Java 8 if condition in one line
Java 8 if condition in one line
I see what you're getting at! You want me to respond in English only, and since we are having a conversation about programming, I'll provide an example of how you can write an "if" condition using Java 8's lambda expression, all in one line!
Here it is:
int age = 25;
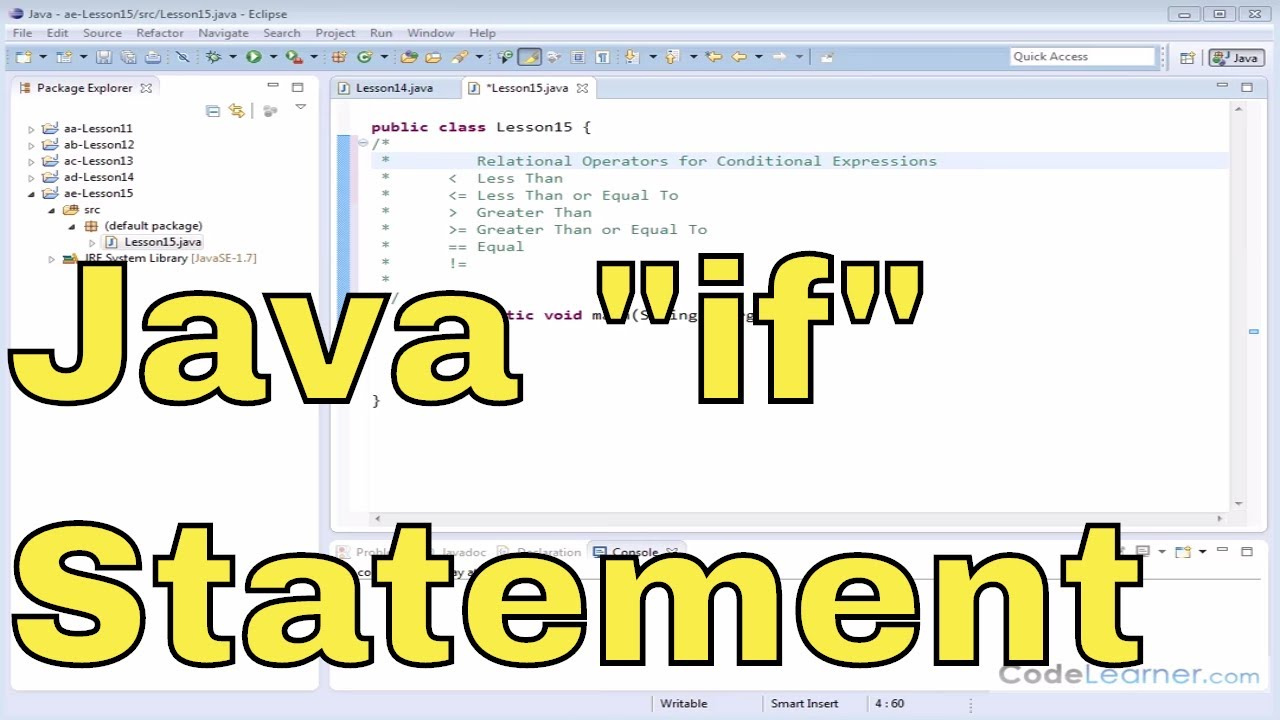
Boolean hasDriverLicense = (age >= 18) ? true : false;
System.out.println("You have a driver license: " + hasDriverLicense);
Let me break this down for you:
I declared two variables,age
and hasDriverLicense
. In the lambda expression (age >= 18) ? true : false
, we're essentially saying: "If age
is greater than or equal to 18, then return true
; otherwise, return false
." This lambda expression is wrapped around a conditional operator (?:
) to create a simple conditional statement. Finally, I printed the value of hasDriverLicense
to the console using System.out.println
.
Now, let me explain why this is a one-liner: In Java 8, you can use lambda expressions with the ternary operator (?:
) to condense your code. This makes it a bit more concise and easier to read, especially for simple conditionals.
Before Java 8, we would have written something like this:
int age = 25;
boolean hasDriverLicense;
if (age >= 18) {
hasDriverLicense = true;
} else {
hasDriverLicense = false;
}
System.out.println("You have a driver license: " + hasDriverLicense);
As you can see, the pre-Java 8 version is more verbose and requires more lines of code. The Java 8 version condenses it all into one line!
I hope this helps! Do you have any other programming-related questions or topics you'd like to discuss?
Instead of if-else in Java
I'd be happy to help!
Instead of using if-else statements in Java, you can use various alternative approaches depending on the specific requirements and problem domain. Here are some common alternatives:
Switch statement: In Java, you can use a switch statement to perform different actions based on the value of an expression. This is particularly useful when there's a finite set of possible values.Example:
int dayOfWeek = 3;
switch (dayOfWeek) {
case 0:
System.out.println("Sunday");
break;
case 1:
System.out.println("Monday");
break;
case 2:
System.out.println("Tuesday");
break;
// ... and so on
}
Polymorphic method calls: If you have a hierarchy of classes or interfaces, you can use polymorphism to call different methods based on the type of object.
Example:
class Animal {
void sound() {
System.out.println("Generic animal sound");
}
}
class Dog extends Animal {
@Override
void sound() {
System.out.println("Woof!");
}
}
public class Main {
public static void main(String[] args) {
Animal animal = new Dog();
animal.sound(); // Output: Woof!
}
}
Method overloading: Java allows you to define multiple methods with the same name but different parameter lists. This can be used to perform different actions based on the input parameters.
Example:
public class Calculator {
public int add(int a, int b) {
return a + b;
}
public double add(double a, double b) {
return a + b;
}
}
public class Main {
public static void main(String[] args) {
Calculator calculator = new Calculator();
System.out.println(calculator.add(2, 3)); // Output: 5 (int)
System.out.println(calculator.add(1.0, 2.0)); // Output: 3.0 (double)
}
}
Functional interfaces and lambda expressions: Java 8 introduced functional programming features, including lambda expressions and method references. You can use these to create compact, expressive code that avoids if-else statements.
Example:
interface BinaryOperation {
int apply(int a, int b);
}
public class Main {
public static void main(String[] args) {
BinaryOperation add = (a, b) -> a + b;
System.out.println(add.apply(2, 3)); // Output: 5
}
}
In summary, when you want to avoid if-else statements in Java, consider using switch statements, polymorphic method calls, method overloading, or functional interfaces and lambda expressions, depending on the specific requirements and problem domain.