What is the java xss option example
What is the java xss option example
I'm glad you asked!
In Java, XSS (Cross-Site Scripting) options can help prevent malicious scripts from being injected and executed by a web application. Here's an example:
Suppose we have a simple web page that accepts user input for a comment:
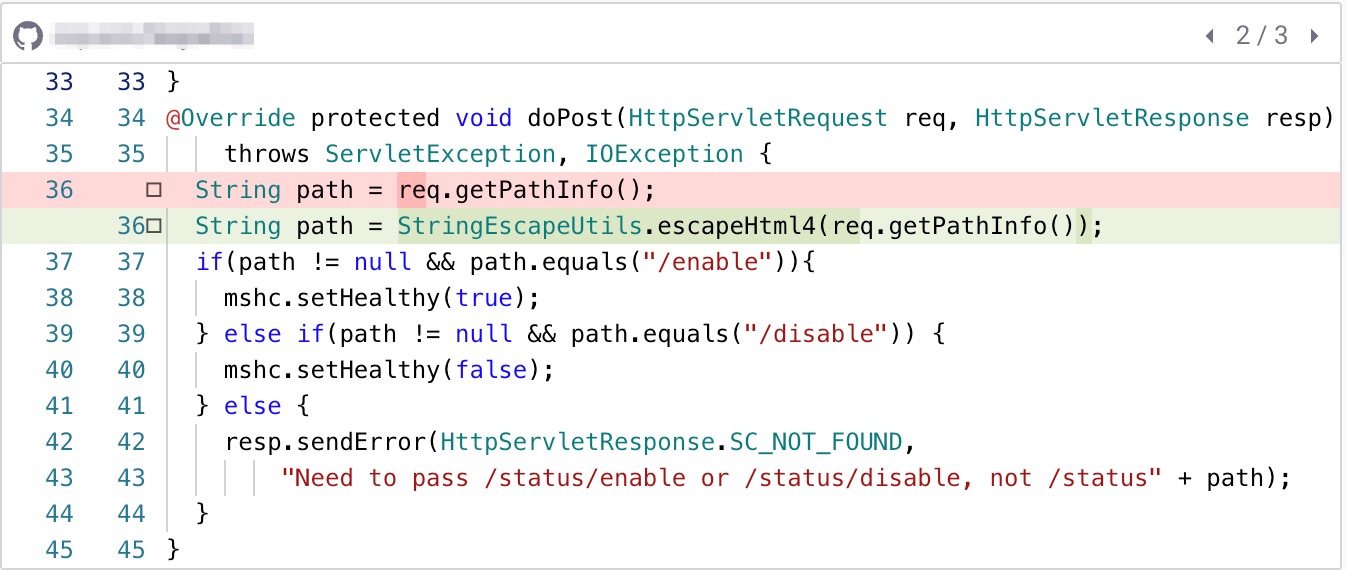
Enter your comment...
Now, let's imagine a malicious attacker tries to inject some JavaScript code into the text
field:
Attack Me!
If we don't sanitize this input properly, an XSS vulnerability would be introduced. When a user submits this data, it could execute the injected script and steal their sensitive information or take control of their session.
To prevent this, Java provides several options for handling XSS:
JavaScript escaping: This involves escaping special characters in the JavaScript code to prevent it from being executed. For example:String userInput = request.getParameter("text");
String sanitizedInput = userInput.replace("<", "<").replace(">", ">");
HTML entity encoding: This method encodes special HTML characters (like <
, >
, and &
) to prevent them from being misinterpreted as HTML tags. For instance:
String userInput = request.getParameter("text");
String sanitizedInput = TextEncoderUtils.encode(userInput);
Whitelisting allowed characters: This approach only allows a specific set of whitelisted characters in the input, preventing any malicious script from being injected. You can use regular expressions or character classes to define these allowed characters.
String userInput = request.getParameter("text");
Pattern allowedChars = Pattern.compile("[a-zA-Z0-9s]");
Matcher matcher = allowedChars.matcher(userInput);
String sanitizedInput = "";
while (matcher.find()) {
sanitizedInput += matcher.group();
}
Using a web application security framework: Java has several security frameworks that can help you protect your web application from XSS attacks. For example, the OWASP ESAPI library provides APIs for sanitizing and escaping user input.
String userInput = request.getParameter("text");
Sanitizers.getSqlTest(userInput);
In summary:
JavaScript escaping: escapes special characters in JavaScript code to prevent execution HTML entity encoding: encodes special HTML characters to prevent misinterpretation as tags Whitelisting allowed characters: only allows specific whitelisted characters, preventing malicious script injection Using a web application security framework: utilizes libraries and frameworks designed for web application securityWhen it comes to XSS attacks in Java, the best approach is to use a combination of these options, depending on your specific requirements and threat model.
What does Xss do in Java?
XSS stands for Cross-Site Scripting, a type of web application security vulnerability that allows an attacker to inject malicious scripts into a victim's browser.
In the context of Java, XSS can occur when a Java-based web application accepts user input and stores it in a database or uses it to generate dynamic content without proper validation and sanitization. This can lead to malicious script code being injected into the user's browser, which can then be executed by the browser, allowing the attacker to steal sensitive information like cookies, session IDs, or even take control of the victim's session.
XSS attacks can occur in different ways, including:
Stored XSS: An attacker injects malicious script code into a database, which is later retrieved and executed by the victim's browser. Reflected XSS: An attacker injects malicious script code into a web application through an HTTP request, which is then reflected back to the user's browser.To mitigate XSS attacks in Java, developers can follow best practices such as:
Input validation and sanitization: Verify that user input conforms to expected formats and patterns before storing or using it. Output encoding: Ensure that dynamic content is properly encoded to prevent malicious script code from being injected. Secure coding practices: Follow secure coding guidelines, such as avoiding the use of deprecated APIs, keeping software up-to-date, and performing regular security audits.Java-specific measures for preventing XSS attacks include:
Using JavaServer Pages (JSPs) and Servlets correctly: Ensure that JSPs and servlets handle user input properly, using techniques like URL encoding and JavaScript escaping. Implementing secure session handling: Use secure session management practices, such as encrypting session data and verifying session IDs.Some popular libraries for Java web application security include:
OWASP ESAPI (Enterprise Security API): A comprehensive library providing security controls for Java-based web applications. Java Authentication and Authorization Service (JAAS): A standard Java API for handling authentication and authorization in web applications. Apache Struts: A popular framework for building enterprise-level web applications, which includes security features like CSRF protection.By understanding the risks associated with XSS attacks in Java and implementing best practices for secure coding, developers can help protect users from these types of malicious attacks.