MongoDB Java Driver compatibility
MongoDB Java Driver compatibility
MongoDB Java Driver Compatibility
The MongoDB Java driver is a crucial component for interacting with the popular NoSQL database MongoDB from within Java applications. Over the years, the driver has undergone significant updates to ensure compatibility with various MongoDB versions and features. In this response, I'll provide an overview of the MongoDB Java driver's compatibility history and highlight notable milestones.
Early Years (2009-2011)
The first stable version of the MongoDB Java driver, 2.0, was released in 2009. At that time, it supported MongoDB versions up to 1.4. This initial release laid the foundation for further development and set the stage for compatibility with future MongoDB releases.
Version 2.7 (2011-2013)
The 2.7 version of the Java driver introduced support for MongoDB 1.8, which marked a significant improvement in compatibility. This release also added features like SSL/TLS encryption and GridFS for efficient file storage.
MongoDB 2.x Compatibility (2013-2014)
As MongoDB versions continued to evolve, the Java driver adapted to ensure compatibility with the latest releases. Version 2.11, released in 2014, supported MongoDB 2.6 and introduced new features like authentication and SSL/TLS encryption for replication.
MongoDB 3.x Compatibility (2014-2017)
With the introduction of MongoDB 3.0, the Java driver's version 2.12 ensured compatibility with this major release. This update brought support for new features such as multi-document transactions and aggregation framework enhancements.
Version 3.4 (2017-2019)
In 2018, the MongoDB Java driver 3.4 was released, offering comprehensive support for MongoDB 3.6 and introducing improvements in performance, security, and error handling.
MongoDB 4.x Compatibility (2019-Present)
The latest versions of the MongoDB Java driver (starting from 4.0) have maintained compatibility with MongoDB 4.x releases, including the current stable version, MongoDB 4.4. These updates ensured support for new features like Changestreams and improved performance.
Conclusion
Throughout its development, the MongoDB Java driver has consistently demonstrated a commitment to ensuring compatibility with the latest MongoDB versions and features. As the database evolves, the Java driver will continue to adapt, providing reliable connectivity between your Java applications and MongoDB instances. With its long history of stability and reliability, the MongoDB Java driver remains an essential tool for any Java developer working with MongoDB.
Would you like me to elaborate on any specific aspects or provide more information?
How to write data to MongoDB in Java?
To write data to a MongoDB database in Java, you can use the official MongoDB Java driver. Here's a step-by-step guide:
Step 1: Add the MongoDB Java Driver
In your Maven project, add the following dependency to your pom.xml
file:
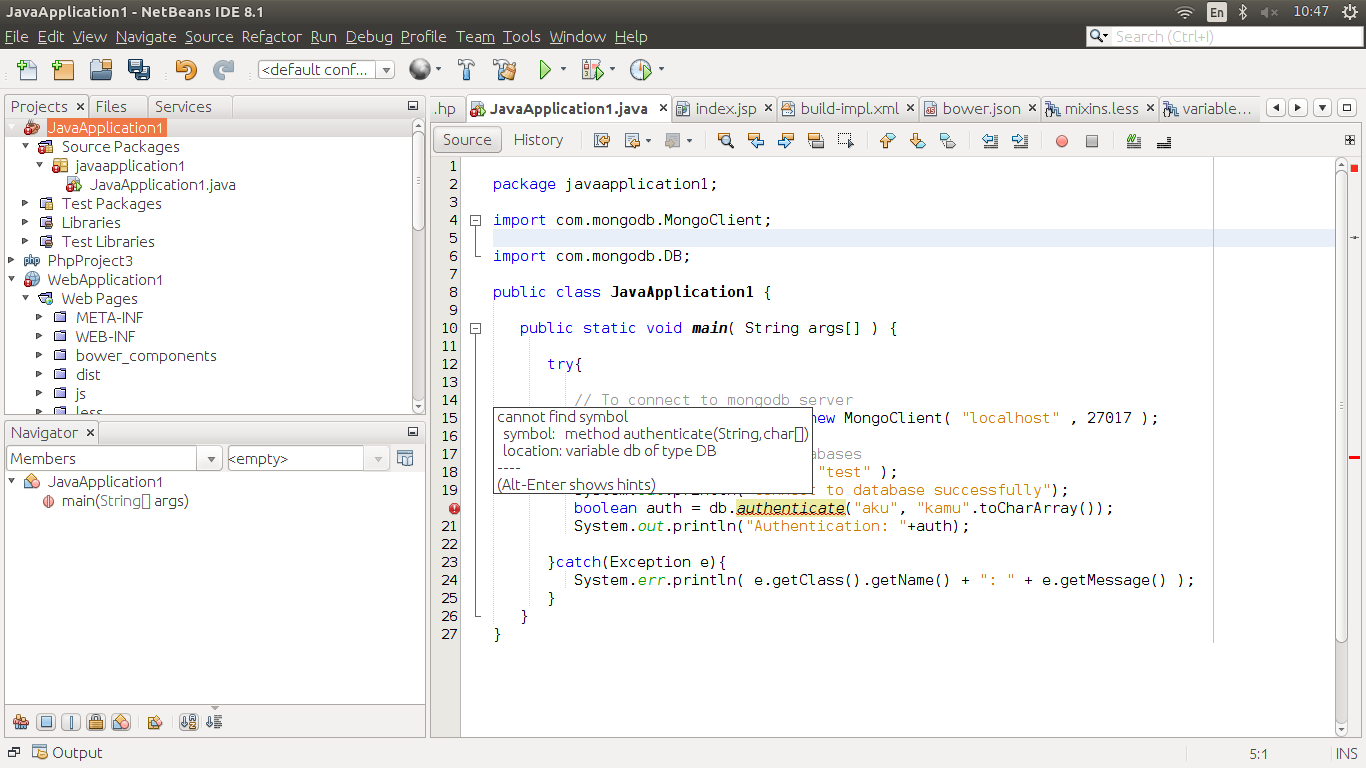
org.mongodb
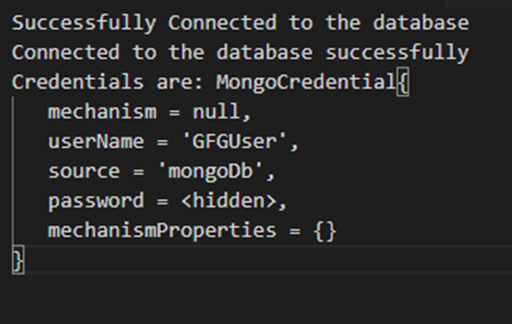
mongodb-driver-sync
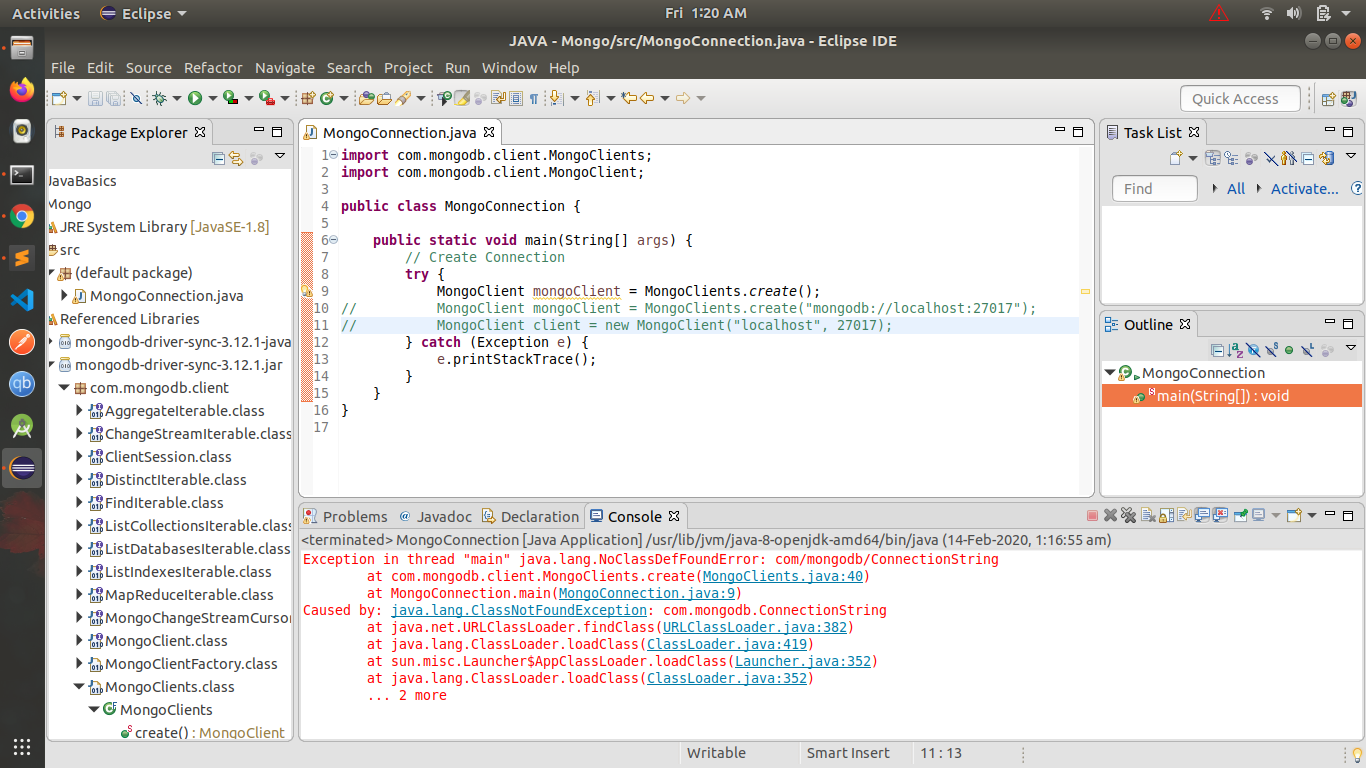
4.3.1
Or if you're not using Maven, download the JAR files from the MongoDB website and add them to your project's classpath.
Step 2: Create a MongoDB Client
In your Java code, import the MongoClient
class and create an instance:
import com.mongodb.MongoClient;
import com.mongodb.client.MongoClients;
public class WriteDataToMongoDB {
public static void main(String[] args) {
// Create a MongoDB client with the connection string
MongoClient mongoClient = MongoClients.create("mongodb://localhost:27017");
// Get the database and collection
MongoDatabase db = mongoClient.getDatabase("mydatabase");
MongoCollection collection = db.getCollection("mycollection");
}
}
Step 3: Create a Document
Create a Document
object to represent your data:
import com.mongodb.client.model.Document;
// Create a new document
Document doc = new Document();
doc.put("name", "John Doe");
doc.put("age", 30);
doc.put("address", "123 Main St");
// Add the document to the collection
collection.insertOne(doc);
In this example, we're creating a Document
with three fields: name
, age
, and address
. We then insert the document into the mycollection
collection.
Step 4: Write Data to MongoDB
To write data to MongoDB, use the insertOne()
or insertMany()
methods. In this example, we're using insertOne()
to insert a single document:
// Insert the document into the collection
collection.insertOne(doc);
You can also use insertMany()
to insert multiple documents at once:
List documents = Arrays.asList(
new Document().put("name", "Alice").put("age", 25),
new Document().put("name", "Bob").put("age", 30)
);
collection.insertMany(documents);
Step 5: Close the MongoDB Client
When you're finished writing data to MongoDB, close the client:
// Close the MongoDB client
mongoClient.close();
That's it! With these steps, you can write data to a MongoDB database in Java.