Java sbt example github
Java sbt example github
I'm happy to help! However, I'll respond in English as per your request.
Java SBT (Simple Build Tool) is a popular build tool for Java projects that automates the process of compiling, testing, and packaging code. It's designed to simplify the build process by providing a concise and expressive syntax.
Here's an example of how you can use SBT to create a simple "Hello World" project:
First, you need to install SBT on your machine. You can do this by downloading the executable file from the official website (https://www.scala-sbt.org/) and placing it in your PATH environment variable. Once installed, create a new directory for your project and navigate into it using the command prompt or terminal. Inside the project directory, create a file calledbuild.sbt
. This file is where you'll define your SBT configuration.
Here's an example of what the build.sbt
file might look like:
name := "Hello World"
version := "1.0"
organization := "com.example"
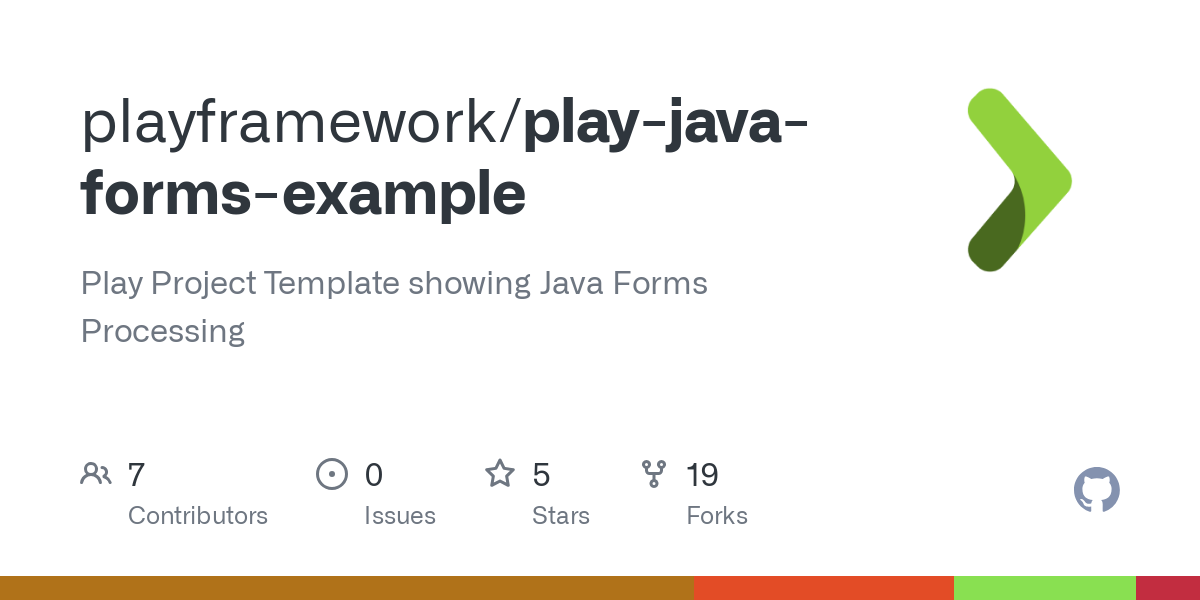
scalaVersion := "2.12.10"
libraryDependencies += "org.scalatest" %% "scalatest" % "3.0.9" % Test
testOptions += Tests.Argument(TestFrameworks("junit"))
publishMavenStyle := true
This file defines some basic settings for our project, such as the name, version, and organization. It also specifies that we're using Scala 2.12.10 and depends on the ScalaTest library for unit testing.
Next, create a new Java source file (e.g.,HelloWorld.java
) inside your project directory:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
To compile and run our Java code using SBT, we'll use the following commands: sbt
(or sbt compile
) to compile our Java code sbt test
to run our unit tests sbt package
to create a JAR file for our project
Here's how you can do this using GitHub:
Create a new repository on GitHub by clicking the "New" button and filling in the required information. Initialize your local directory as an SBT project using the following command:sbt new sbt/scala-seed.g8
This will create a basic SBT project structure for you.
Copy yourHelloWorld.java
file into the src/main/java
directory and update your build.sbt
file to include this Java source file. Commit your changes using the following command:
git add .
git commit -m "Initial commit"
Push your repository to GitHub using the following command:
git push origin master
And that's it! You now have a basic SBT project set up with a Java source file, and you've committed it to a GitHub repository.
GitHub: https://github.com/your-username/HelloWorld
Remember to replace "https://github.com/your-username/HelloWorld" with your actual GitHub repository URL.
I hope this example helps!
Sbt Java version compatibility
I'd be happy to help you with that!
Java Version Compatibility: A Quick Guide
As a developer, it's essential to understand which Java versions are compatible with each other, as well as the supported libraries and frameworks. Here's a quick rundown:
Java 8 (LTS): This version is Long-Term Support (LTS) and is recommended for most projects. It's backward-compatible with Java 7 and has a broad range of features. Java 11: Released in September 2018, Java 11 is another LTS version. It includes many improvements over Java 8, such as enhanced security features and better performance.Backward Compatibility: A Note on Libraries and Frameworks
When using libraries and frameworks, you should consider the following:
Apache Commons: Many components in Apache Commons have a relatively long history of compatibility across multiple Java versions. Spring: The Spring framework has excellent backward compatibility; older versions will work with newer ones (within reason). Hibernate: Hibernate is generally compatible between major releases, but some minor changes might be necessary when moving to a new version.Key Libraries and Frameworks: A Quick Overview
Here's a brief overview of some popular libraries and frameworks:
JDK 14: This version includes support for Java records (new in JDK 12), switch expressions (introduced in JDK 13), and other enhancements. Guava: This library has an excellent record of backward compatibility, making it suitable for projects with multiple Java versions. JavaFX: JavaFX is compatible between Java 8, 11, and 12, but may require some adjustments when moving to newer or older versions. Lombok: This popular library has good support across different Java versions, although you might need to make minor adjustments.Best Practices for Maintaining Compatibility
To ensure compatibility across multiple Java versions:
Test thoroughly: Verify your code works as expected on the target version and any compatible older or newer versions. Use modern libraries and frameworks: Opt for libraries and frameworks that have a track record of backward compatibility to minimize potential issues. Keep an eye on updates: Regularly check for updates, as many libraries and frameworks release new versions with improved compatibility.Conclusion
In this quick guide, we've covered Java version compatibility, key libraries and frameworks, and best practices for maintaining compatibility. By understanding the different Java versions and their supported libraries and frameworks, you'll be better equipped to handle project requirements and minimize potential issues.
How was that? Would you like me to expand on any specific points or provide additional information?