Java multithreading programming questions and answers
Java multithreading programming questions and answers
I'm happy to help with your request! Here are some common Java multithreading programming questions and their answers:
Q1: What is the difference between a Thread and a Runnable?
A: A Thread
is an object that represents a single thread of execution. It has its own run()
method, which gets called when the thread starts. On the other hand, a Runnable
is an interface that defines a single method, run()
. You can wrap any class implementing the Runnable
interface inside a Thread
to create a new thread.
Q2: Can you explain the concept of synchronization in Java multithreading?
A: In Java multithreading, synchronization refers to the process of coordinating access to shared resources among multiple threads. Synchronization is achieved using locks (such as synchronized
blocks or Lock
objects) and other mechanisms that ensure only one thread can modify a resource at any given time.
Q3: How do you create a new thread in Java?
A: You can create a new thread in Java by extending the Thread
class, implementing the Runnable
interface, or using the Thread
constructor with a Runnable
object. Here's an example of creating a thread using the Thread
constructor:
public class MyThread extends Thread {
public void run() {
System.out.println("Hello from thread!");
}
}
MyThread myThread = new MyThread();
myThread.start();
Q4: What is the purpose of the join()
method in Java multithreading?
A: The join()
method allows one thread to wait for another thread to finish. When a thread calls join()
on another thread, it will suspend its execution until the other thread finishes.
Q5: How do you handle exceptions in Java multithreading?
A: In Java multithreading, exceptions can occur when handling threads. You can use try-catch blocks or exception handlers to catch and handle exceptions within a thread. Alternatively, you can let the exception propagate up the call stack and be caught by a higher-level handler.
Q6: What is a Semaphore
in Java multithreading?
A: A Semaphore
in Java multithreading is a countable lock that allows only a certain number of threads to access a resource at any given time. Semaphores are useful for implementing resource-limited scenarios, such as limiting the number of users who can access a database.
Q7: How do you create a daemon thread in Java?
A: You can create a daemon thread in Java by calling the setDaemon()
method on a Thread
object. Daemon threads run indefinitely and do not prevent the JVM from exiting when they complete their tasks.
Q8: What is the purpose of the volatile
keyword in Java multithreading?
A: The volatile
keyword in Java multithreading ensures that changes to variables are always visible to all threads, even if a thread does not perform a write operation. This is useful when multiple threads access shared resources.
Q9: How do you detect whether a thread has completed its task in Java?
A: You can use the join()
method or check the isAlive()
or getState()
methods of a Thread
object to determine whether a thread has finished its execution.
Q10: What is the difference between synchronized
and ReentrantLock
in Java multithreading?
A: synchronized
is a built-in locking mechanism in Java that allows only one thread to execute a block of code at any given time. ReentrantLock
, on the other hand, is a more flexible and customizable locking mechanism that can be used to create complex synchronization scenarios.
I hope these questions and answers help you with your Java multithreading programming needs!
What is thread safe in Java JavaTpoint?
I'm glad you asked!
In Java, thread-safe refers to a piece of code that can be safely executed by multiple threads concurrently without causing any problems or unexpected behavior. This ensures that the code works correctly even when it's accessed by multiple threads simultaneously.
Java provides various mechanisms for creating thread-safe code:
Synchronized Methods: These methods are protected from being accessed by other threads while they're executing. Thesynchronized
keyword is used to declare these methods, which locks the entire object until the method finishes execution. This prevents any thread from accessing the method until the previous access has completed.
Example:
public class Counter {
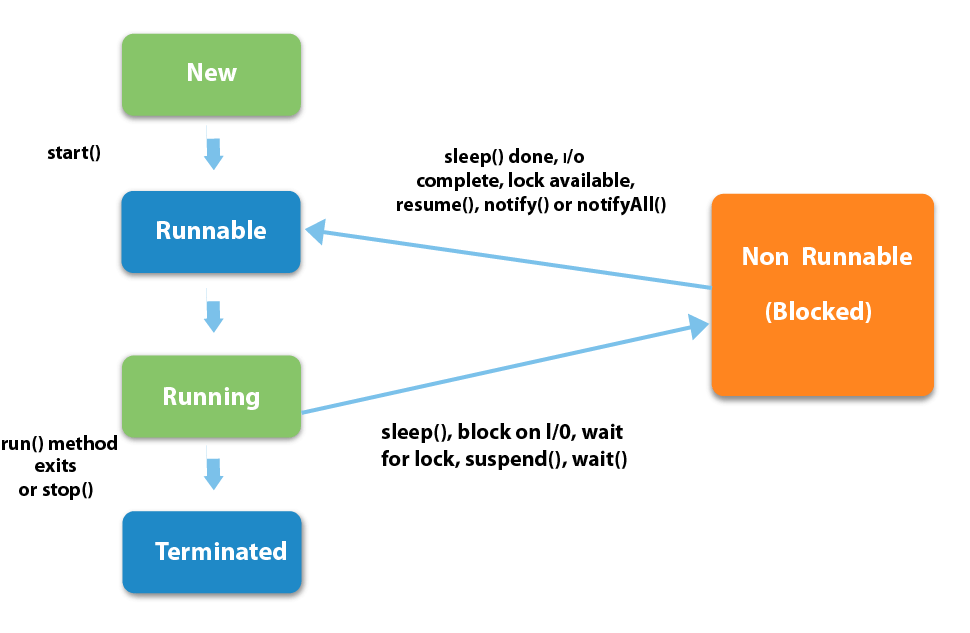
private int count = 0;
public synchronized void increment() {
count++;
}
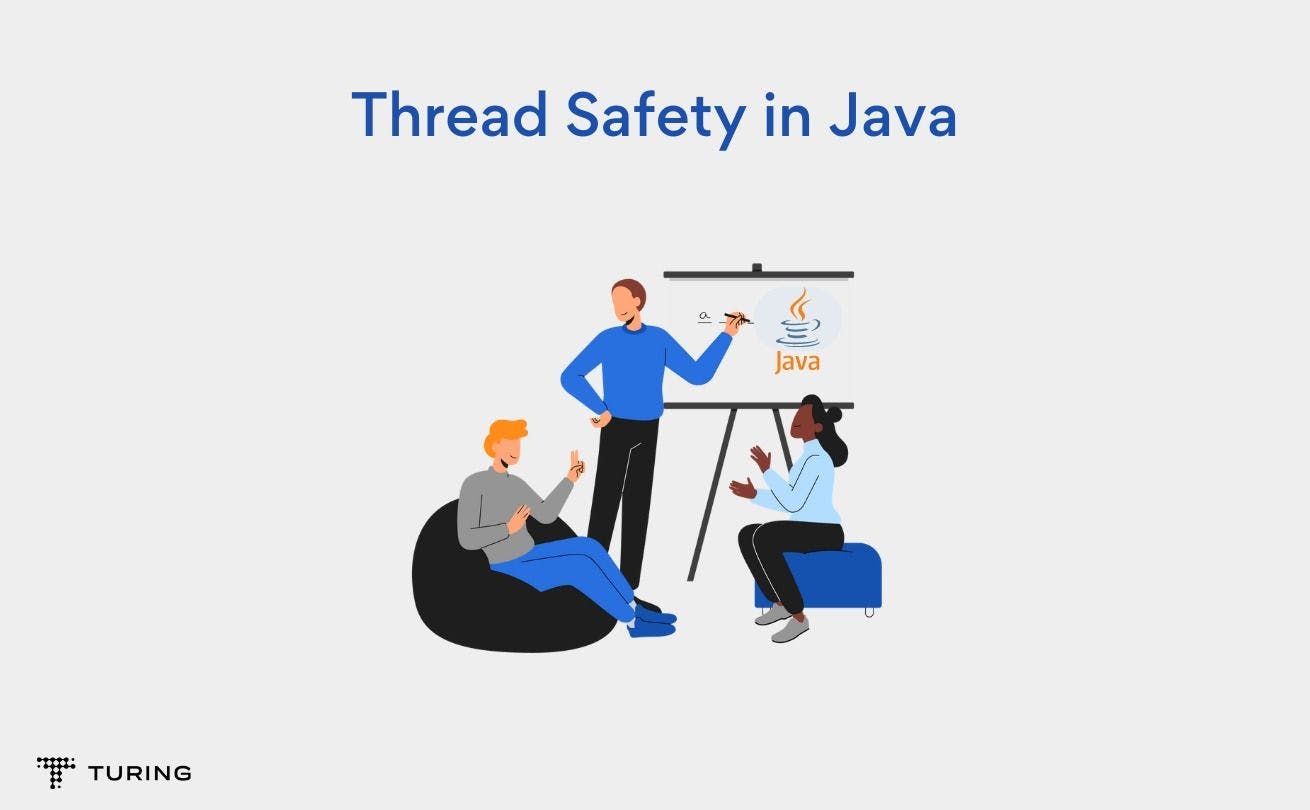
public int getCount() {
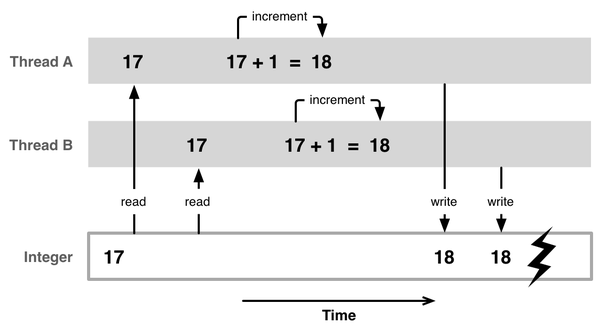
return count;
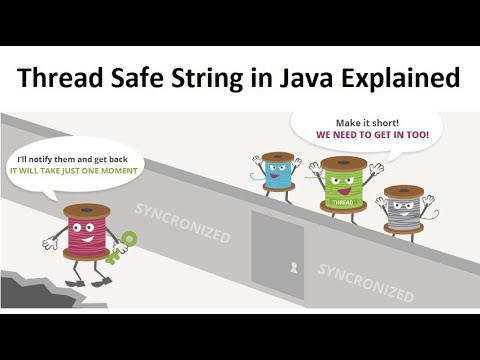
}
}
Locks: Java provides ReentrantLock
and ReentrantReadWriteLock
classes in the java.util.concurrent.locks
package to create custom locks for thread-safe code.
Example:
public class Counter {
private int count = 0;
private final ReentrantLock lock = new ReentrantLock();
public void increment() {
lock.lock();
try {
count++;
} finally {
lock.unlock();
}
}
public int getCount() {
lock.lock();
try {
return count;
} finally {
lock.unlock();
}
}
}
Atomic Variables: Java 5 and later introduced atomic variables, such as AtomicInteger
, which provide thread-safe operations for updating shared variables.
Example:
public class Counter {
private final AtomicInteger count = new AtomicInteger(0);
public void increment() {
count.incrementAndGet();
}
public int getCount() {
return count.get();
}
}
Immutable Objects: Making objects immutable by design ensures that their state is not changed externally, eliminating the need for thread-safe code.
Example:
public class Person {
private final String name;
private final int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
In summary, Java provides various mechanisms for creating thread-safe code, including synchronized methods, locks, atomic variables, and immutable objects. By understanding these concepts and using them appropriately, you can write robust, concurrent code that's suitable for multi-threaded applications.
Now, would you like me to explain the implications of Java 8's CompletableFuture
on thread safety?