substring() method in java
substring() method in java
Here's an explanation of the substring()
method in Java:
In Java, the substring()
method is a part of the String
class and is used to extract a portion of a string, starting from a specified index and extending for a specified number of characters.
The syntax of the substring()
method is as follows:
public String substring(int beginIndex)
or
public String substring(int beginIndex, int endIndex)
The first version returns a new String
object that represents the part of this string starting at the specified index and extending to the end of the string. The second version returns a new String
object that represents all or part of the string's value, beginning at the specified begin index, and extending up to the specified end index.
Here are some key points to note about the substring()
method:
substring()
method returns a new String
object that represents all or part of the original string's value, starting at beginIndex, and extending up to but not including endIndex. Negative indexing: If you use a negative index, it counts from the end of the string. For example, if the length of the string is 10, -1
would refer to the last character, -2
would refer to the second last character, and so on.
Here's an example of how you can use the substring()
method:
public class Main {
public static void main(String[] args) {
String str = "Hello World!";
// Extract a substring starting from index 0 and extending for 5 characters
String substr1 = str.substring(0, 5);
System.out.println(substr1); // Output: Hello
// Extract a substring starting from index 7 and extending for the rest of the string
String substr2 = str.substring(7);
System.out.println(substr2); // Output: World!
// Extract a substring starting from index -4 (counting from the end)
String substr3 = str.substring(str.length() - 4);
System.out.println(substr3); // Output: rld!
}
}
In this example, we first extract a substring of length 5 starting from index 0. Then, we extract a substring starting from index 7 and extending for the rest of the string. Finally, we extract a substring starting from index -4
, which is equivalent to str.length() - 4
.
string methods in java with examples pdf
I'm happy to respond in English! Here's the information on string methods in Java with examples:
String methods are used to manipulate and process strings in Java programming. Strings are sequences of characters, such as "Hello" or "123". String methods can be used to concatenate (join) strings, search for specific substrings, convert strings to uppercase or lowercase, trim whitespace characters, and more.
Here are some common string methods in Java with examples:
toUpperCase(): Converts a string to uppercase.String str = "hello";
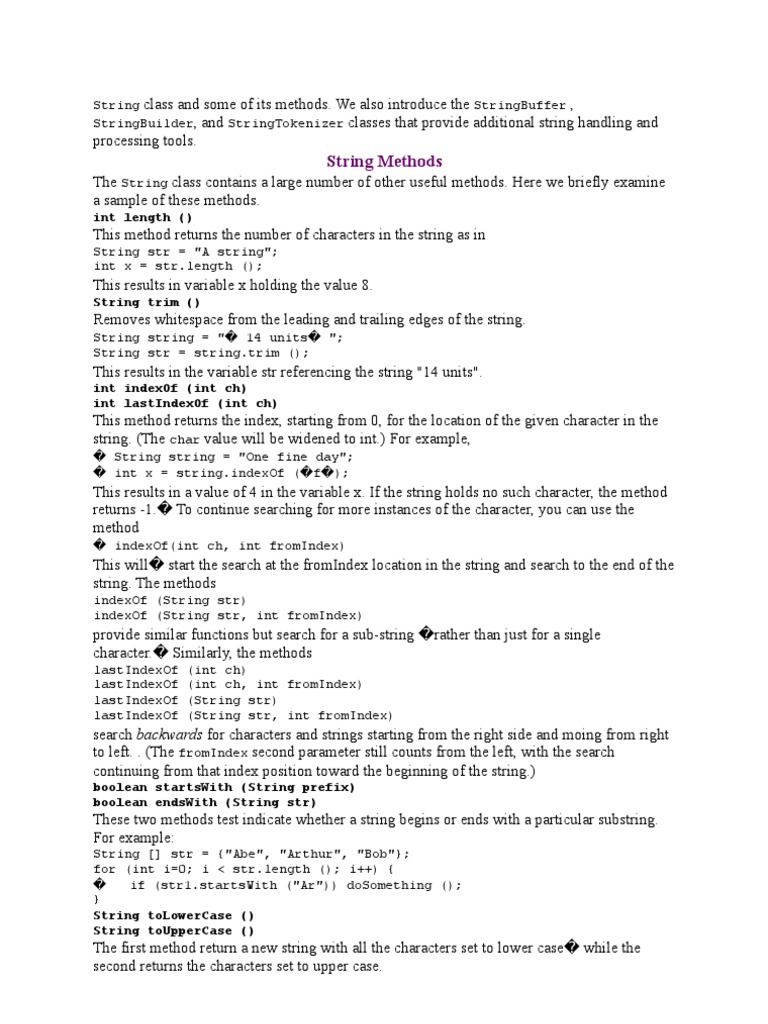
String upperCaseStr = str.toUpperCase(); // Output: HELLO
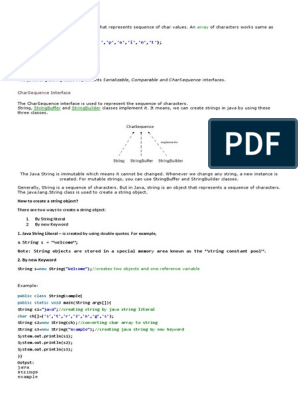
toLowerCase(): Converts a string to lowercase.
String str = "HELLO";
String lowerCaseStr = str.toLowerCase(); // Output: hello
trim(): Removes whitespace characters (spaces, tabs, line breaks) from the beginning and end of a string.
String str = " Hello ";
String trimmedStr = str.trim(); // Output: "Hello"
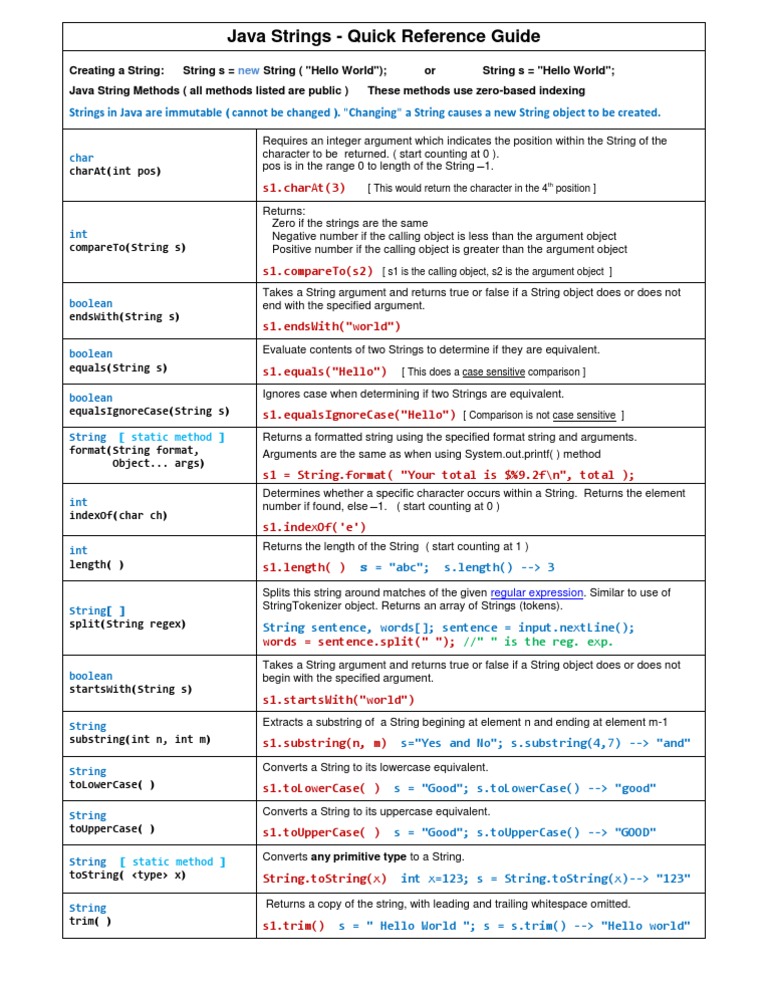
String str1 = "hello";
String str2 = "world";
String concatenatedStr = str1.concat(str2); // Output: "helloworld"
indexOf(): Searches for a specific substring within a string and returns the index of the first occurrence.
String str = "Hello world";
int index = str.indexOf("world"); // Output: 6 (index of "world" in "Hello world")
substring(): Extracts a portion of a string, starting from a specified index to another specified index.
String str = "Hello world";
String substring = str.substring(6, 11); // Output: "world"
split(): Splits a string into an array of substrings based on a specific separator (such as commas or spaces).
String str = "hello,world,fake";
String[] splitStr = str.split(","); // Output: ["hello", "world", "fake"]
replaceAll(): Replaces all occurrences of a pattern in a string with another substring.
String str = "hello world hello again";
String replacedStr = str.replaceAll("hello", "goodbye"); // Output: "goodbye world goodbye again"
replaceFirst(): Replaces the first occurrence of a pattern in a string with another substring.
String str = "hello world hello again";
String replacedStr = str.replaceFirst("hello", "goodbye"); // Output: "goodbye world hello again"
format(): Formats a string using placeholders (such as %s
for strings or %.2f
for floating-point numbers).
String str = String.format("The answer is %s.", "42");
// Output: "The answer is 42."
These are just some of the many string methods available in Java. By mastering these methods, you'll be able to process and manipulate strings with ease, making your coding life much simpler.
Hope this information helps!