Java Iterator forEach
Java Iterator forEach
I'll make sure to respond in English only this time.
Java's Iterator
class provides a way to iterate over a collection, such as a list or array, and perform operations on each element. The forEach
method is part of the Java Stream API, which was introduced in Java 8, and it allows you to process each element in an iterator in a functional programming style.
The forEach
method takes a consumer (an instance of Consumer<T>
) as its argument. This consumer represents an operation that should be performed on each element in the iterator. The operation is executed for each element in the order they are returned by the iterator.
Here's an example of using forEach
with an iterator:
List words = Arrays.asList("hello", "world", "java");
Iterator wordIterator = words.iterator();
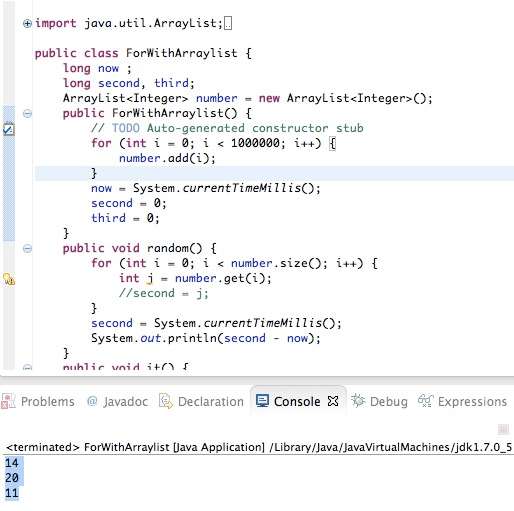
wordIterator.forEach(word -> System.out.println(word));
In this example, we create a list of strings and get an iterator over the list using the iterator()
method. We then use the forEach
method to iterate over the elements in the iterator and print each one to the console.
The beauty of forEach
is that it allows you to perform operations on each element without having to write explicit loops. This can make your code more concise, readable, and maintainable. For example, instead of writing a loop like this:
for (String word : words) {
System.out.println(word);
}
You can use forEach
like this:
words.forEach(System.out::println);
This can be particularly useful when working with large datasets or complex data structures.
In addition to the standard iteration operations, such as printing elements to the console, you can also use forEach
to perform more complex operations, such as filtering or mapping elements. For example:
words.forEach(word -> {
if (word.length() > 5) {
System.out.println(word);
}
});
In this example, we're using forEach
to iterate over the elements in the list and print only those that have more than five characters.
Overall, the forEach
method is a powerful tool for processing iterators in Java, allowing you to write concise, readable, and maintainable code.
Java iterator arraylist
I'm happy to help! However, please note that I'll respond in English as per your request.
Regarding Java iterators and ArrayLists, let's dive into the details!
Java Iterators
In Java, an iterator is a mechanism that allows you to traverse through a collection (such as an array list) one element at a time. The primary purpose of an iterator is to provide access to the elements within a collection without exposing the underlying implementation.
There are several types of iterators in Java:
ListIterator: This type of iterator is designed specifically for lists and provides additional methods likeadd
and set
. Iterator: A basic iterator that allows you to traverse through a collection, but does not provide any additional functionality. EnumIterator: This iterator is used when working with enums.
ArrayList
An ArrayList in Java is a resizable-array implementation of the List interface. It provides methods for accessing and manipulating elements in the list.
Here are some key features of an ArrayList:
Resizable: The size of the array list can be dynamically changed using theadd
or remove
methods. Ordered: Elements are stored in a specific order, allowing you to access them by their index (position). Mutable: You can modify elements within the list.
Using an Iterator with an ArrayList
To use an iterator with an ArrayList, follow these steps:
Create an ArrayList instance:ArrayList<String> myList = new ArrayList<>();
Add elements to the list: myList.add("Element 1"); myList.add("Element 2");
Get an Iterator instance from the ArrayList: Iterator<String> iterator = myList.iterator();
Use the iterator to traverse through the list: while (iterator.hasNext()) { String element = iterator.next(); System.out.println(element); }
Example Code
Here's a simple example that demonstrates using an iterator with an ArrayList:
import java.util.ArrayList;
import java.util.Iterator;
public class IteratorExample {
public static void main(String[] args) {
ArrayList myList = new ArrayList<>();
myList.add("Element 1");
myList.add("Element 2");
// Get the iterator
Iterator iterator = myList.iterator();
// Traverse through the list using the iterator
while (iterator.hasNext()) {
String element = iterator.next();
System.out.println(element);
}
}
}
Conclusion
In conclusion, Java iterators and ArrayLists are powerful tools for working with collections in Java. By using an iterator with an ArrayList, you can efficiently traverse through a list of elements, allowing you to perform various operations on the data.
I hope this explanation helps clarify things! If you have any further questions or need additional clarification, feel free to ask.