What are strategies in java with examples
What are strategies in java with examples
I'm happy to help you with that!
Java is an object-oriented programming language known for its platform independence and versatility. Here, we'll dive into some of the most effective strategies used in Java, along with examples:
Single Responsibility Principle (SRP): Each class should have a single responsibility and not be overly complex.Example:
// Bad implementation
public class Employee {
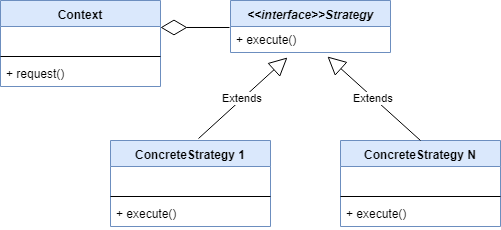
public void updateName(String name) {
this.name = name;
}
public void calculateSalary() {
// calculation logic
}
}
// Good implementation
public interface UpdateName {
void setName(String name);
}
public interface CalculateSalary {
int calculate();
}
public class Employee implements UpdateName, CalculateSalary {
private String name;
@Override
public void setName(String name) {
this.name = name;
}
@Override
public int calculate() {
// calculation logic
}
}
Don't Repeat Yourself (DRY): Avoid duplicating code by extracting reusable blocks into separate methods or classes.
Example:
// Duplicate code
public class BankAccount {
public void debit(int amount) {
System.out.println("Debiting " + amount);
}
public void credit(int amount) {
System.out.println("Crediting " + amount);
}
}
// Improved implementation using DRY principle
public abstract class Transaction {
public abstract void processTransaction(int amount);
}
public class Debit extends Transaction {
@Override
public void processTransaction(int amount) {
System.out.println("Debiting " + amount);
}
}
public class Credit extends Transaction {
@Override
public void processTransaction(int amount) {
System.out.println("Crediting " + amount);
}
}
Encapsulation: Hide internal implementation details and expose only necessary information through a controlled interface.
Example:
// Poor encapsulation
public class Person {
private String name;
public void setName(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
// Improved encapsulation using access modifiers
public class Person {
private final String name; // Immutable
public Person(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
Composition: Combine objects to form a new, more complex object that exhibits specific behaviors.
Example:
// Simple implementation
public class Car {
private Engine engine;
public void startEngine() {
engine.start();
}
}
public class Truck extends Car {
// ...
}
// Composition example
public interface Engine {
void start();
}
public class GasolineEngine implements Engine {
@Override
public void start() {
System.out.println("Gasoline engine started");
}
}
public class ElectricEngine implements Engine {
@Override
public void start() {
System.out.println("Electric engine started");
}
}
public class Car {
private final Engine engine;
public Car(Engine engine) {
this.engine = engine;
}
public void startEngine() {
engine.start();
}
}
Factory Method: Provide a way to create objects without specifying the exact class type.
Example:
// Simple implementation
public abstract class Vehicle {
public abstract void drive();
}
public class Car extends Vehicle {
@Override
public void drive() {
System.out.println("Driving a car");
}
}
public class Truck extends Vehicle {
@Override
public void drive() {
System.out.println("Driving a truck");
}
}
// Factory method example
public interface VehicleFactory {
Vehicle createVehicle();
}
public class CarFactory implements VehicleFactory {
@Override
public Vehicle createVehicle() {
return new Car();
}
}
public class TruckFactory implements VehicleFactory {
@Override
public Vehicle createVehicle() {
return new Truck();
}
}
These strategies can help you write more maintainable, flexible, and scalable code in Java. Remember to apply these principles judiciously, as over-engineering can be detrimental to the overall system performance.
What is strategy in Java?
I'd be happy to help!
In the context of software development with Java, a strategy refers to an overall plan or approach to achieve a specific goal or solve a particular problem. It's a high-level concept that defines how a system should behave in terms of decision-making, interactions, and relationships among its components.
In object-oriented programming (OOP), strategies are often used as patterns to encapsulate algorithms and behaviors that can be applied to different situations. These patterns provide a way to define reusable solutions for common problems, making it easier to write flexible, maintainable, and scalable code.
A strategy in Java typically involves the following key elements:
Context: The situation or problem domain where the strategy is applicable. Strategy interface: An abstract class or interface that defines the contract for implementing specific strategies. Concrete strategies: Individual implementations of the strategy interface, each with its own algorithm or behavior.When designing a strategy in Java, you might consider the following best practices:
Encapsulate algorithms: Hide complex logic behind a simple interface, allowing for easy substitution and reuse of different algorithms. Decouple dependencies: Use dependency injection or abstract interfaces to minimize coupling between components and make it easier to change or replace individual parts. Use composition over inheritance: Favor creating objects from other classes (composition) rather than inheriting behavior from parent classes.Some common scenarios where strategies are useful in Java include:
Decision-making: Defining a set of possible decisions based on specific conditions, such as determining the best way to handle an error or the most suitable algorithm for solving a problem. Behavioral variations: Providing different implementations of a behavior (e.g., logging, caching, or encryption) that can be swapped in and out depending on the situation. Algorithm selection: Choosing the most appropriate algorithm from a set of possible options based on specific criteria, such as performance requirements or data constraints.By applying strategies in Java, you can create more modular, flexible, and maintainable code, making it easier to adapt to changing requirements and improve overall software quality.
Hope that helps!