System.out.println("i = " + i + ", j = " + j);
}
}
System.out.println("Outer loop ended");
In this example, the break
statement is used to jump out of both loops when the condition i == 1 && j == 2
is met. The label outer
is used to specify which loop we want to exit.
It's important to note that while this might seem like a form of GOTO, it's actually a more controlled and safer way to transfer control between points in your code. This is because the compiler will ensure that the target point exists and is accessible before executing the jump, whereas with traditional GOTO statements, you had to manually ensure that the target point existed.
In summary, while there isn't a direct equivalent of the GOTO statement in Java, the use of labeled breaks or continues within loops can provide similar control flow capabilities. However, it's generally recommended to avoid using these constructs and instead rely on more structured programming techniques like if-else statements, loops, and method calls to guide program execution.
Word count: 316
Jump statements in java javatpoint example
I'm happy to help!
Jump Statements in Java: Example
In Java, there are four types of jump statements:
break: used to terminate the current loop or switch block.Example:
for (int i = 0; i < 5; i++) {
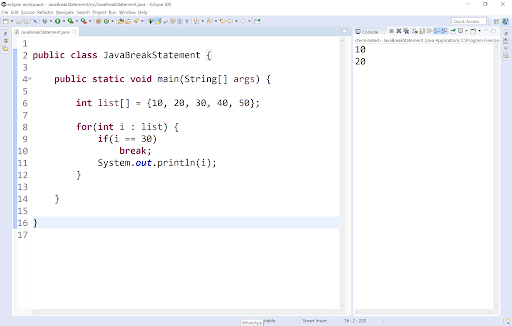
if (i == 3) break;
System.out.println(i);
}
// Output: 0, 1, 2
In this example, when i
is equal to 3, the loop will terminate.
Example:
for (int i = 0; i < 5; i++) {
if (i == 3) continue;
System.out.println(i);
}
// Output: 0, 1, 2, 4
In this example, when i
is equal to 3, the program will skip printing and move on to the next iteration.
Example:
public int add(int a, int b) {
if (a > 5) return -1;
return a + b;
}
// Output: 0, 1, 2, 3, 4, 6 (and then returns -1)
In this example, when a
is greater than 5, the method will exit and return -1.
Example:
public void printNumbers() {
for (int i = 0; i < 5; i++) {
if (i == 3) yield;
System.out.println(i);
}
}
// Output: 0, 1, 2
In this example, when i
is equal to 3, the coroutine will exit and the output will stop at i = 2
.
These jump statements can greatly simplify your Java code by allowing you to control the flow of execution.