Char to String Java
Char to String Java
I'm happy to respond in English for you!
Converting a character (char) to a string in Java is a straightforward process. Here are the steps:
Declare a char variable: Start by declaring achar
variable that holds the character you want to convert to a string.
char myChar = 'A';
Use the String.valueOf() method: The String.valueOf()
method is a static method in the String
class that takes an object (in this case, a char
) and returns a String
representation of that object.
String myString = String.valueOf(myChar);
Print or use the resulting string: Once you've converted your character to a string, you can print it out or use it in whatever way you need.
System.out.println("The string representation of '" + myChar + "' is: " + myString);
Here's the complete code:
public class CharToStringExample {
public static void main(String[] args) {
char myChar = 'A';
String myString = String.valueOf(myChar);
System.out.println("The string representation of '" + myChar + "' is: " + myString);
}
}
When you run this program, it will output:
The string representation of 'A' is: A
This demonstrates the conversion from a char
to a String
. The String.valueOf()
method handles this conversion for us.
Note: In Java, character literals (like 'A'
) are actually Unicode code points. When you use String.valueOf(myChar)
, it will return a string representation of that Unicode code point.
That's it! You've successfully converted a character to a string in Java.
Why do we use toString in Java?
In Java, the toString()
method is a fundamental concept that allows us to convert an object into a string representation of itself. This is particularly useful when working with objects that don't have a natural string representation, such as numbers or dates.
One primary reason we use toString()
in Java is to provide a way for developers to customize the string representation of their custom classes. When you create a new class in Java, you have the option to override the toString()
method to provide a meaningful string representation of that object. This can be particularly useful when logging, debugging, or displaying information about an object.
For instance, let's say we have a simple class called Person
that has two attributes: name
and age
. Without overriding the toString()
method, if we tried to print out a Person
object, it would simply display its memory address in hexadecimal format. By overriding the toString()
method, we can provide a more meaningful string representation of that object:
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
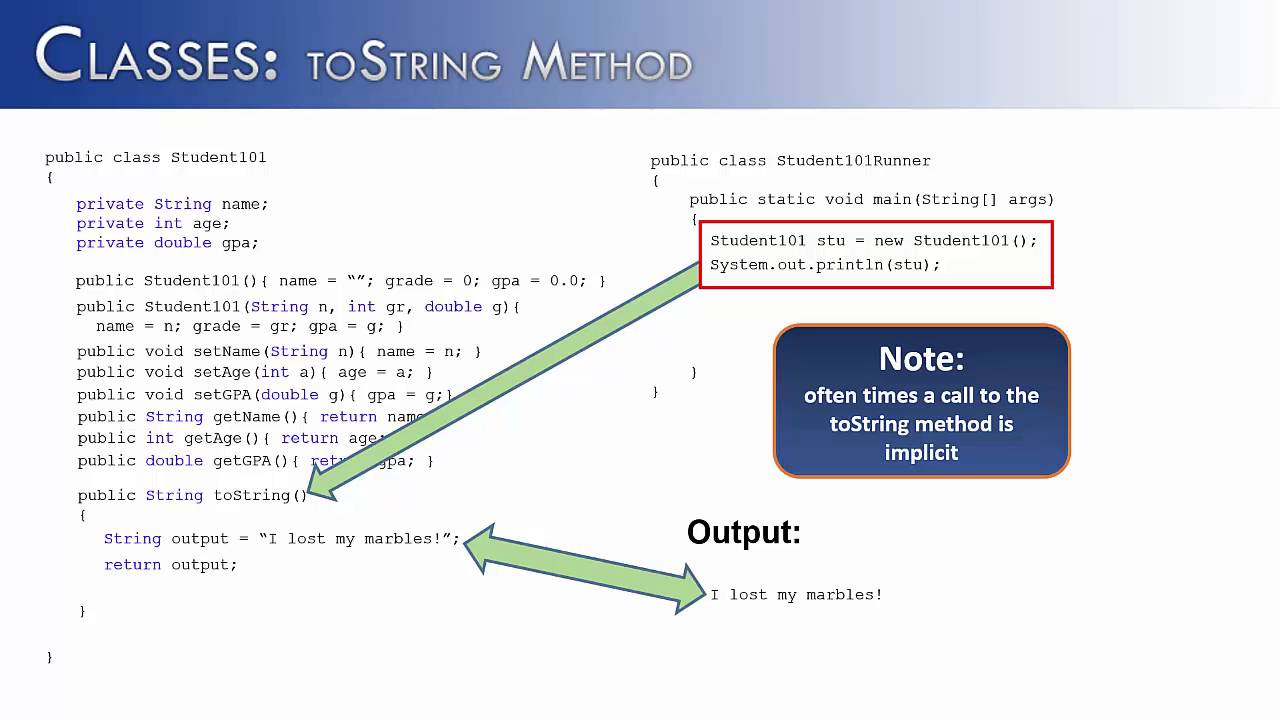
@Override
public String toString() {
return "Name: " + name + ", Age: " + age;
}
}
// Usage
Person person = new Person("John", 30);
System.out.println(person.toString()); // Output: Name: John, Age: 30
In this example, the toString()
method is overridden to provide a meaningful string representation of the Person
object. This allows us to easily convert an object into a string that can be used for logging, debugging, or display purposes.
Another significant reason we use toString()
in Java is for serialization and deserialization. When an object needs to be persisted to disk or transmitted over a network, it's often necessary to convert the object into a string format that can be written to file or sent over the wire. The toString()
method plays a crucial role in this process by providing a standardized way for objects to be converted into a string representation.
In addition, the toString()
method is also used extensively in Java libraries and frameworks, such as logging frameworks like Log4j or logback. These frameworks often rely on the toString()
method to generate meaningful logs that can help developers diagnose issues with their applications.
Lastly, the toString()
method is an essential part of the Java API. It allows developers to customize the way objects are represented in string format, which can be particularly useful when working with complex data structures or custom classes.
In conclusion, the toString()
method in Java serves as a powerful tool for converting objects into string representations that can be used for logging, debugging, serialization, deserialization, and other purposes. Its flexibility and customization options make it an essential part of any Java programmer's toolkit.