Socket programming in Java example
Socket programming in Java example
Here is an example of socket programming in Java:
Server Side:
import java.io.IOException;
import java.net.ServerSocket;
import java.net.Socket;
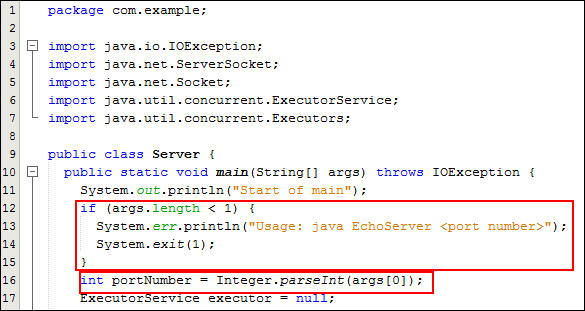
public class Server {
public static void main(String[] args) throws IOException {
ServerSocket server = new ServerSocket(8000);
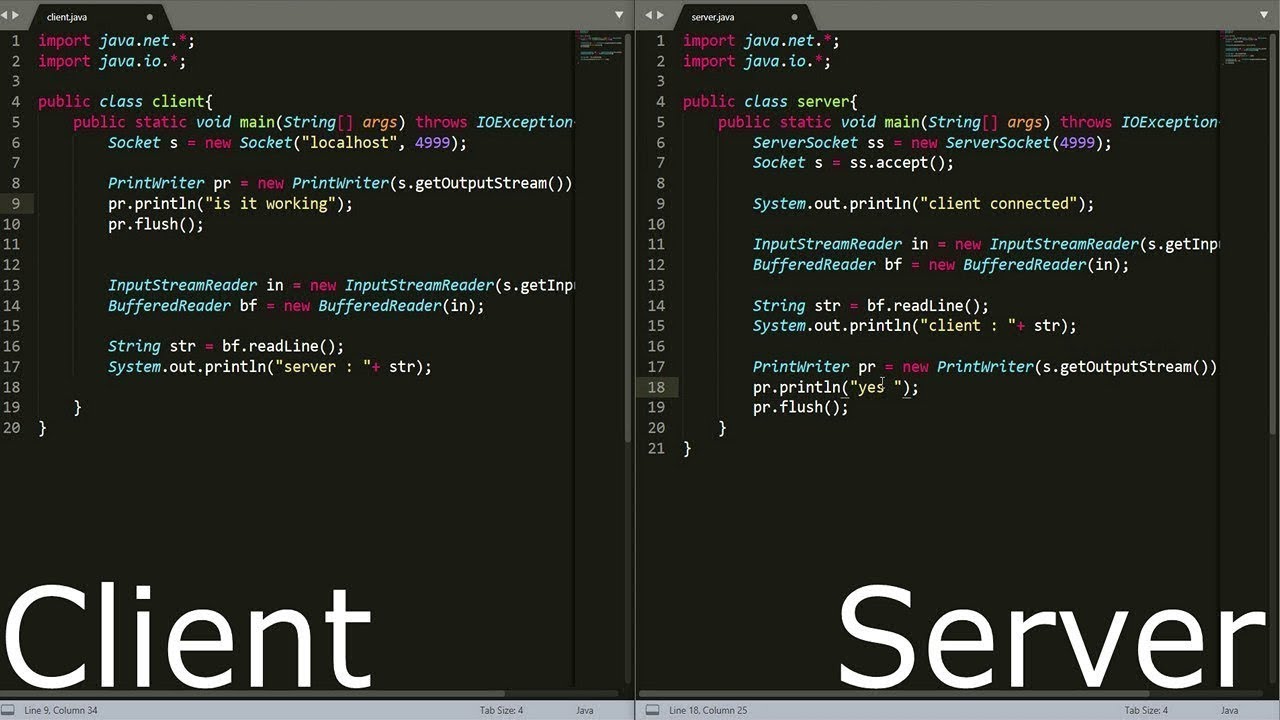
System.out.println("Server is running...");
while (true) {
Socket client = server.accept();
// Create a separate thread for each client connection
Thread t = new Thread(new ClientHandler(client));
t.start();
}
}
private static class ClientHandler implements Runnable {
private final Socket socket;
public ClientHandler(Socket socket) {
this.socket = socket;
}
@Override
public void run() {
try {
// Read from client and write to the console
java.io.BufferedReader in = new java.io.BufferedReader(new java.io.InputStreamReader(socket.getInputStream()));
String inputLine;
while ((inputLine = in.readLine()) != null) {
System.out.println("Received: " + inputLine);
}
} catch (IOException e) {
// Handle exception
} finally {
try {
socket.close();
} catch (IOException e) {
// Handle exception
}
}
}
}
}
Client Side:
import java.io.*;
import java.net.*;
public class Client {
public static void main(String[] args) throws IOException {
Socket socket = new Socket("localhost", 8000);
PrintWriter out = new PrintWriter(socket.getOutputStream(), true);
BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
// Send a message to the server
out.println("Hello from client!");
String inputLine;
while ((inputLine = in.readLine()) != null) {
System.out.println("Received: " + inputLine);
}
socket.close();
}
}
In this example, we have a simple echo server and client implemented using Java sockets. The server runs continuously and listens for incoming connections on port 8000. When a connection is established, it creates a separate thread to handle the client communication.
The client initiates a connection with the server, sends a message ("Hello from client!"), and then receives any responses from the server until the end of the transmission.
Key Concepts:
Socket creation: In both the server and client sides, we create a socket object usingnew Socket()
or new ServerSocket()
. The latter is used to create a listening socket, while the former creates an outgoing socket. I/O Streams: We use I/O streams (e.g., PrintWriter
, BufferedReader
) to read from and write to sockets. These streams are used for both sending and receiving data over the network. Multithreading: In the server example, we create a new thread for each client connection using new Thread(new ClientHandler(client))
. This allows multiple clients to be handled concurrently without blocking the main thread.
Advantages:
Concurrent handling of multiple connections: Java's threading mechanism enables us to handle multiple client connections simultaneously, improving system responsiveness. Flexibility in I/O operations: Using I/O streams for socket communication provides flexibility and ease of use when handling different types of data (e.g., text, binary). Robust error handling: We can incorporate robust error handling mechanisms using try-catch blocks to handle exceptions and ensure reliable communication.This example demonstrates the basics of socket programming in Java, showcasing key concepts and advantages.
How to make connection server to port using Java socket programming
I'm happy to help!
Java Socket Programming allows developers to create network-based applications that can connect to servers and clients, facilitating communication between them. To establish a connection from a client (your application) to a server on a specific port, you'll need to follow these steps:
Step 1: Create a ServerSocket object
Create a ServerSocket
object by specifying the port number you want to listen to:
int port = 8080; // choose your desired port
ServerSocket serverSocket = new ServerSocket(port);
Step 2: Listen for incoming connections
Use the accept()
method of the ServerSocket
object to start listening for incoming connections:
Socket clientSocket = serverSocket.accept();
The accept()
method blocks until a connection is established. When a connection is made, it returns a Socket
object representing the connection.
Step 3: Establish a connection
Create a new Socket
object to establish a connection from your client (your application) to the server:
String host = "localhost"; // or replace with your server's hostname/IP address
int port = 8080; // match the server's listening port
SocketAddress remoteSocketAddress = new InetSocketAddress(host, port);
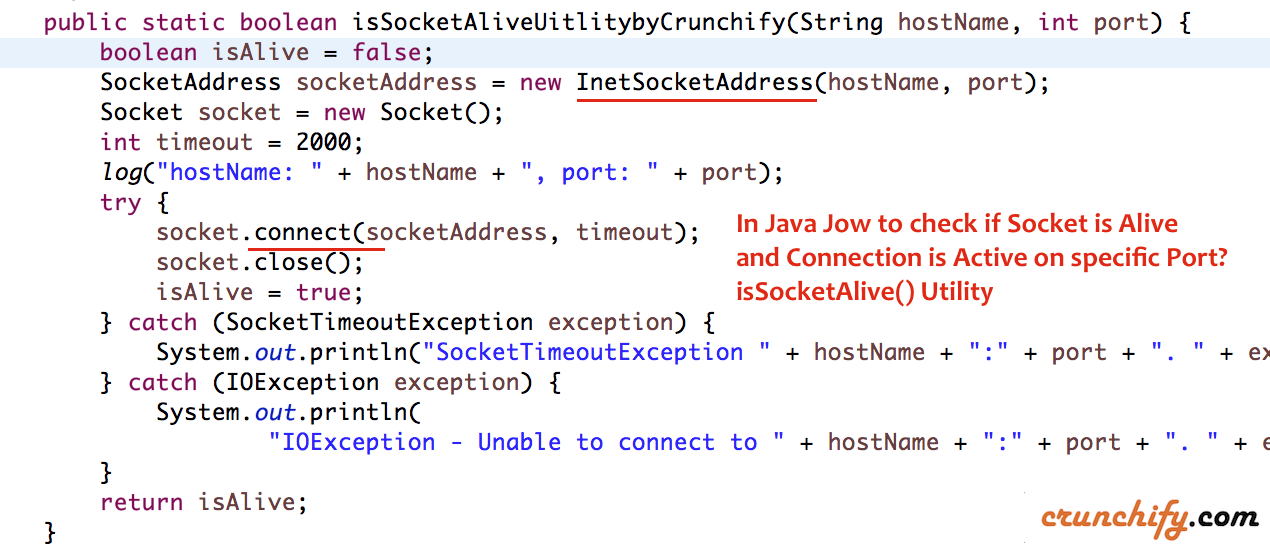
Socket socket = new Socket(remoteSocketAddress);
Step 4: Read and write data
To send and receive data between the client and server, use methods like read()
and write()
:
// sending data from client to server
byte[] dataToSend = "Hello Server!".getBytes();
socket.getOutputStream().write(dataToSend);
// receiving data from server to client
byte[] dataReceived = new byte[1024];
int bytesRead = socket.getInputStream().read(dataReceived);
Step 5: Close the connection
When you're done with the connection, close the Socket
objects using methods like close()
:
socket.close();
clientSocket.close();
Here's a simple example of connecting to a server from a client (your application) using Java Socket Programming:
public class Client {
public static void main(String[] args) {
String host = "localhost"; // or replace with your server's hostname/IP address
int port = 8080; // match the server's listening port
try (
Socket socket = new Socket(host, port);
BufferedReader reader = new BufferedReader(new InputStreamReader(socket.getInputStream()));
PrintWriter writer = new PrintWriter(socket.getOutputStream(), true)
) {
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
// Send message to server
writer.println("Hello Server!");
writer.flush();
} catch (UnknownHostException e) {
System.out.println("Host not found: " + e.getMessage());
} catch (IOException e) {
System.out.println("Error connecting to host: " + e.getMessage());
}
}
}
And on the server side:
public class Server {
public static void main(String[] args) throws IOException {
int port = 8080; // match the client's connection port
try (ServerSocket serverSocket = new ServerSocket(port);
Socket socket = serverSocket.accept();
BufferedReader reader = new BufferedReader(new InputStreamReader(socket.getInputStream()));
PrintWriter writer = new PrintWriter(socket.getOutputStream(), true)
) {
String line;
while ((line = reader.readLine()) != null) {
System.out.println("Received from client: " + line);
}
// Send response back to client
writer.println("Hello Client!");
writer.flush();
} catch (IOException e) {
System.out.println("Error accepting connection: " + e.getMessage());
}
}
}
In this example, the client sends a message ("Hello Server!") and receives any responses from the server. The server listens for incoming connections on port 8080, accepts a connection, and reads lines sent by the client.
Remember to replace "localhost"
with your actual server's hostname/IP address, and adjust the port numbers as needed.
Hope this helps you get started with Java Socket Programming!