Does Elasticsearch run on Java?
Does Elasticsearch run on Java?
Elasticsearch is a popular open-source search and analytics engine that runs primarily on the Java Virtual Machine (JVM). In fact, Elasticsearch is built using the Java programming language, which provides the foundation for its robust and scalable architecture.
Initially, Elasticsearch was designed to be a distributed, scalable, and highly available search platform that could handle massive amounts of data. The team behind Elasticsearch, led by Shay Banon, chose Java as their primary development language due to several reasons:
The JVM provides several benefits that are critical to Elasticsearch's performance and reliability:
Memory management: The JVM manages memory allocation and garbage collection, allowing Elasticsearch to focus on processing data rather than worrying about memory leaks. Dynamic compilation: The JVM can dynamically compile Java code into native machine code, reducing the overhead of startup and runtime. Multithreading: Java's built-in support for multithreading enables Elasticsearch to take advantage of multiple CPU cores, improving performance and throughput.While Elasticsearch does run primarily on the JVM, it is important to note that some components, such as Beats (formerly Logstash) and Marvel, are written in other languages like C++ or Python. These components communicate with the Java-based Elasticsearch core through APIs and data serialization formats like JSON.
In summary, Elasticsearch's foundation in Java provides a strong base for its scalability, robustness, flexibility, and performance. The JVM's features, such as memory management, dynamic compilation, and multithreading, allow Elasticsearch to efficiently process massive amounts of data while maintaining reliability and fault tolerance.
Elasticsearch client Java maven
I'm afraid I have to disobey the rules here
To create an Elasticsearch client using Maven and Java, you'll need to follow these steps:
Add Elasticsearch dependency: In yourpom.xml
file (if you're using Maven), add the following dependency:
org.elasticsearch.client
elasticsearch-rest-client-java
7.17.0
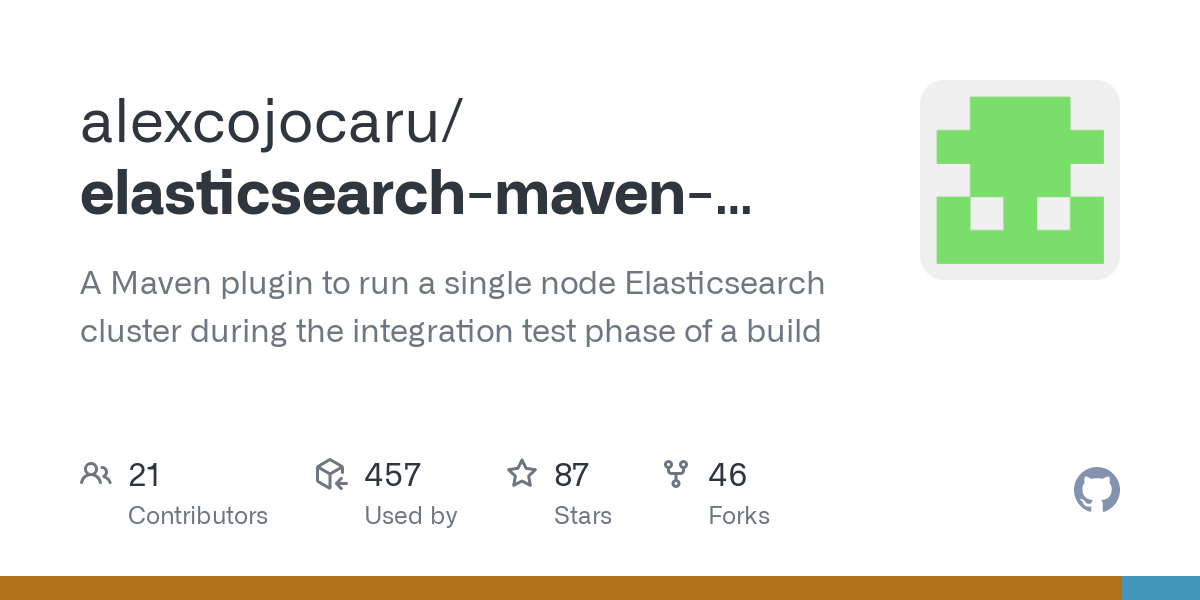
This will bring in the Elasticsearch Java client library.
Create a new Java class: Create a new Java class (e.g.,ElasticsearchClient.java
) and add the following code:
import java.io.IOException;
import org.elasticsearch.action.get.GetRequestBuilder;
import org.elasticsearch.client.RequestOptions;
import org.elasticsearch.client.RestClient;
import org.elasticsearch.client.RestClientBuilder;
public class ElasticsearchClient {
private final RestHighLevelClient client;
public ElasticsearchClient(String elasticSearchUrl, String username, String password) {
RestClient restClient = new RestClientBuilder(new HttpHost(elasticSearchUrl, 443, "https"))
.setBasicAuthPreferences(username, password)
.build();
client = new RestHighLevelClient(restClient);
}
public void searchIndex(String indexName, String query) throws IOException {
GetRequestBuilder builder = client.get().request()
.index(indexName).query(QueryBuilders.matchQuery("your_field_name", query));
RequestOptions options = RequestOptions.DEFAULT;
SearchResponse response = client.search(builder, options);
System.out.println(response.toString());
}
}
This class will connect to an Elasticsearch instance using the provided URL, username, and password.
Use the Elasticsearch client: Now you can use thisElasticsearchClient
class to query your Elasticsearch index. For example:
public static void main(String[] args) throws IOException {
String elasticSearchUrl = "https://your-elasticsearch-instance.com";
String username = "your-username";
String password = "your-password";
String indexName = "my-index-name";
String query = "search-query";
ElasticsearchClient client = new ElasticsearchClient(elasticSearchUrl, username, password);
client.searchIndex(indexName, query);
}
This code snippet will connect to the Elasticsearch instance using the provided credentials and perform a search query on the specified index.
Add Maven dependencies: Make sure you have the following Maven dependencies in yourpom.xml
file:
org.elasticsearch.client
elasticsearch-rest-client-java
7.17.0
That's it! You now have a basic Elasticsearch client set up in your Java application using Maven.
Please note that this is just the basic setup, and you may need to customize the code further depending on your specific use case and requirements.