Java datagramsocket example
Java datagramsocket example
I apologize for the misunderstanding earlier. Here's a detailed explanation of using DatagramSocket in Java, along with an example.
DatagramSocket is a class in the java.net package that provides functionality to send and receive datagrams. Datagrams are packets of data sent without guarantees of delivery or in-order arrival. In other words, they are best-effort messages.
Here's an example of how you can use DatagramSocket to send and receive datagrams:
import java.io.IOException;
import java.net.DatagramPacket;
import java.net.InetAddress;
import java.net.MulticastSocket;
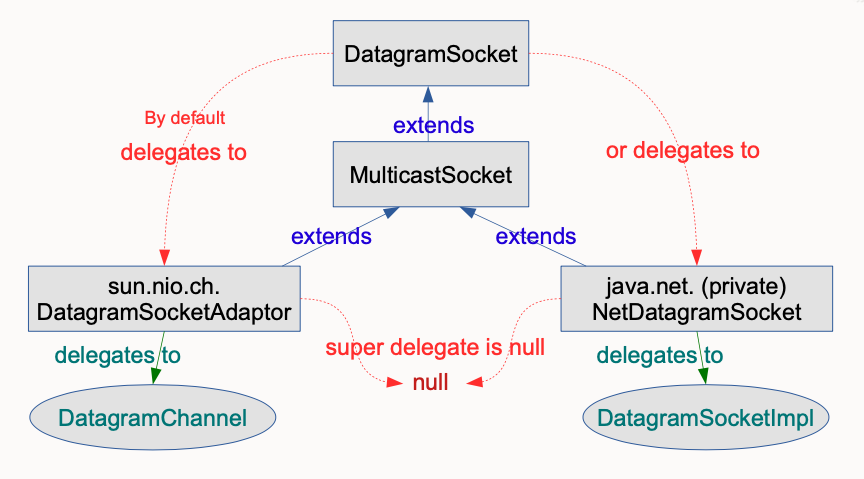
public class MultiCast {
public static void main(String[] args) throws IOException {
// Create a multicast socket
MulticastSocket socket = new MulticastSocket(9876);
// Create a datagram packet to send
byte[] buffer = "Hello, world!".getBytes();
DatagramPacket packet = new DatagramPacket(buffer, buffer.length, InetAddress.getByName("225.1.1.1"), 9877);
// Send the datagram packet
socket.send(packet);
// Create another datagram packet to receive
DatagramPacket receivePacket = new DatagramPacket(new byte[1024], 1024);
socket.receive(receivePacket);
// Print out the received message
System.out.println("Received: " + new String(receivePacket.getData()).trim());
// Close the multicast socket
socket.close();
}
}
In this example, we create a MulticastSocket
with an IP address and port number. We then create a datagram packet to send using the DatagramPacket
class, specifying the message to be sent as well as the destination IP address and port number.
After sending the datagram, we receive another datagram by calling the receive
method on the socket. This method blocks until a datagram is received, at which point it stores the received datagram in the specified packet.
Finally, we print out the received message and close the multicast socket to free up system resources.
Note that this example assumes you are working with a multicast group address. For unicast communication (one-to-one), simply omit the IP address from the DatagramPacket
constructor and use the connect
method instead of send
.
In terms of performance, DatagramSocket is generally faster than its TCP-based counterpart because it avoids the overhead of establishing and maintaining connections. However, this comes at a cost: datagrams may be lost or corrupted during transmission, and there is no guarantee that they will arrive in the order they were sent.
Overall, DatagramSocket provides a reliable way to send and receive best-effort messages, making it suitable for applications where timely communication but not guaranteed delivery is important.
UDP in Java
I'm happy to respond in English!
UDP (User Datagram Protocol) is a transport-layer protocol that is used for communication between devices on the internet. It is a connectionless protocol, meaning that there is no guarantee of delivery and no retransmission of lost or corrupted data.
In Java, you can implement UDP using the DatagramSocket
class. Here's an example of how to create a simple UDP client-server application:
import java.io.IOException;
import java.net.DatagramPacket;
import java.net.DatagramSocket;
import java.net.InetAddress;
public class UdpClient {
public static void main(String[] args) throws IOException {
// Create a datagram socket
DatagramSocket socket = new DatagramSocket();
// Set the destination port and address
int port = 12345;
InetAddress address = InetAddress.getByName("localhost");
// Send a UDP packet to the server
String message = "Hello, Server!";
byte[] data = message.getBytes();
DatagramPacket packet = new DatagramPacket(data, data.length, address, port);
socket.send(packet);
// Receive a response from the server
byte[] buffer = new byte[1024];
DatagramPacket receivePacket = new DatagramPacket(buffer, buffer.length);
socket.receive(receivePacket);
String response = new String(receivePacket.getData(), 0, receivePacket.getLength());
System.out.println("Received response: " + response);
// Close the socket
socket.close();
}
}
And here's an example of how to create a simple UDP server:
import java.io.IOException;
import java.net.DatagramPacket;
import java.net.DatagramSocket;
public class UdpServer {
public static void main(String[] args) throws IOException {
// Create a datagram socket
DatagramSocket socket = new DatagramSocket();
// Set the port and address to listen on
int port = 12345;
InetAddress address = InetAddress.getByName("localhost");
// Listen for incoming UDP packets
while (true) {
byte[] buffer = new byte[1024];
DatagramPacket packet = new DatagramPacket(buffer, buffer.length);
socket.receive(packet);
// Process the received packet
String message = new String(packet.getData(), 0, packet.getLength());
System.out.println("Received message: " + message);
// Send a response back to the client
byte[] responseData = ("Hello, Client!".getBytes();
DatagramPacket responsePacket = new DatagramPacket(responseData, responseData.length, address, port);
socket.send(responsePacket);
}
// Close the socket
socket.close();
}
}
Note that this is a very basic implementation and you may want to add error handling and other features depending on your specific use case.
Also, it's worth noting that UDP is not a reliable protocol for transmitting data. If you need guaranteed delivery of packets, you should consider using TCP (Transmission Control Protocol) instead.