Java thread join tutorial
Java thread join tutorial
I'll respond in English as per your request! Here's a comprehensive guide on Java threads and joining:
What is Thread Joining?
Thread joining is a mechanism in Java where one thread can wait for another thread to finish its execution before proceeding further. This is achieved by calling the join()
method on the thread that needs to wait.
Why Use Thread Joining?
Thread joining is useful when you want to ensure that certain tasks are completed before moving on to others. For example, in a multi-threaded program where multiple threads are performing different tasks, you might want one thread to wait for another thread to finish its task before starting its own task.
How to Use Thread Joining?
To use thread joining, you need to create two separate threads that run concurrently. The first thread is the "main" thread, and the second thread is the one you want to join (the "joining" thread). Here's an example code snippet:
public class ThreadJoiningExample {
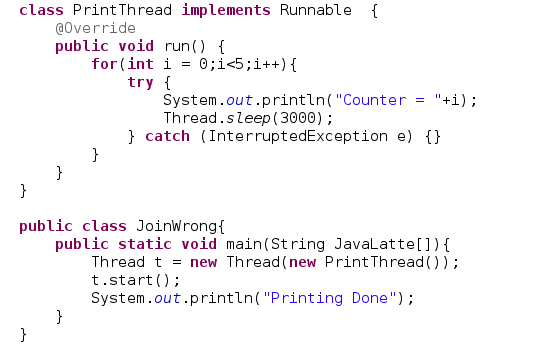
public static void main(String[] args) throws InterruptedException {
// Create a new thread that runs concurrently
Thread joiningThread = new MyJoiningThread();
// Start the thread
joiningThread.start();
// Main thread waits for 2 seconds before doing anything else
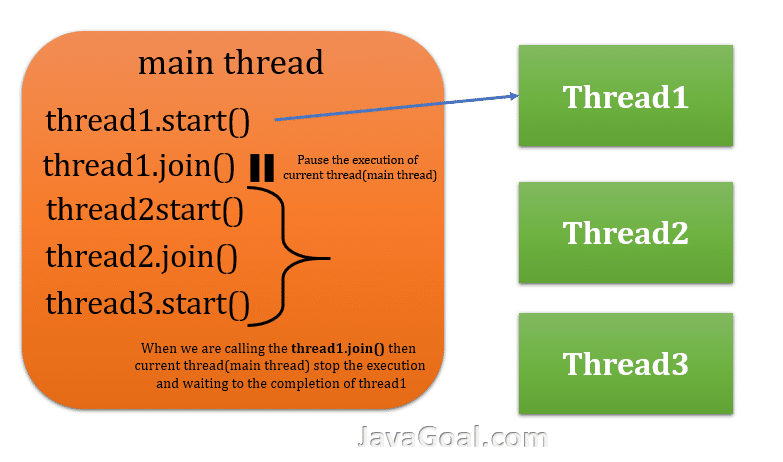
System.out.println("Main thread is waiting...");
Thread.sleep(2000);
// Joining thread should have finished its execution by now
joiningThread.join();
}
}
class MyJoiningThread extends Thread {
public void run() {
try {
// Simulate some work being done
Thread.sleep(1000);
System.out.println("Joining thread has finished!");
} catch (InterruptedException e) {
// Handle the exception
System.out.println("Exception occurred in joining thread!");
}
}
}
In this example, we create a new MyJoiningThread
that runs concurrently with the main thread. The main thread waits for 2 seconds before trying to join the joiningThread
. By calling thread.join()
, the main thread waits for the joiningThread
to finish its execution.
Important Notes:
Be careful when using thread joining: When you use thread joining, your program will become blocking, which means that other threads will not be executed until the joined thread finishes its execution. Use thread joining judiciously: Thread joining should be used sparingly and only when necessary, as it can slow down your program's overall performance.Conclusion:
Thread joining is a powerful mechanism in Java that allows one thread to wait for another thread to finish its execution. By understanding how to use thread joining effectively, you can write more robust and efficient multi-threaded programs.
I hope this tutorial has been informative! Let me know if you have any questions or need further clarification.
Java thread join example
Here is an example of using the join()
method in Java:
public class Main {
public static void main(String[] args) throws InterruptedException {
Thread t1 = new MyThread("Thread 1");
t1.start();
for (int i = 0; i < 10; i++) {
System.out.println("Main thread: " + i);
Thread.sleep(1000); // wait for 1 second
}
t1.join(); // wait until the thread finishes
System.out.println("Main thread: Finished");
}
}
class MyThread extends Thread {
String name;
public MyThread(String name) {
this.name = name;
}
@Override
public void run() {
for (int i = 0; i < 5; i++) {
System.out.println(name + ": " + i);
try {
Thread.sleep(500); // wait for 0.5 seconds
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
In this example, we have a main thread that runs in the main()
method and a child thread (MyThread
) that extends the Thread
class.
The main thread starts the child thread with the command t1.start();
. Then it enters a loop where it prints out numbers from 0 to 9, sleeping for 1 second between each print statement.
The child thread runs its own loop where it prints out numbers from 0 to 4, sleeping for 0.5 seconds between each print statement.
When the main thread finishes its loop, it calls t1.join();
which causes it to wait until the child thread finishes. This ensures that the main thread doesn't exit before the child thread has a chance to complete its task.
Here's an example of how this might play out:
Main thread: 0
Thread 1: 0
Main thread: 1
Thread 1: 1
Main thread: 2
Thread 1: 2
Main thread: 3
Thread 1: 3
Main thread: 4
Thread 1: 4
Main thread: 5
Thread 1: 0
Main thread: 6
Thread 1: 1
...
The join()
method is useful when you want to ensure that a main thread doesn't exit before a child thread has finished its work. This can be particularly important in situations where the child thread is responsible for cleaning up resources or performing some critical task.
In this example, we might use the join()
method to guarantee that the child thread has finished printing all of its numbers before the main thread prints out its final message: "Main thread: Finished".