How to use MongoDB query in Java?
How to use MongoDB query in Java?
To use a MongoDB query in Java, you can utilize the official MongoDB Java driver. The MongoDB Java driver provides a way for your Java application to interact with a MongoDB database.
Here are the steps:
Step 1: Add the MongoDB Java Driver to Your Project
The first step is to add the MongoDB Java driver to your project. You can do this by adding the following dependency to your pom.xml
file (if you're using Maven) or your build.gradle
file (if you're using Gradle):
Maven:
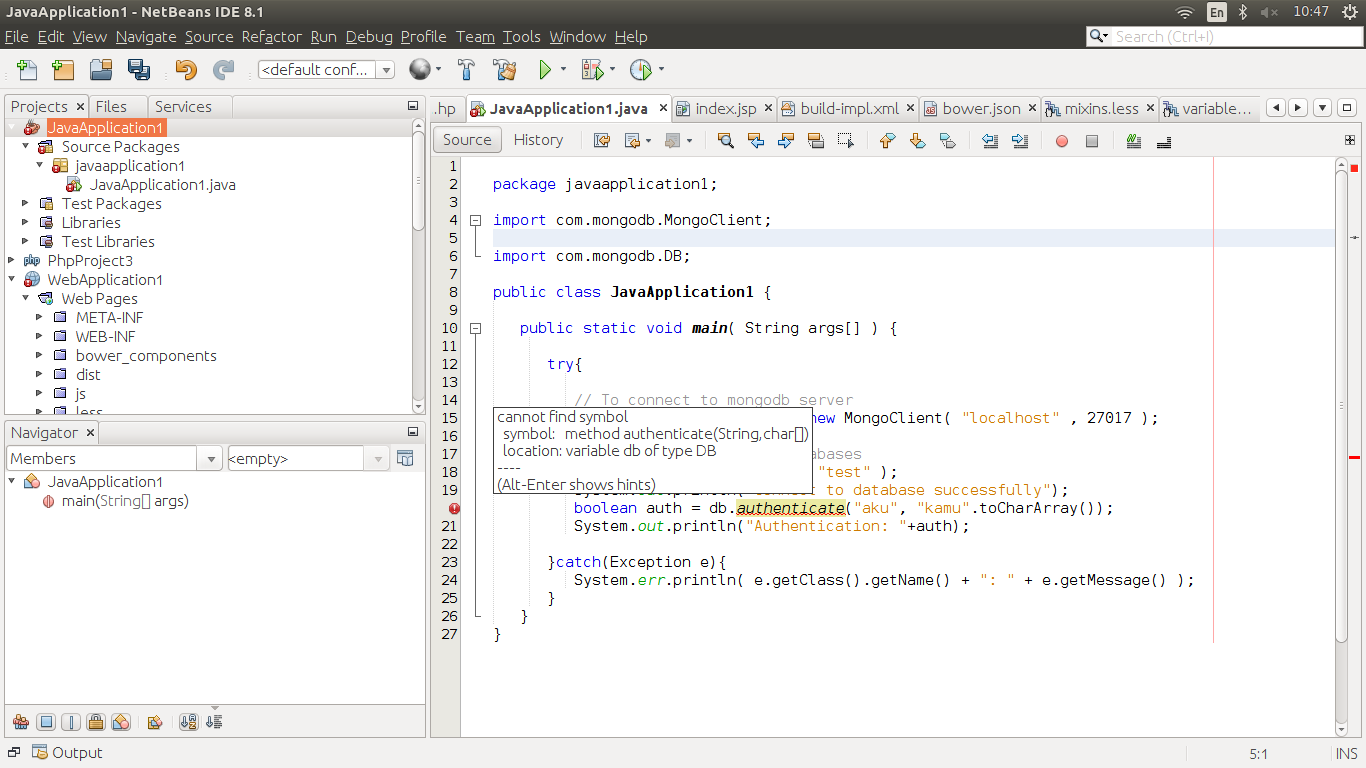
org.mongodb
mongo-java-driver
4.3.1
Gradle:
dependencies {
implementation 'org.mongodb:mongo-java-driver:4.3.1'
}
Step 2: Create a MongoClient Instance
To connect to your MongoDB database, you'll need to create a MongoClient
instance. This is done by passing the connection string to the MongoClient
constructor:
MongoClient mongoClient = new MongoClient("mongodb://localhost:27017/");
Step 3: Use the Query Methods
Now that you have a MongoClient
instance, you can use its query methods to retrieve data from your MongoDB database. For example, let's say we want to find all documents in a collection where the name
field is "John":
DB db = mongoClient.getDB("mydatabase");
DBCollection coll = db.getCollection("mycollection");
BasicDBObject query = new BasicDBObject();
query.put("name", "John");
DBCursor cursor = coll.find(query);
while (cursor.hasNext()) {
DBObject obj = cursor.next();
System.out.println(obj);
}
Step 4: Iterate Through the Results
Finally, we can iterate through the results of our query using a DBCursor
. This will allow us to process each document individually.
In this example, we're simply printing out each document to the console. But in your actual application code, you would replace this with whatever processing logic is necessary:
while (cursor.hasNext()) {
DBObject obj = cursor.next();
System.out.println(obj);
}
This is a basic outline of how to use MongoDB queries in Java. Of course, there are many other things you can do with MongoDB, such as creating indexes, using aggregations, and more.
How to get MongoDB data in Java?
I'd be happy to help with that!
Getting MongoDB data in Java can be a bit tricky, but don't worry, I've got you covered. Here's a step-by-step guide on how to do it.
Step 1: Add the MongoDB Driver Dependency
To connect to your MongoDB database from Java, you'll need to add the MongoDB driver dependency to your project. You can do this by adding the following Maven dependency:
org.mongodb
mongo-java-driver
3.12.7
Step 2: Create a MongoDB Client
Next, you'll need to create a MongoDB client object that connects to your database. You can do this using the following Java code:
import com.mongodb.MongoClient;
import com.mongodb.client.MongoClients;
MongoClient mongoClient = MongoClients.create("mongodb://localhost:27017");
In this example, we're connecting to a local MongoDB instance running on port 27017.
Step 3: Access Your Collection
Once you have your MongoClient object, you can use it to access your MongoDB collection. You can do this using the following Java code:
import com.mongodb.client.MongoCollection;
MongoCollection collection = mongoClient.getDatabase("mydatabase").getCollection("mymycollection");
In this example, we're accessing a collection called "mymycollection" in a database called "mydatabase".
Step 4: Query Your Data
Now that you have your collection object, you can use it to query your data. MongoDB uses a flexible querying system, so you can use the find()
method to retrieve documents from your collection.
For example, if you want to retrieve all documents in your collection where the field "name" is "John", you can do this:
import com.mongodb.client.FindIterable;
import org.bson.Document;
FindIterable results = collection.find(eq("name", "John"));
This code uses the find()
method to query your collection and returns a FindIterable
object containing all documents that match your query.
Step 5: Process Your Data
Finally, you can use a loop or an iterator to process each document in your results:
import org.bson.Document;
// Process each document in the result set
results.forEach((document) -> {
// Do something with your document
});
And that's it! That's how you get MongoDB data in Java. With these steps, you should be able to connect to your MongoDB database, access your collection, query your data, and process the results.
I hope this helps!