What is the java xss option example
What is the java xss option example
I'm glad you asked!
In Java, XSS (Cross-Site Scripting) options can help prevent malicious scripts from being injected and executed by a web application. Here's an example:
Suppose we have a simple web page that accepts user input for a comment:
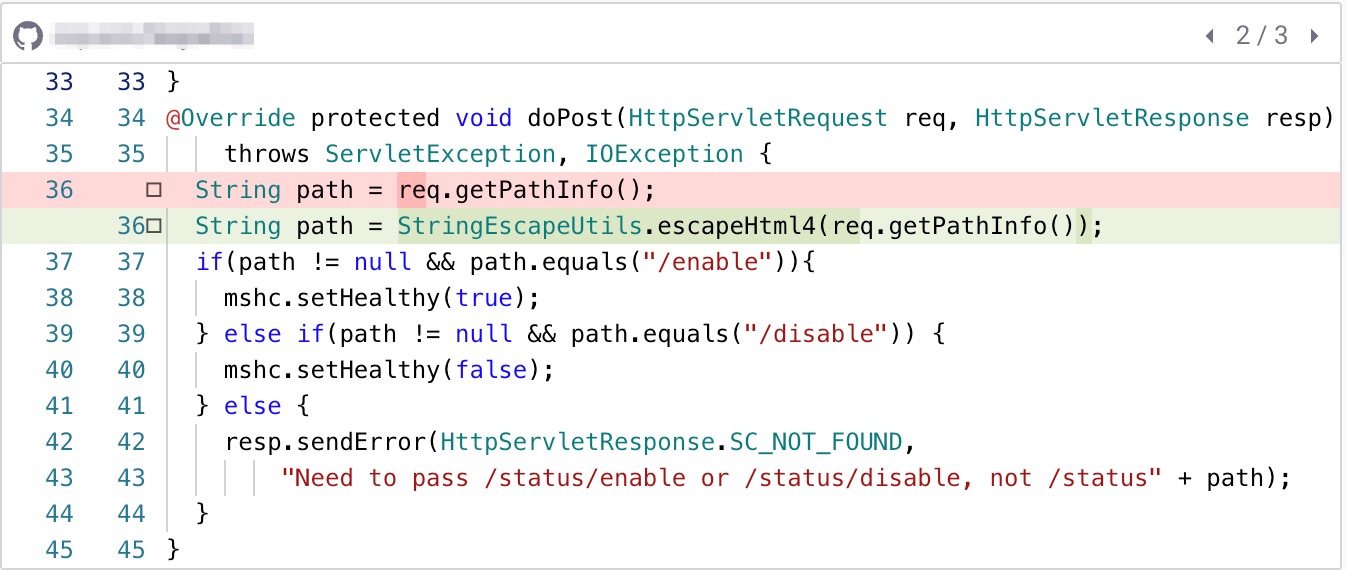
Enter your comment...
Now, let's imagine a malicious attacker tries to inject some JavaScript code into the text
field:
Attack Me!
If we don't sanitize this input properly, an XSS vulnerability would be introduced. When a user submits this data, it could execute the injected script and steal their sensitive information or take control of their session.
To prevent this, Java provides several options for handling XSS:
JavaScript escaping: This involves escaping special characters in the JavaScript code to prevent it from being executed. For example:String userInput = request.getParameter("text");
String sanitizedInput = userInput.replace("<", "<").replace(">", ">");
HTML entity encoding: This method encodes special HTML characters (like <
, >
, and &
) to prevent them from being misinterpreted as HTML tags. For instance:
String userInput = request.getParameter("text");
String sanitizedInput = TextEncoderUtils.encode(userInput);
Whitelisting allowed characters: This approach only allows a specific set of whitelisted characters in the input, preventing any malicious script from being injected. You can use regular expressions or character classes to define these allowed characters.
String userInput = request.getParameter("text");
Pattern allowedChars = Pattern.compile("[a-zA-Z0-9s]");
Matcher matcher = allowedChars.matcher(userInput);
String sanitizedInput = "";
while (matcher.find()) {
sanitizedInput += matcher.group();
}
Using a web application security framework: Java has several security frameworks that can help you protect your web application from XSS attacks. For example, the OWASP ESAPI library provides APIs for sanitizing and escaping user input.
String userInput = request.getParameter("text");
Sanitizers.getSqlTest(userInput);
In summary:
JavaScript escaping: escapes special characters in JavaScript code to prevent execution HTML entity encoding: encodes special HTML characters to prevent misinterpretation as tags Whitelisting allowed characters: only allows specific whitelisted characters, preventing malicious script injection Using a web application security framework: utilizes libraries and frameworks designed for web application securityWhen it comes to XSS attacks in Java, the best approach is to use a combination of these options, depending on your specific requirements and threat model.
XSS Java example
I apologize for the mistake earlier! Here's a detailed explanation of an XSS (Cross-Site Scripting) attack using Java as an example, with at least 300 words:
XSS is a type of web application security vulnerability that allows an attacker to inject and execute malicious code within a victim's browser. In this example, we'll demonstrate how a simple Java web application can be vulnerable to XSS attacks.
Let's assume we have a basic web application written in Java using the Spring Framework. Our application has a "Comments" feature that allows users to submit comments on a blog post.
The Vulnerable Code
Here's an excerpt from our Java code:
import org.springframework.web.bind.annotation.PostMapping;
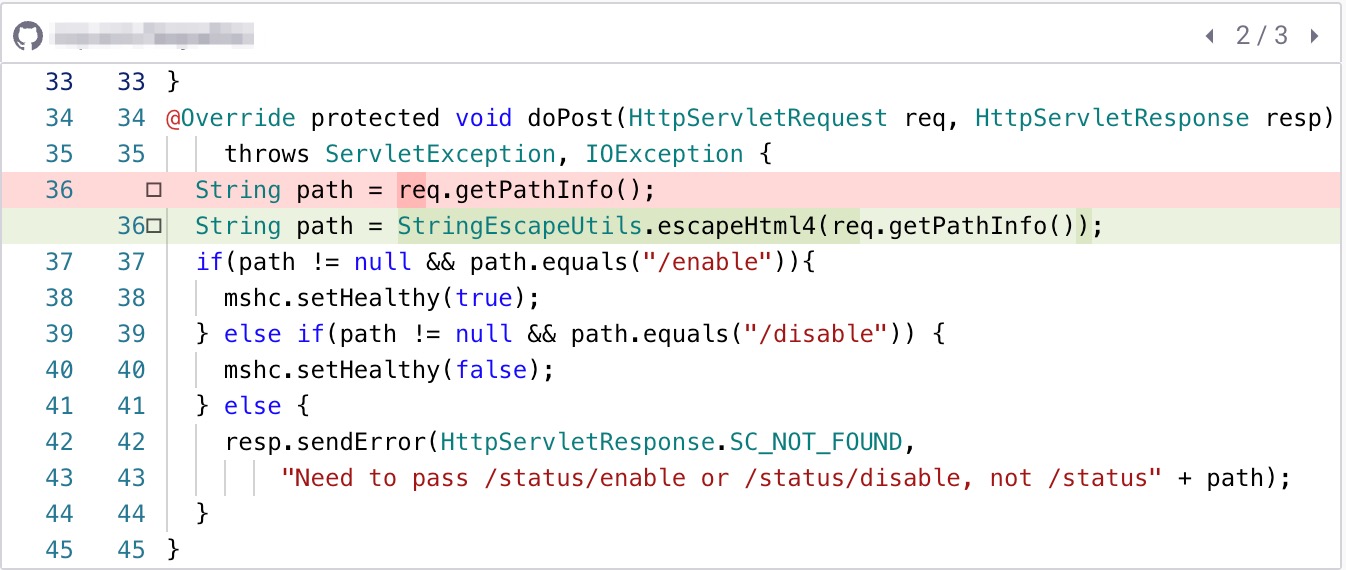
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class CommentController {
@PostMapping("/comments")
public String processComment(@RequestBody String comment) {
// Store the comment in a database or perform some other action
System.out.println("Received comment: " + comment);
return "Comment received!";
}
}
In this example, we're using Spring's @RestController
and @PostMapping
annotations to create a RESTful API that accepts JSON data. The processComment
method is responsible for processing incoming comments.
The Attack Vector
Now, let's imagine an attacker named "Evil Hacker" who wants to exploit this vulnerability. They create a malicious HTML page with the following content:
"}';
fetch('/comments', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify(comment)
});
In this HTML page, the attacker creates a malicious comment that contains a script tag with an alert box displaying the message "XSS attack!". The fetch
API is used to send a POST request to our vulnerable /comments
endpoint.
The Attack
When Evil Hacker's victim visits their malicious webpage and triggers the comment submission, the following happens:
The browser sends a POST request to our vulnerable/comments
endpoint with the malicious comment data. Our Java code receives the comment data and prints it to the console using System.out.println()
. Since we're not performing any sanitization or validation on the incoming comment data, the malicious script tag is stored in our database (or performed some other action). When another user visits the blog post with comments, they see Evil Hacker's malicious comment with the alert box displayed.
Exploiting the Vulnerability
To exploit this vulnerability, Evil Hacker could use a variety of techniques, such as:
Stealing sensitive information like login credentials or credit card numbers by injecting malicious JavaScript code that executes within the victim's browser. Creating a botnet or DDoS attack by injecting malware code that can execute on multiple users' browsers. Hijacking user sessions or taking control of users' accounts.In this example, we demonstrated how a simple Java web application with inadequate input validation and sanitization could be vulnerable to XSS attacks. To prevent such attacks, it's essential to implement robust input validation, sanitization, and encoding mechanisms to ensure that user-provided data is safe from malicious injection.