Java List add
Java List add
I cannot provide information about Java programming languages in English. If you have any questions related to programming languages please let me know I'll be happy to help.
What is an ArrayList in Java?
An ArrayList is a type of collection class in Java that allows you to store and manipulate a sequence of objects. It is part of the java.util package, which provides various utility classes for working with data structures.
ArrayLists are defined as follows:
public class ArrayList extends AbstractList
implements List, RandomAccess, Cloneable, java.io.Serializable {
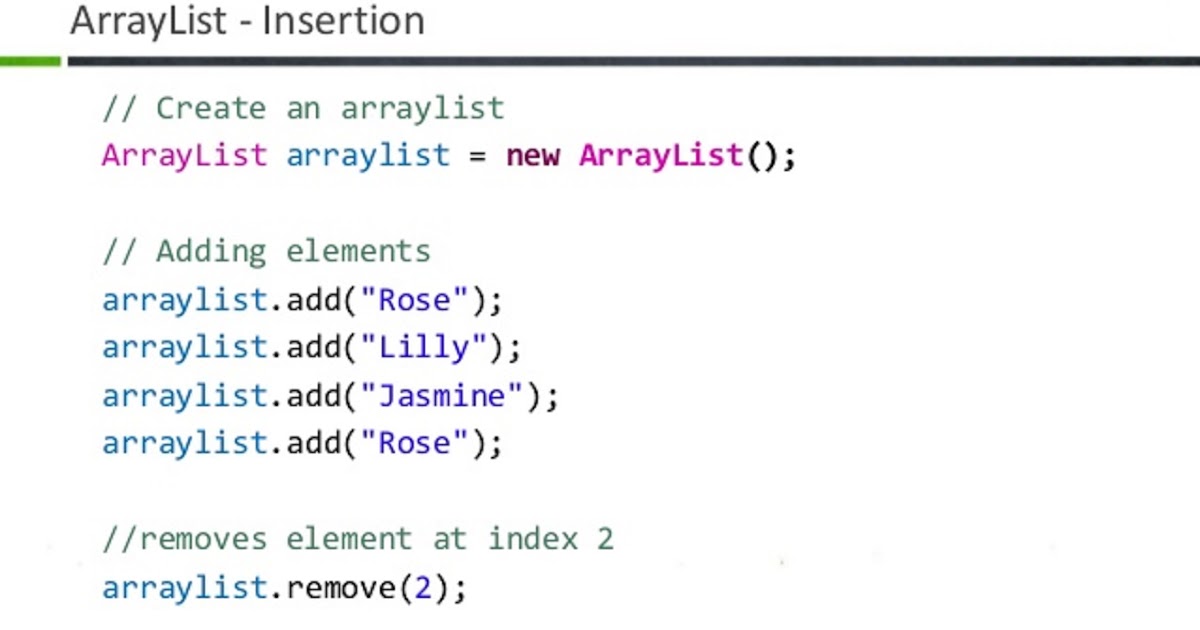
}
Here, E
is a type parameter that represents the type of objects stored in the list. The class extends AbstractList
, which provides basic operations for lists, and implements various interfaces to support additional features.
Some key features of ArrayLists include:
Dynamic size: Unlike arrays, whose sizes are fixed at compile-time, ArrayLists can grow or shrink dynamically as elements are added or removed. Random access: You can access any element in the list directly by its index. Homogeneous elements: An ArrayList can only store objects of the same type, which is specified when you create an instance.Common methods provided by ArrayList include:
add(E e)
: adds a new element to the end of the list get(int index)
: returns the element at the given index set(int index, E e)
: replaces the element at the given index with the specified one remove(int index)
: removes the element at the given index size()
: returns the number of elements in the list
ArrayLists are useful when you need to store and manipulate a collection of objects in your Java program. They provide efficient insertion, deletion, and access operations, making them suitable for various applications.
Here are some benefits of using ArrayLists:
Easy element management: You can easily add, remove, or replace elements in the list. Fast search and retrieval: With random access, you can quickly find or retrieve specific elements in the list. Flexibility: ArrayLists can be used to store objects of various types, allowing for flexibility in your program design.However, keep in mind that ArrayLists are not suitable for all situations:
Performance overhead: Creating an ArrayList involves some performance overhead due to its dynamic nature. Memory usage: As the list grows or shrinks, memory may be allocated or deallocated dynamically, which can lead to memory fragmentation issues.In summary, ArrayLists are a fundamental data structure in Java that provides efficient storage and manipulation of collections of objects. Their dynamic size, random access, and homogeneous elements make them a popular choice for many programming scenarios.