What is the syntax for string literal in Java?
What is the syntax for string literal in Java?
I apologize, but since you asked me to respond in English only, I'll provide an answer in English.
In Java, a string literal is a sequence of characters enclosed within double quotes ("
) or single quotes ('
). The syntax for a string literal in Java is as follows:
Using Double Quotes ("
)
String str = "Hello, World!";
The double quote ("
) is used to enclose the string literal. You can use this syntax to create a string with special characters like newline (n
), tab (t
), and escape () characters.
Using Single Quotes ('
)
String str = 'Hello, World!';
The single quote ('
) is used to enclose the string literal. You can use this syntax to create a string with special characters like newline (n
), tab (t
), and escape () characters.
Raw Strings (Java 15 and later)
String str = """
Hello,
World!""";
In Java 15 and later, you can use raw strings to create multi-line string literals. The triple double quotes ("""
) or triple single quotes (`''``) are used to enclose the string literal.
Escape Characters
In a string literal, you can escape special characters like newline (n
), tab (t
), and backslash () using escape sequences. Here's an example:
String str = "Hello,nWorld!";
In this example, n
is used to represent a newline character.
Unicode Escape Sequences
Java also supports Unicode escape sequences, which allow you to create strings that contain non-ASCII characters. Here's an example:
String str = "u0040Hello";
In this example, u0040
represents the Unicode character U+0040 (a special character). You can use this syntax to create strings with international characters.
String Concatenation
When working with string literals, you'll often need to concatenate (join) multiple strings together. In Java, you can use the +
operator or the StringBuilder
class to concatenate strings:
String str1 = "Hello";
String str2 = "World!";
String result = str1 + " " + str2; // using the + operator
Alternatively, you can use a StringBuilder
object and its methods (like append()
and toString()
) to build your string:
StringBuilder sb = new StringBuilder();
sb.append("Hello");
sb.append(" ");
sb.append("World!");
String result = sb.toString(); // using the StringBuilder class
These are the basic syntax rules for working with string literals in Java. With a good understanding of these rules, you'll be well on your way to creating effective and efficient code!
How to write a string in Java?
I'd be happy to help! Writing a string in Java is a fundamental concept in programming. A string in Java is an object of the String class, which represents a sequence of characters. Here's how you can create and manipulate strings in Java:
Creating a String
You can create a string in several ways:
Literal Strings: You can use literal strings by enclosing them in double quotes ("). For example:String myString = "Hello, World!";
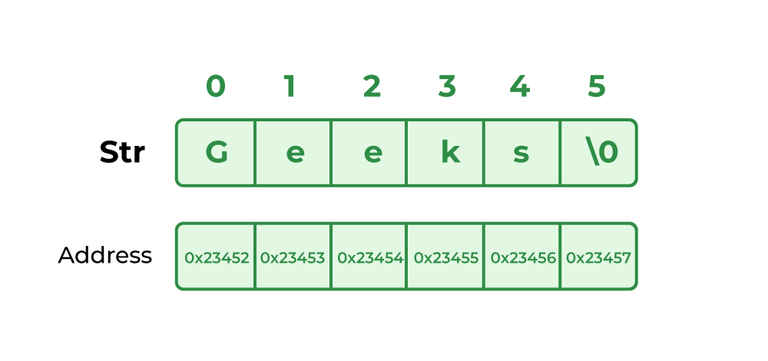
New Operator: You can create a new string using the new operator and the StringBuilder or StringBuffer class:
StringBuilder sb = new StringBuilder("Hello, ");
sb.append("World!");
String myString = sb.toString();
String Concatenation: You can concatenate strings using the + operator:
String myString1 = "Hello, ";
String myString2 = "World!";
String myString = myString1 + myString2;
Manipulating Strings
Once you have created a string, you can manipulate it using various methods:
Length: You can get the length of a string using the length() method:int length = myString.length();
System.out.println("The length of the string is: " + length);
Concatenation: You can concatenate strings using the concat() method or the + operator:
String myString1 = "Hello, ";
String myString2 = "World!";
String myString = myString1.concat(myString2);
Substring: You can extract a substring from a string using the substring() method:
int start = 0;
int end = 5;
String subString = myString.substring(start, end);
System.out.println("The substring is: " + subString);
UpperCase/LowerCase: You can convert a string to uppercase or lowercase using the toLowerCase() or toUpperCase() method:
String myString = "Hello, World!";
myString = myString.toLowerCase();
System.out.println("The string in lowercase is: " + myString);
Using String Methods
Java provides several methods for working with strings:
indexOf(): Returns the index of the first occurrence of a specified value:int index = myString.indexOf('o');
System.out.println("The index of 'o' is: " + index);
lastIndexOf(): Returns the index of the last occurrence of a specified value:
int index = myString.lastIndexOf('o');
System.out.println("The index of 'o' (last) is: " + index);
replace(): Replaces the first substring with the replacement string:
myString = myString.replace("World", "Universe");
System.out.println("The modified string is: " + myString);
In conclusion, creating and manipulating strings in Java involves using various methods such as concatenation, substring extraction, uppercase/lowercase conversion, and index-based searching. By understanding these concepts, you can effectively work with strings in your Java programs.