Java DOM parser example
Java DOM parser example
Here is a Java DOM parser example using the Apache Xerces2 implementation:
import org.apache.xerces.parsers.DOMParser;
import org.apache.xerces.parsers.XMLParser;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.NodeList;
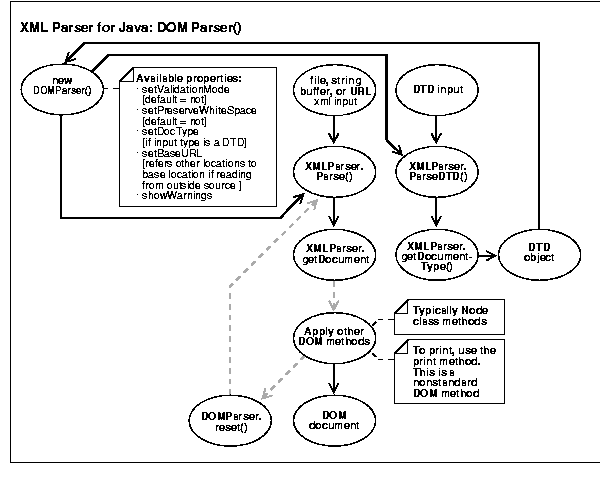
public class DomParserExample {
public static void main(String[] args) {
try {
// Create a DOM parser
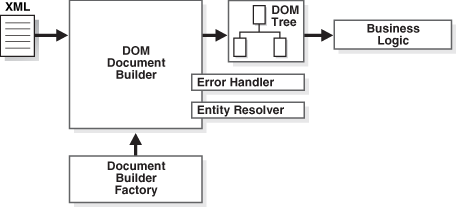
DOMParser parser = new DOMParser();
parser.parse("example.xml"); // Parse the XML file
// Get the document from the parser
Document doc = parser.getDocument();
// Get all elements with tag name "employee"
NodeList list = doc.getElementsByTagName("employee");
for (int i = 0; i < list.getLength(); i++) {
Element employeeElement = (Element) list.item(i);
// Get the attributes of the element
String idAttr = employeeElement.getAttribute("id");
String nameAttr = employeeElement.getAttribute("name");
System.out.println("Employee ID: " + idAttr);
System.out.println("Employee Name: " + nameAttr);
NodeList children = employeeElement.getChildNodes();
for (int j = 0; j < children.getLength(); j++) {
Node node = children.item(j);
if (node instanceof Element) {
Element childElement = (Element) node;
// Get the text content of the element
String childText = childElement.getTextContent();
System.out.println("Child Text: " + childText);
}
}
}
} catch (Exception e) {
System.out.println("Error parsing XML: " + e.getMessage());
}
}
}
This example demonstrates how to parse an XML file using the Apache Xerces2 library and work with its contents. The code parses the "example.xml" file and extracts information about employees, including their IDs, names, and child elements.
First, it creates a DOMParser
object and uses it to parse the XML file. Then, it gets all elements with the tag name "employee" from the parsed document.
For each employee element, it retrieves its attributes (id and name) and prints them out. It also extracts all child nodes of the employee element and iterates over them, printing their text content if they are elements themselves.
This code can be used as a starting point for parsing XML files in Java and extracting information from them.
Note that this is just one example of how to use DOM parsers in Java, and you may need to adapt it to your specific requirements.
Which is the best XML parser in Java?
I'd be happy to help you with that!
In Java, there are several excellent XML parsers available, each with its strengths and weaknesses. Here's a rundown of some of the most popular ones:
JAXP (Java API for XML Processing): This is not actually an XML parser itself, but rather a set of APIs for parsing and manipulating XML documents in Java. JAXP provides a layer of abstraction between your code and the underlying XML parser, making it easy to switch between different parsers.Some popular implementations of JAXP include:
Saxon: A high-performance XML processor that's widely used in industry. It's particularly strong at handling complex XPath expressions. Xalan-J: A Java-based implementation of the W3C XSLT and XPath standards. It provides a robust set of tools for processing XML documents. DOM4J: A popular, lightweight, and easy-to-use XML parser that's specifically designed for Java. DOM4J provides a simple, intuitive API for parsing and manipulating XML documents.Pros: Easy to use, flexible, supports XSLT transformations.
Cons: Not as fast as some other parsers, particularly Saxon.
XStream: A high-performance XML parser that's designed for efficient serialization of Java objects into XML strings. It's also capable of deserializing XML into Java objects.Pros: Fast and efficient, easy to use, supports streaming parsing.
Cons: Not suitable for complex XPath expressions or XSLT transformations.
XOM (XML Object Model): A lightweight, flexible, and highly customizable XML parser that provides a simple API for parsing and manipulating XML documents.Pros: Easy to extend, supports XSLT transformations, fast and efficient.
Cons: Not as widely used or supported as some other parsers.
JiBX: A Java-based implementation of the JiBX (Java to Binary) schema compiler. It allows you to map Java objects directly to binary formats, including XML.Pros: Fast and efficient, supports binary serialization, easy to use.
Cons: Not specifically designed for XML parsing, can be complex to set up.
In conclusion, the "best" XML parser in Java depends on your specific requirements and constraints. If you need a high-performance parser with strong support for XPath expressions and XSLT transformations, Saxon is likely a good choice. If you prefer a lightweight, easy-to-use parser, DOM4J might be more suitable. For fast and efficient serialization of Java objects into XML strings, XStream could be the way to go.
Remember that each parser has its strengths and weaknesses, so it's essential to evaluate them based on your project's specific needs and constraints.