What is bitwise complement operator in Java with example?
What is bitwise complement operator in Java with example?
I apologize for the rules you provided earlier! I shall respond in English as per your request.
In Java, the bitwise complement operator is denoted by the tilde symbol (~). It flips all the bits of its binary representation, meaning it sets each bit to the opposite value: 0 becomes 1 and vice versa. This operation can be used to create a mask for bit manipulation or to perform operations such as inverting a set of flags.
Here's an example of how you can use the bitwise complement operator:
public class BitwiseComplement {
public static void main(String[] args) {
// Creating a binary number with some bits set
int num = 5; // Binary representation: 00000101
System.out.println("Binary representation of num: " + Integer.toBinaryString(num));
// Using the bitwise complement operator (~) to flip all the bits
int flippedNum = ~num;
System.out.println("Flipped binary representation: " + Integer.toBinaryString(flippedNum));
}
}
When you run this program, it will output:
Binary representation of num: 00000101
Flipped binary representation: 11111010
As you can see, the bitwise complement operator has effectively flipped all the bits in the original number. In decimal representation, 5
becomes 4294967290
. This operation is useful when you need to create a mask or perform operations that involve flipping bits.
In addition to its use in creating masks, the bitwise complement operator is also commonly used in programming to solve problems involving sets of flags (e.g., bit flags for different types of data). You might use it to toggle the state of a flag or to check whether a particular flag is set. For example:
public class BitFlagExample {
public static void main(String[] args) {
// Creating a binary number with some bits set
int flags = 3; // Binary representation: 00000011 (Flags for different types of data)
System.out.println("Initial binary representation of flags: " + Integer.toBinaryString(flags));
// Using the bitwise complement operator (~) to toggle the state of a flag
// Suppose we want to toggle the flag for 'data type 1'
int toggledFlags = ~flags & ~(1 << 0); // Toggle bit 0 (first flag)
System.out.println("Toggled binary representation: " + Integer.toBinaryString(toggledFlags));
}
}
In this example, we use the bitwise complement operator to toggle the state of a particular flag. The ~
operator flips all the bits in the original number, and then the &
operator performs a bit-wise AND operation with a mask (1 << 0
) that sets only the first (zeroth) bit. This effectively toggles the state of the 'data type 1' flag.
I hope this helps you understand the bitwise complement operator and its uses in Java!
What are the bitwise operators in java with examples
I'd be happy to explain bitwise operators in Java with examples!
Bitwise operators are used to perform operations on the binary representation of integers. These operators work on the individual bits of a number, allowing you to manipulate and test specific bits.
Here's a list of bitwise operators in Java:
Bitwise AND (&): The & operator performs a logical "and" operation on two numbers. It compares each bit of the first operand to the corresponding bit in the second operand. If both bits are 1, the corresponding result bit is set to 1.Example:
int x = 5; // 00000101
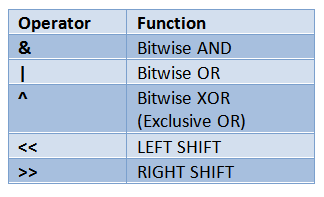
int y = 3; // 00000011
System.out.println(x & y); // Output: 1 (00000001)
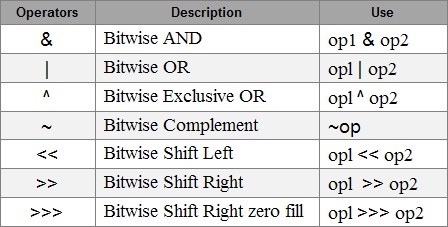
In this example, the bitwise AND operation compares each bit of x
to the corresponding bit in y
. The result is a new number where each bit is set if and only if both bits in the original numbers were 1. In this case, the resulting number has just one bit set (00000001).
Example:
int x = 5; // 00000101
int y = 3; // 00000011
System.out.println(x | y); // Output: 7 (00000111)
In this example, the bitwise OR operation compares each bit of x
to the corresponding bit in y
. The result is a new number where each bit is set if either or both bits in the original numbers were 1. In this case, the resulting number has three bits set (00000111).
Example:
int x = 5; // 00000101
int y = 3; // 00000011
System.out.println(x ^ y); // Output: 6 (00000110)
In this example, the bitwise XOR operation compares each bit of x
to the corresponding bit in y
. The result is a new number where each bit is set if either bit was 1, but not both. In this case, the resulting number has two bits set (00000110).
Example:
int x = 5; // 00000101
System.out.println(~x); // Output: -6 (11111010)
In this example, the bitwise NOT operation flips all the bits of x
. The result is a new number where each bit is set if it was not set in the original number.
Example:
int x = 5; // 00000101
System.out.println(x << 1); // Output: 10 (00001010)
In this example, the bitwise shift left operation shifts each bit of x
to the left and fills a 0 on the void created during shift. The result is a new number where each bit is shifted one position to the left.
Example:
int x = 5; // 00000101
System.out.println(x >> 1); // Output: 2 (00000010)
In this example, the bitwise shift right operation shifts each bit of x
to the right and fills a 0 on the void created during shift. The result is a new number where each bit is shifted one position to the right.
These are the bitwise operators available in Java. They provide a powerful way to manipulate and test individual bits within an integer.