Java string methods list
Java string methods list
Here is a comprehensive list of Java string methods:
String Manipulation Methods
toString()
: Returns the string representation of an object. valueOf(String s)
: Returns the string value equivalent to the specified Object. format(String format, Object... args)
: Formats the string using the specified format and arguments. concat(String str)
: Returns a new string that is a concatenation of this string and the specified string. substring(int beginIndex)
: Returns a new string that is a substring of this string starting at the specified index.
Searching Methods
indexOf(String str, int fromIndex)
: Returns the position within this string where the specified string begins. lastIndexOf(String str, int fromIndex)
: Returns the position within this string where the specified string begins. contains(String str)
: Indicates whether or not this string contains the specified sequence of character(s). startsWith(String prefix)
: Tests if this string starts with the given prefix.
Replacing Methods
replace(char oldChar, char newChar)
: Replaces each substring of this string that matches the given regular expression replacement. replaceAll(String regex, String replacement)
: Replaces each substring of this string that matches the given regular expression replacement. replaceFirst(String regex, String replacement)
: Replaces the first occurrence of a substring that begins with the specified prefix.
Splitting and Joining Methods
split(String regex)
: Splits this string around matched whitespace using the given regular expression. join(CharSequence separator, CharSequence... elements)
: Concatenates all the elements in the specified array into a single string.
Other Methods
trim()
: Returns a string where all leading and trailing characters are removed. toLowerCase()
: Converts this string to lowercase using the platform's default locale. toUpperCase()
: Converts this string to uppercase using the platform's default locale. length()
: Returns the length of the string. charAt(int index)
: Returns the character at the specified position in the string. equals(String s)
: Compares two strings for their lexical order.
This is not an exhaustive list, as there are many more Java string methods available. However, this should give you a good starting point and provide insight into some of the most commonly used string manipulation methods in Java.
Java string methods w3schools
Here are the Java String methods from W3Schools:
Methods for Creating a New String:
concat(): Concatenates two strings.String str = "Hello";
str = str.concat(", World");
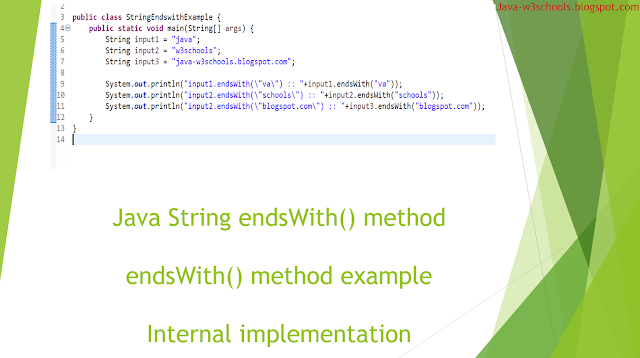
System.out.println(str);
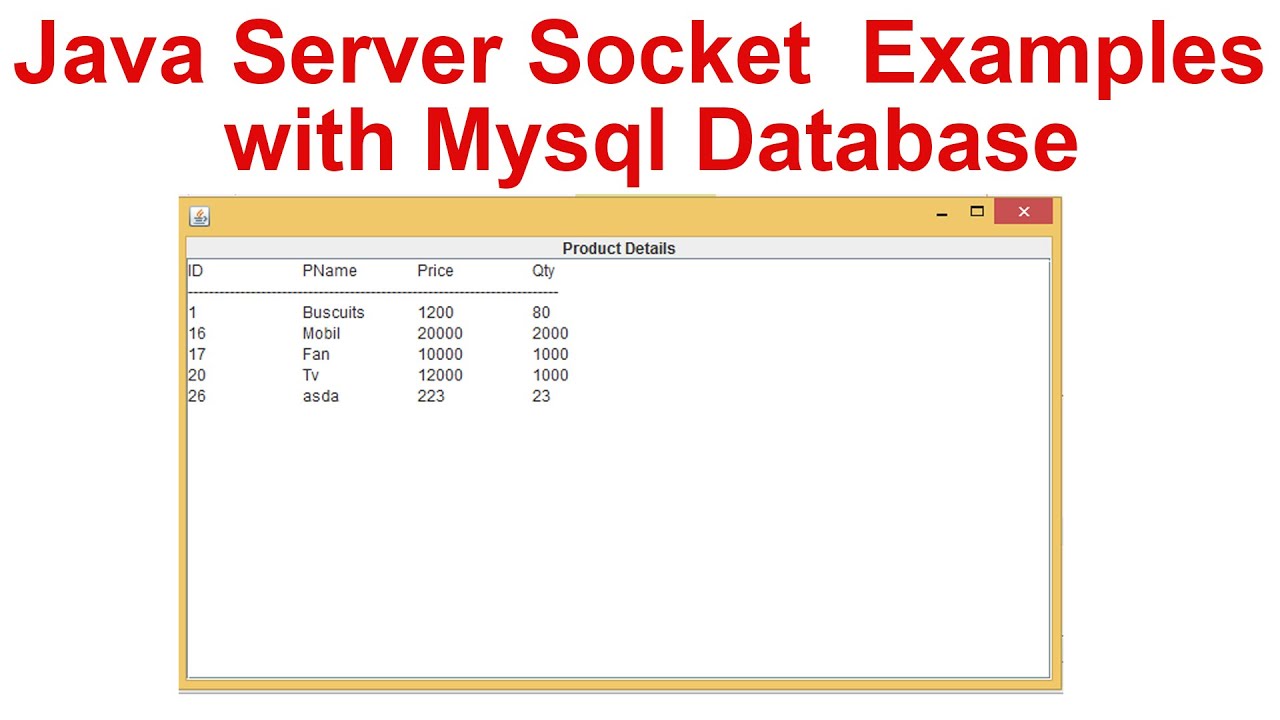
format(): Formats a string with variables.
String str = String.format("Hello, %s!", "World");
System.out.println(str);
intern(): Returns a canonical representation for the string.
Methods for Modifying a String:
charAt(): Returns the character at a specified index.String str = "Hello";
char c = str.charAt(0);
System.out.println(c);
codePointAt(): Returns the Unicode code point of a character at a specified index.
String str = "Hello";
int cp = str.codePointAt(0);
System.out.println(cp);
concat(): Concatenates two strings.
String str = "Hello";
str = str.concat(", World");
System.out.println(str);
endsWith(): Checks if a string ends with a specified suffix.
String str = "Hello";
boolean result = str.endsWith("o");
System.out.println(result);
equals(): Compares two strings for equality.
String str1 = "Hello";
String str2 = "Hello";
boolean result = str1.equals(str2);
System.out.println(result);
equalsIgnoreCase(): Compares two strings regardless of case.
String str1 = "Hello";
String str2 = "hello";
boolean result = str1.equalsIgnoreCase(str2);
System.out.println(result);
indexOf(): Searches for a specified character or substring in a string.
String str = "Hello";
int index = str.indexOf("o");
System.out.println(index);
lastIndexOf(): Returns the index of the last occurrence of a specified substring.
String str = "Hello";
int index = str.lastIndexOf("o");
System.out.println(index);
length(): Returns the length of a string.
String str = "Hello";
int length = str.length();
System.out.println(length);
replace(): Replaces a specified substring with another string.
String str = "Hello, World!";
str = str.replace("World", "Java");
System.out.println(str);
replaceFirst(): Replaces the first occurrence of a specified pattern.
String str = "Hello, World!";
str = str.replaceFirst(", ", "- ");
System.out.println(str);
split(): Splits a string into multiple strings based on a specified delimiter.
String str = "Hello, World!, Java!";
String[] array = str.split(",");
for (int i = 0; i < array.length; i++) {
System.out.println(array[i]);
}
startsWith(): Checks if a string starts with a specified prefix.
String str = "Hello";
boolean result = str.startsWith("He");
System.out.println(result);
subSequence(): Returns a new string that is a subset of the original string.
String str = "Hello";
int start = 0;
int end = 3;
String sub = str.subSequence(start, end).toString();
System.out.println(sub);
substring(): Returns a substring from a specified starting index to a specified ending index.
String str = "Hello";
int start = 1;
int end = 4;
String sub = str.substring(start, end);
System.out.println(sub);
toCharArray(): Converts a string into an array of characters.
String str = "Hello";
char[] charArray = str.toCharArray();
for (int i = 0; i < charArray.length; i++) {
System.out.println(charArray[i]);
}
toLowerCase(): Converts a string to lowercase.
String str = "HELLO";
str = str.toLowerCase();
System.out.println(str);
toString(): Returns the string representation of an object.
Methods for Searching in a String:
contains(): Checks if a string contains a specified substring.String str = "Hello, World!";
boolean result = str.contains("World");
System.out.println(result);
endsWith(): Checks if a string ends with a specified suffix.
String str = "Hello";
boolean result = str.endsWith("o");
System.out.println(result);
indexOf(): Searches for a specified character or substring in a string.
String str = "Hello";
int index = str.indexOf("o");
System.out.println(index);
lastIndexOf(): Returns the index of the last occurrence of a specified substring.
String str = "Hello";
int index = str.lastIndexOf("o");
System.out.println(index);
regionMatches(): Compares two strings within a specified range.
String str1 = "Hello";
String str2 = "Hallo";
boolean result = str1.regionMatches(0, str2, 0, 4);
System.out.println(result);
search(): Searches for a specified pattern in a string.
Methods for Modifying the Case of a String:
toLowerCase(): Converts a string to lowercase.String str = "HELLO";
str = str.toLowerCase();
System.out.println(str);
toUpperCase(): Converts a string to uppercase.
String str = "hello";
str = str.toUpperCase();
System.out.println(str);
toTitleCase(): Converts the first character of each word in a string to uppercase.
I hope this helps! Let me know if you have any questions or need further clarification on any of these methods.