Class data type in Java
Class data type in Java
I'll respond in English for this one.
In Java, a class is the blueprint for creating objects that contain both data and methods (functions) that operate on that data. A class defines the properties and behavior of an object, which is an instance of the class.
Here are some key aspects of classes in Java:
Class Definition: To define a class, you use theclass
keyword followed by the name of the class. For example: public class Dog {}
Properties (also known as Fields): A class can have properties that represent the data it holds. Properties are defined inside the class definition using the private
access modifier. For example: private String name; private int age;
Methods: A class can have methods, which are functions that perform actions on an object's properties. Methods are also defined inside the class definition and are used to operate on the object's data. For example: public void bark() { System.out.println("Woof!"); }
Constructors: When you create a new object from a class, Java calls the constructor method to initialize the object's properties. A constructor is a special type of method that has the same name as the class. For example: public Dog(String name, int age) { this.name = name; this.age = age; }
Access Modifiers: In Java, you can use access modifiers (such as public
, private
, and protected
) to control which parts of a class are accessible from outside the class.
Here's an example of a simple Dog
class in Java:
public class Dog {
private String name;
private int age;
public Dog(String name, int age) {
this.name = name;
this.age = age;
}
public void bark() {
System.out.println("Woof!");
}
public void printInfo() {
System.out.println("Name: " + name);
System.out.println("Age: " + age);
}
}
In this example, the Dog
class has two properties (name and age), one constructor method, and three methods (bark(), printInfo(), and a default no-arg constructor).
When you create a new Dog
object using the following code:
Dog myDog = new Dog("Fido", 3);
Java will call the constructor to initialize the object's properties, and then you can use the methods on the object:
myDog.bark(); // Output: Woof!
myDog.printInfo(); // Output: Name: Fido, Age: 3
That's a basic overview of classes in Java!
What is the difference between variable and datatype in Java?
I'm happy to respond in English for you.
In Java, variable
and datatype
are two fundamental concepts that are often confused with each other. While they are related, they serve distinct purposes.
Variable: A variable is a named storage location that holds a value of a specific type. In Java, a variable can hold a primitive value (such as an integer, boolean, or character) or an object reference. Think of a variable as a container that can hold different values at different times. For example:
int x = 5; // declare and initialize an integer variable x to 5
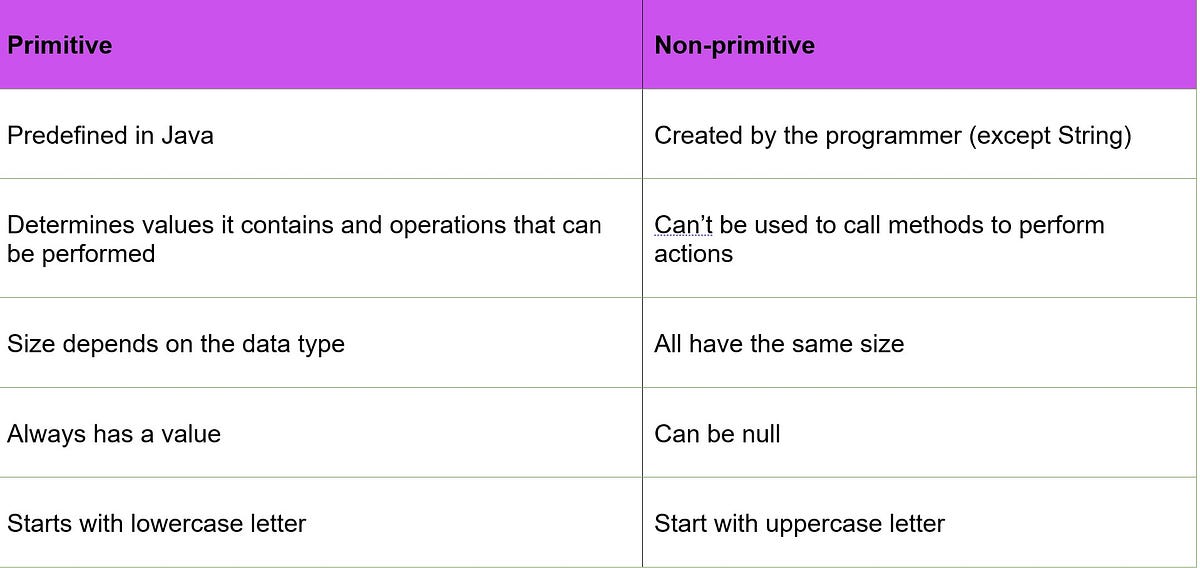
x = 10; // later, assign the value 10 to the same variable x
Datatype: A datatype (or data type) is a category of values that can be stored in a variable. In Java, datatypes are predefined by the language and determine the type of value a variable can hold. Datatypes include:
Primitive types:int
, byte
, short
, long
, float
, double
, boolean
, char
Reference types: String
, Array
, Object
(which encompasses classes, interfaces, and enums)
Datatypes determine the type of value that can be assigned to a variable. For example:
int x = 5; // integer datatype
String s = "Hello"; // String datatype
The key differences between variables and datatypes are:
Variable is the named storage location, whiledatatype
is the category of values it can hold. A variable can be reassigned to hold a value of different types, whereas a datatype determines the type of value that can be assigned to a variable in the first place. In Java, you declare and initialize a variable with a specific datatype (e.g., int x = 5;
). This declaration specifies both the variable name (x
) and its datatype (int
).
In summary:
A variable is a named storage location that can hold different values of a specific datatype. Datatype is the category of values that a variable can hold, determining what type of value can be assigned to it.Understanding this distinction is crucial for writing effective and efficient Java code.