What is a double type in java with example
What is a double type in java with example
I'll be happy to help you!
In Java, a double
is a primitive data type that represents a 64-bit floating-point value. It has a minimum value of approximately 4.9 × 10^-324 and a maximum value of approximately 1.8 × 10^308.
Here's an example:
public class Main {
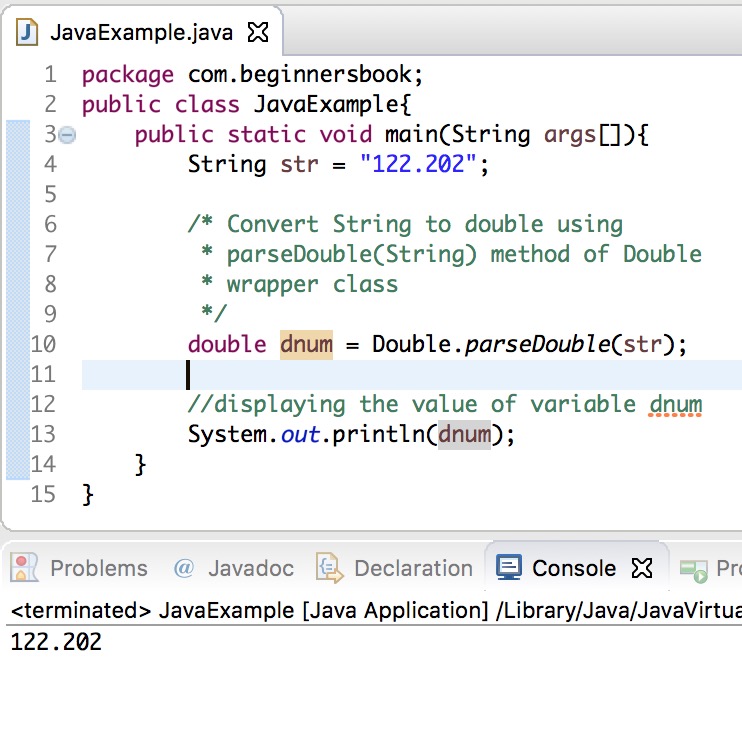
public static void main(String[] args) {
// Declare and initialize a double variable
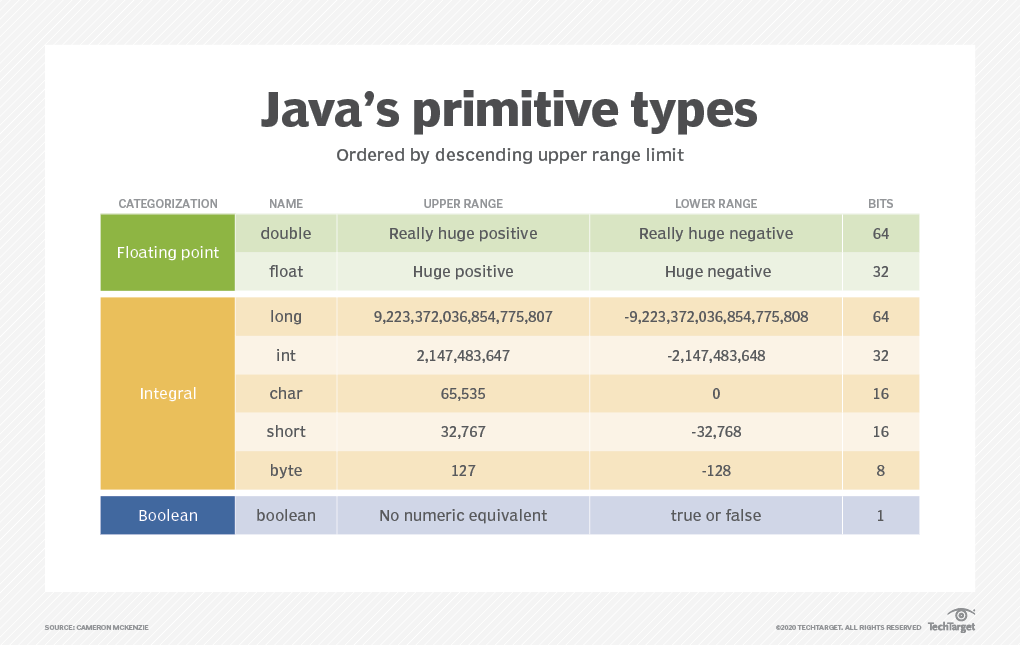
double myDouble = 3.14;
System.out.println("The value of myDouble is: " + myDouble);
// Perform some mathematical operations with the double variable
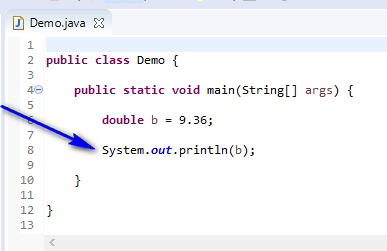
double result1 = myDouble * 2;
double result2 = Math.sqrt(myDouble); // Calculate the square root
System.out.println("Result 1: " + result1);
System.out.println("Result 2: " + result2);
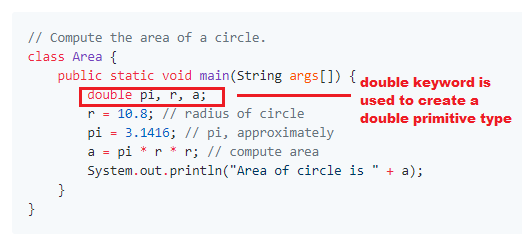
}
}
When you run this program, it will print:
The value of myDouble is: 3.14
Result 1: 6.28
Result 2: 1.772295
As you can see, double
variables are useful for storing and manipulating decimal values in Java. They can be used to represent a wide range of numerical values, from very small fractions to very large numbers.
Some important notes about double
variables:
double
values, it's generally a good idea to use the double
type consistently throughout your program to avoid unexpected results. Be aware that due to the way floating-point numbers are represented in memory, you might encounter small rounding errors when working with double
values. For example:
double a = 0.1;
double b = 0.2;
if (a + b == 0.3) {
System.out.println("True");
} else {
System.out.println("False");
}
In this case, the output will be False
, because the sum of a
and b
is actually very close to, but not exactly equal to, 0.3.
Overall, double
variables are a powerful tool in Java for working with decimal values, but it's essential to understand their limitations and quirks when performing mathematical operations.
Hope that helps!
Float in Java
I'll respond in English and provide an example of floating in Java.
Floating is a fundamental concept in programming languages like Java. It's used to represent decimal numbers or real numbers with fractional parts. In Java, you can use the float
or double
data types to declare variables that hold floating-point values.
Here's an example of how you can create and manipulate floating-point values in Java:
public class FloatingExample {
public static void main(String[] args) {
// Declare a float variable
float myFloat = 3.14f;
// Print the value of myFloat
System.out.println("myFloat: " + myFloat);
// Declare a double variable
double myDouble = 2.71d;
// Print the value of myDouble
System.out.println("myDouble: " + myDouble);
// Perform arithmetic operations with floating-point numbers
float sum = myFloat + myDouble;
System.out.println("Sum: " + sum);
// Check if a float is equal to another using the == operator
boolean isEqual = myFloat == 3.14f;
System.out.println("Is myFloat equal to 3.14? " + isEqual);
}
}
In this example, we declare two floating-point variables myFloat
and myDouble
, both with initial values. We then print their values using the System.out.println()
method.
Next, we demonstrate how to perform basic arithmetic operations like addition on floating-point numbers. In Java, you can combine a float
and a double
without explicit type casting.
Lastly, we show that you can use the ==
operator to check if two floating-point values are equal. Note that this might not work as expected due to how floating-point numbers are represented internally (using binary fractions). For precise comparisons, consider using methods like equals()
or compareTo()
instead of the ==
operator.
When working with floating-point numbers in Java, keep in mind:
Precision: Floating-point calculations can result in slight imprecision, especially when performing complex operations. Type conversions: Be aware that Java performs implicit type conversions betweenfloat
and double
values, which might affect the precision of your results.
By understanding these concepts and best practices for working with floating-point numbers, you'll be well on your way to creating accurate and efficient programs in Java!