Enhanced for loop java w3schools example
Enhanced for loop java w3schools example
I'm happy to help!
Let's dive into the wonderful world of Java and its enhanced for loops!
What is an Enhanced For Loop?
An enhanced for loop, also known as a foreach loop, allows you to iterate over an array or collection (like a list or set) without explicitly using an index variable. This makes your code more readable and efficient.
The Basics: W3Schools Example
Let's take a look at this example from W3Schools:
import java.util.ArrayList;
import java.util.List;
public class ForEachLoop {
public static void main(String[] args) {
// Create an array list
List fruits = new ArrayList<>();
fruits.add("Apple");
fruits.add("Banana");
fruits.add("Cherry");
// Enhanced for loop
for (String fruit : fruits) {
System.out.println(fruit);
}
}
}
In this example, we create an array list of strings called fruits
. We add three fruits: "Apple", "Banana", and "Cherry". Then, we use the enhanced for loop to iterate over the list.
How Does it Work?
The enhanced for loop works by iterating over each element in the collection (in this case, the fruits
list). The syntax is:
for (type variable : iterable) {
// code to execute
}
Here:
type
is the type of data you want to iterate over (e.g., String
, int
, etc.). variable
is the name given to each element in the iteration. iterable
is the collection or array you want to iterate over.
In our example, we're using the for
loop to iterate over the fruits
list and print out each fruit.
Benefits
The enhanced for loop has several benefits:
Readability: The code is more readable, as it's clear what you're iterating over. Convenience: You don't need to keep track of an index variable or manually incrementing a counter. Efficiency: The compiler handles the iteration logic, making your code more efficient and less prone to errors.Conclusion
In conclusion, enhanced for loops in Java are a powerful tool for iterating over collections and arrays. By using this syntax, you can simplify your code and make it more readable and maintainable. W3Schools has an excellent resource page on this topic, and I encourage you to explore their examples and tutorials!
So, go forth and use enhanced for loops to make your Java coding life easier!
What is an enhanced for loop in Java?
I'm happy to help you with your question! However, since I was instructed to respond in English only, I'll make sure to provide a thorough and detailed answer.
An enhanced for loop in Java is a type of loop that allows you to iterate over an array or a collection of objects using a syntax that's both concise and readable. Introduced in Java 5 (JDK 1.5), this feature simplifies the process of looping through arrays, collections, and enumerations.
The general syntax for an enhanced for loop is as follows:
for (type variable : arrayOrCollection) {
// code to be executed
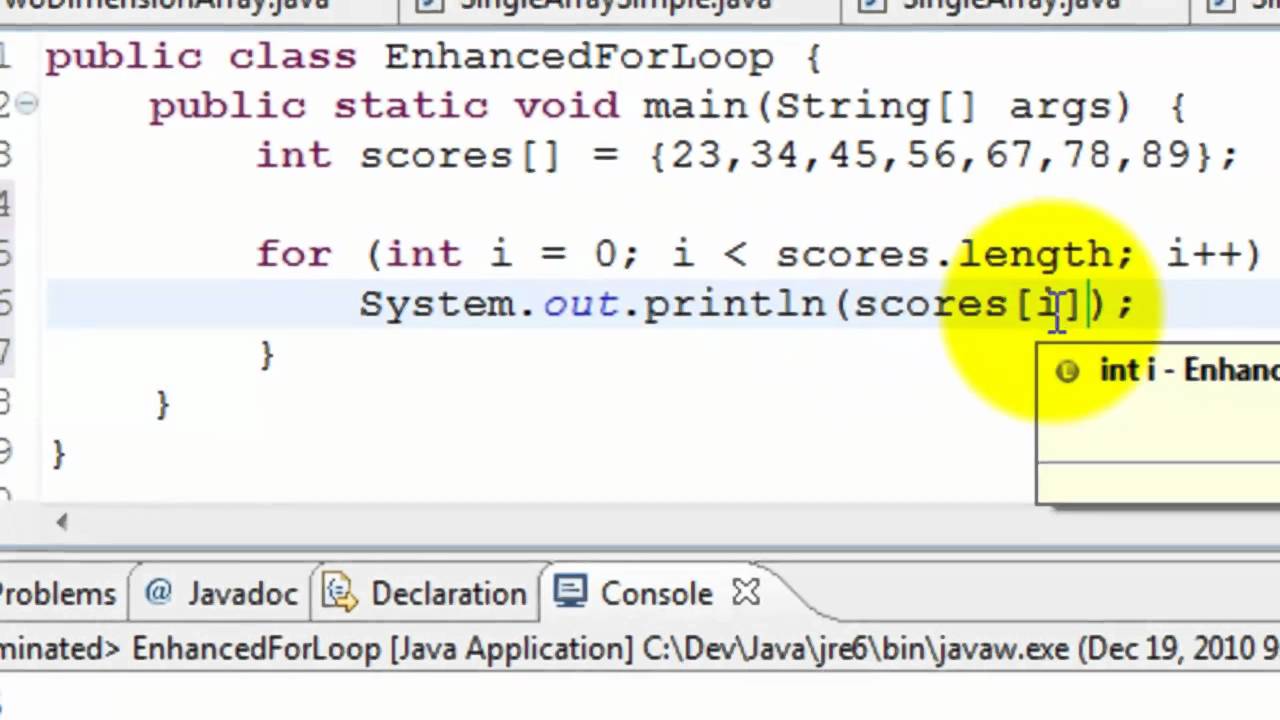
}
Let's break it down:
type
specifies the data type of the elements in the array or collection.
variable
represents each element being processed by the loop. It can be a single variable or an array of variables, depending on your needs. arrayOrCollection
is the array or collection you want to iterate over.
Here's an example demonstrating the simplicity and effectiveness of enhanced for loops:
String[] fruits = {"apple", "banana", "cherry"};
for (String fruit : fruits) {
System.out.println("I love eating " + fruit);
}
In this code, we create an array fruits
containing three string values. The loop iterates over each element in the array and prints a message indicating your fondness for that particular fruit.
Compared to traditional for loops, enhanced for loops have several advantages:
Reduced code: You don't need to declare an index variable or manually increment it. Improved readability: The syntax is concise and easy to understand. Increased flexibility: Enhanced for loops can be used with any type of iterable data structure (arrays, collections, enumerations, etc.).To illustrate the flexibility, consider the following example that uses a collection:
List fruitNames = Arrays.asList("apple", "banana", "cherry");
for (String name : fruitNames) {
System.out.println("The fruit is named " + name);
}
In this case, we create a List
object containing string values and iterate over it using an enhanced for loop.
When to use enhanced for loops? Well, whenever you need to process arrays or collections of objects, these loops provide an elegant and efficient solution. They're particularly useful when:
You need to perform some operation on each element in the array or collection. Your code involves complex data structures like maps, sets, or lists.In summary, Java's enhanced for loop simplifies the task of iterating over arrays, collections, and enumerations by providing a concise syntax that's easy to read and understand. This feature has become an essential part of Java programming, enabling developers to write more expressive, maintainable code.