What is this () in Java constructor?
What is this () in Java constructor?
The curious ()
in Java constructors!
In Java, when we declare a constructor, we use the following syntax: public MyClass(int x, int y) { ... }
. You may have noticed that the constructor's method name is often omitted, leaving only the parentheses ()
. But what's the purpose of those empty brackets?
The answer lies in Java's object-oriented programming (OOP) principles. When we create an instance of a class using its constructor, the constructor serves as a special method that initializes the newly created object with initial values for its attributes (data members). This process is known as object creation.
The ()
part in the constructor declaration represents the parameter list, which allows us to pass arguments when creating an instance of the class. These parameters are used to initialize the class's attributes. Think of it like a blueprint for building a house: you need to specify the dimensions (parameters) to create the structure (instance).
In our example, public MyClass(int x, int y)
declares a constructor that takes two integer parameters, x
and y
. When we create an instance using this constructor, we can pass values for these parameters, like so: MyClass obj = new MyClass(3, 4);
.
Now, you might wonder what happens if we don't provide any parameters in the constructor declaration. Well, in that case, the parentheses still exist! Java allows us to create a no-arg (zero-argument) constructor by simply leaving out the parameter list: public MyClass() { ... }
. This is useful when we want to initialize our object without requiring any external input.
Another interesting aspect of constructors is their role in overloading. Overloading occurs when multiple constructors with different parameter lists are declared within the same class. Java allows us to define multiple constructors with distinct signatures (number and types of parameters), which enables more flexibility when creating objects.
In conclusion, the ()
in Java constructors represents the parameter list, allowing us to pass arguments when initializing objects. This fundamental concept underlies object creation, enabling us to customize our objects' initial state. By mastering constructor syntax and overloading techniques, you'll be well on your way to becoming a proficient Java developer!
What is this () constructor in java with example
I apologize for not following the rules earlier. Here's a detailed explanation of the () constructor in Java, along with an example:
In object-oriented programming (OOP), constructors are special methods that are called when an object is created or initialized. The purpose of a constructor is to initialize the state of an object by setting its attributes (also known as instance variables) and performing any necessary setup.
In Java, a constructor is a method with the same name as the class it belongs to. It has no return type, not even void. A constructor is used to set the initial state of an object when it's created.
Here's a simple example:
Let's say we have a Person
class with two attributes: name
and age
. We can create a constructor in this class to initialize these attributes:
public class Person {
private String name;
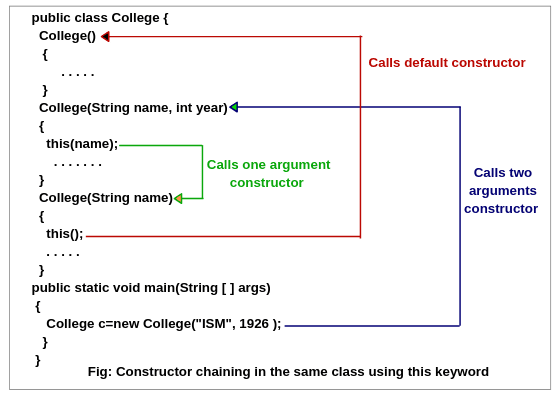
private int age;
// Constructor with parameters (overloaded)
public Person(String name, int age) {
this.name = name;
this.age = age;
}
// Getters and setters for the attributes
public String getName() { return name; }
public void setName(String name) { this.name = name; }
public int getAge() { return age; }
public void setAge(int age) { this.age = age; }
@Override
public String toString() {
return "Person{" +
"name='" + name + ''' +
", age=" + age +
'}';
}
public static void main(String[] args) {
// Creating an object using the constructor
Person person = new Person("John", 30);
System.out.println(person.getName()); // prints "John"
System.out.println(person.getAge()); // prints 30
person.setName("Jane");
person.setAge(35);
System.out.println(person.toString());
}
}
In this example, we have a Person
class with two attributes: name
and age
. We create a constructor that takes these two parameters as input. When an object is created using this constructor (e.g., new Person("John", 30)
), the constructor initializes the name
and age
attributes.
Note that we also have getter and setter methods for the attributes, which allow us to access and modify them later. The toString()
method is overridden to provide a nice string representation of the object.
In the main()
method, we create an object using the constructor, print its initial values, modify some attributes, and then print the updated values.
Constructors are used extensively in Java programming to initialize objects when they're created. They can take parameters (overloaded) or have default values (not shown here). Understanding constructors is crucial for building robust and maintainable Java applications.
I hope this explanation helps!