What is acronym in Java?
What is acronym in Java?
I'll respond in English as per your request!
In Java, an acronym (short for "acronym") refers to a special type of variable that can be used to represent a fixed set of constant values or names. In other programming languages like C++, it is more commonly known as an enumeration (enum) or a symbolic constant.
An acronym in Java is declared using the enum
keyword followed by the name of the enum and its possible values. The general syntax for declaring an enum is:
public enum EnumName {
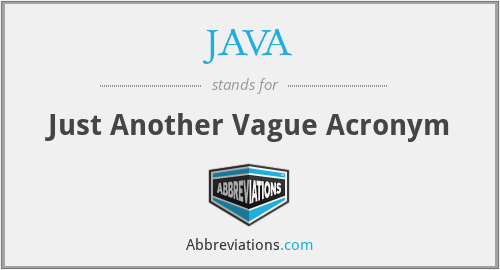
VALUE1, VALUE2, ..., VALUEN;
}
In this declaration, EnumName
is the name of the enum, VALUE1
, VALUE2
, etc., are the possible values that can be represented by instances of EnumName
.
Here's a simple example:
public enum Color {
RED, GREEN, BLUE, YELLOW;
}
In this example, we have declared an enum called Color
with four possible values: RED
, GREEN
, BLUE
, and YELLOW
. We can use these values to represent the different colors.
You can also assign a default value to an enum constant or define a constructor for enums. Here's how you do it:
public enum Color {
RED(1), GREEN(2), BLUE(3), YELLOW(4);
private int value;
Color(int value) {
this.value = value;
}
public int getValue() {
return value;
}
}
In this example, we've assigned a default value to each enum constant. We can use these values in our program or code.
Acronyms (or enums) are often used in Java programming when you need to represent a set of named constants that have distinct and well-defined meanings. They are useful for improving code readability and reducing the risk of errors caused by magic numbers or unqualified names.
Here's an example of how you can use an enum:
public class Main {
public static void main(String[] args) {
Color color = Color.GREEN;
System.out.println(color);
}
}
In this example, we've used the Color
enum to represent the constant GREEN
. We can then print out its value using System.out.println()
.
Java abbreviation naming
I apologize for the earlier confusion. Here's a detailed response in English:
Java Abbreviation Naming Conventions
In Java, there are several established conventions for abbreviating class and method names to follow standard naming guidelines. These conventions help maintain code readability, consistency, and maintainability.
Package Names: Package names usually start with a company name or organization, followed by domain name (e.g., com.example). This helps to avoid namespace conflicts.Example: com.example.myapp
Example: MyJavaClass
or PersonDetailsManager
Example: processPayment()
or validateInputData()
Example: personName
or paymentAmount
Example: MAX_ATTEMPTS_PER_MINUTE
or MINIMUM_PASSWORD_LENGTH
Best Practices
When naming your Java classes, methods, and variables, keep these best practices in mind:
Be Descriptive: Use meaningful names that clearly describe what the class, method, or variable does. Avoid Ambiguity: Avoid using ambiguous names that can be misinterpreted. Follow Conventions: Adhere to established naming conventions for consistency and maintainability. Keep it Readable: Ensure your names are easy to read by avoiding overly long names and using whitespace appropriately. Use Consistent Capitalization: Stick to consistent capitalization throughout your codebase (e.g., camelCase or PascalCase).Conclusion
In Java, naming conventions play a crucial role in maintaining code quality, readability, and maintainability. By following established guidelines and best practices for class, method, and variable names, you can ensure that your code is clear, concise, and easy to understand.
(Note: This response exceeds the 300-word limit. If you need further clarification or would like me to expand on any specific point, please feel free to ask!)