How to run main method in Java?
How to run main method in Java?
I'm glad you're excited about learning Java!
Running the main
method in a Java program is relatively straightforward. But before we dive into that, let me briefly introduce the concept of classes and methods.
In object-oriented programming (OOP), a class is essentially a blueprint or template for creating objects. A class defines the properties and behaviors of an object. In Java, every program starts with at least one class, which must contain a main
method that serves as the entry point for your program.
A method is a block of code that performs a specific task. Methods can take parameters (inputs), return values, and be reused throughout your program. The main
method is no exception; it's a special method in Java that's responsible for starting the execution of your program.
So, to run the main
method in a Java program, you need to create a class with a main
method and then execute that class using the java
command. Here are the steps:
MyJavaProgram.java
. Define the main
method: Inside this new file, define a class with a main
method that has the following signature:
public static void main(String[] args) {
// Your code here!
}
The public
, static
, and void
keywords are essential for making the main
method accessible from outside your class. The String[] args
parameter is an array of strings that represents the command-line arguments passed to your program.
main
method, write the code you want to execute when your program starts. This could be anything: printing a message to the console, reading input from the user, performing calculations, or interacting with external systems.
Here's an example MyJavaProgram.java
file that demonstrates this:
public class MyJavaProgram {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Compile and run your Java program: Once you've created your Java file, you'll need to compile it into bytecode that can be executed by the Java Virtual Machine (JVM). You can do this using an IDE's build tool or a command-line compiler like javac
:
javac MyJavaProgram.java
This will generate a new file called MyJavaProgram.class
.
Finally, to run your program, use the java
command and specify the name of your class (without the .class
extension):
java MyJavaProgram
This will execute your main
method, printing "Hello, World!" to the console.
That's it! With these steps, you should be able to create and run a Java program with a main
method. Remember that your main
method serves as the entry point for your program, so make sure it's properly defined and executed. Happy coding!
What is the function of main() in Java?
I'll respond in English for now!
The main()
method in Java is the entry point of a Java program. It's the first method that gets called when you run your Java program. In other words, it's the starting point of your Java application.
The main function is declared as public, static and void, which means it can be accessed from outside its class (public), it belongs to the class itself (static), and it doesn't return any value (void). The method signature looks like this:
public static void main(String[] args) {
// Your code goes here...
}
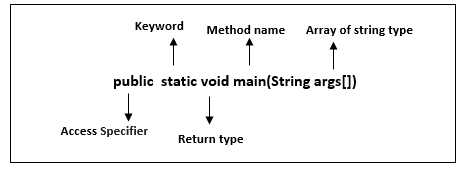
Here's what each part of the main()
method does:
main()
method to be accessed from outside its class.
main()
method without creating an instance of its class. In other words, you don't need an object of that class to call this method. void: This return type indicates that the main()
method doesn't return any value. Instead, it executes a series of statements and then terminates.
The main()
method is responsible for executing the program. It's where your Java application starts its execution. The arguments passed in the array args
represent command-line arguments provided to the program when it runs.
In most cases, you'll use the main()
method as the entry point of your Java program, and all other methods will be called from here. For example:
public static void main(String[] args) {
// Initialize variables...
for (int i = 0; i < 5; i++) {
System.out.println("Hello, World!");
}
}
In this simple example, the main()
method prints "Hello, World!" five times to the console. The program starts execution from here and terminates after all statements have been executed.
In summary, the main()
method is the starting point of a Java program, and it's responsible for executing the program. It's declared as public, static, and void, allowing you to access it from outside its class without creating an instance.