How do you handle exceptions in Java?
How do you handle exceptions in Java?
Handling exceptions in Java is a crucial part of writing robust and reliable code. Java provides several mechanisms for handling exceptions, which are:
try {
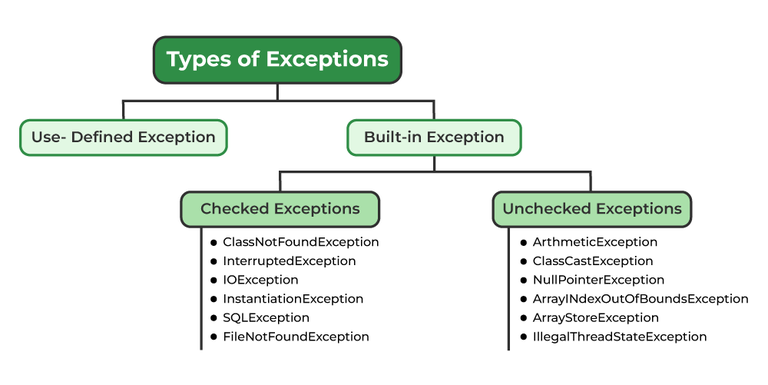
// Code that might throw an exception
} catch (ExceptionType e) {
// Code to handle the exception
}
Finally Block: A finally block is used to execute code regardless of whether an exception was thrown or not. It's often used for cleaning up resources such as closing files or database connections.
try {
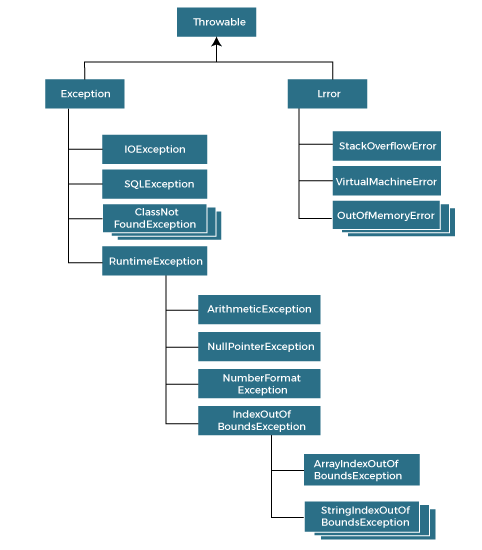
// Code that might throw an exception
} catch (ExceptionType e) {
// Code to handle the exception
} finally {
// Code that will always run
}
Throwing Exceptions: You can also use the throw
keyword to explicitly throw an exception. This is often used when you need to re-throw an exception that has already been caught. Multi-Catch Blocks: Java 7 introduced multi-catch blocks, which allow you to catch multiple exceptions in a single catch clause.
try {
// Code that might throw an exception
} catch (ExceptionType1 | ExceptionType2 e) {
// Code to handle the exception
}
Custom Exceptions: Java allows you to create your own custom exceptions by extending the Exception
class or implementing the Throwable
interface.
When deciding how to handle exceptions in Java, you should consider the following factors:
Type of Exception: Is it a runtime exception that can be handled with try-catch blocks, or is it a compile-time error that needs to be addressed with annotations like@SuppressWarnings
? Frequency and Impact: How often does this exception occur, and how much impact will it have on your application if left unhandled? Code Readability: How will handling this exception affect the readability of your code?
Best practices for handling exceptions in Java include:
Don't Swallow Exceptions: Avoid using empty catch blocks that simply re-throw the exception or ignore it. This can lead to hidden bugs and make debugging more difficult. Use Meaningful Exception Messages: When creating custom exceptions, provide meaningful error messages that help developers understand what went wrong. Handle Exceptions Near Their Point of Origin: Try to handle exceptions as close to their point of origin as possible. This makes it easier to debug and maintain your code.In summary, Java provides several mechanisms for handling exceptions, including try-catch blocks, finally blocks, throwing exceptions, multi-catch blocks, and custom exceptions. By considering the type, frequency, and impact of an exception, you can choose the best approach for handling it in a way that improves the readability and maintainability of your code.
What is meant by exception in Java?
In Java programming language, an exception represents an unforeseen event that occurs during the execution of a program, which disrupts its normal flow. Think of it like an unexpected obstacle that suddenly appears on your road trip, requiring you to take a detour.
Exceptions are used to handle and manage situations where your code might not be able to proceed normally due to various reasons such as:
When an exception occurs, the execution of your program is interrupted, and control passes to a special block of code designed to handle the situation: the catch
block. This block is part of a larger construct called a try-catch
statement.
A try-catch
statement typically consists of three parts:
try
and catch
blocks have completed.
Here's a simple example of how you might use a try-catch
statement:
public class Calculator {
public int divide(int numerator, int denominator) {
try {
return numerator / denominator;
} catch (ArithmeticException e) { // handle division by zero or invalid values
System.out.println("Error: Division by zero!");
return 0; // or throw a new exception if you prefer
}
}
}
In this example, the divide
method is wrapped within a try-catch
statement to handle potential arithmetic exceptions (like division by zero). If an exception occurs during execution (e.g., when denominator is zero), control passes to the catch
block, where you can take corrective actions or log errors.
Why use exceptions in Java?
Error handling: Exceptions provide a structured way to handle runtime errors and unexpected situations. Code robustness: By anticipating potential issues and coding for them, your program becomes more resilient and less prone to crashing. Debugging ease: With exceptions, you can quickly identify and diagnose issues during debugging, making it easier to fix problems.In summary, exceptions in Java represent unpredictable events that disrupt normal program execution. Using try-catch
statements effectively helps manage these unexpected situations, allowing your code to recover from errors and continue running smoothly.