What is a method example in java with arguments
What is a method example in java with arguments
I'd be happy to help you with that!
In Java, methods are blocks of code that can be reused multiple times in a program. They allow us to encapsulate a piece of functionality and reuse it throughout our application.
A method in Java is defined using the public
, private
, or protected
access modifier followed by the return type (which is optional), the method name, and finally, a list of parameters enclosed in parentheses.
Let's create an example of a simple method called greetUser()
that takes two string arguments: name
and greeting
. This method will print out a customized greeting message:
public class HelloWorld {
public static void greetUser(String name, String greeting) {
System.out.println(greeting + " " + name);
}
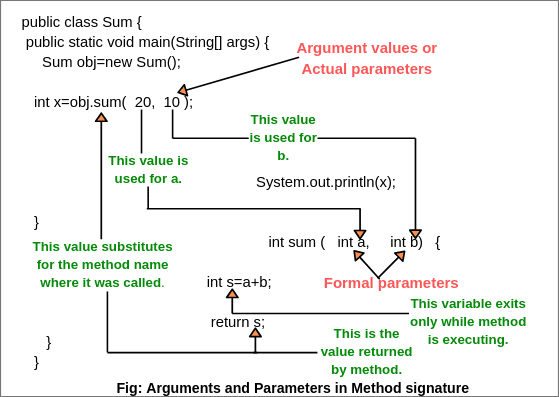
public static void main(String[] args) {
// Calling the greetUser() method with arguments
greetUser("John", "Hello");
}
}
In this example:
We define a classHelloWorld
that contains a greetUser()
method. The greetUser()
method takes two parameters: name
and greeting
. It prints out a customized greeting message using these arguments. In the main()
method, we call greetUser()
with the arguments "John"
and "Hello"
, passing these values into the method.
When you run this program, it will print:
Hello John
This demonstrates how methods in Java can take arguments and execute specific functionality based on those inputs. This is just a simple example; methods can be much more complex and handle various scenarios based on their parameters.
Here are some key points to remember about methods in Java:
Methods can have zero or more parameters. Parameters must match the method's parameter list exactly (number, type). Methods can return values using thereturn
statement. Methods can have a void return type if they don't return any value. You can use overloading to create multiple methods with the same name but different parameters. You can also use method overriding to customize behavior in subclasses.
Now that you've seen this example, I hope you have a solid understanding of how methods work in Java!
What is a method example in java w3schools
I'm happy to help!
A Method Example in Java:
Methods are blocks of code that can be executed repeatedly with different inputs without repeating the entire process. In Java, methods are declared inside classes and are used to perform specific tasks.
Let's consider an example of a simple method in Java that adds two numbers:
public class Main {
public static void main(String[] args) {
int sum = addNumbers(10, 20);
System.out.println("The sum is: " + sum);
}
public static int addNumbers(int num1, int num2) {
return (num1 + num2);
}
}
In this example:
We define a classMain
with a main method that serves as the entry point for our program. The main
method is where we call the addNumbers
method and store its result in the sum
variable. The addNumbers
method takes two integers (num1
and num2
) as input, adds them together using the return (num1 + num2);
statement, and returns the resulting sum.
Key Takeaways:
Methods can be reused with different inputs to achieve different results. Methods are defined inside classes, which provides organization and encapsulation for our code. The return statement is used to pass the result of a method back to its caller.Benefits of Using Methods in Java:
Reusability: As mentioned earlier, methods can be reused with different inputs to achieve different results, promoting code reuse and reducing duplication. Modularity: By breaking down our program into smaller, more manageable blocks of code (methods), we create a more modular and maintainable codebase. Readability: Methods help organize our code, making it easier for other developers to understand the purpose and functionality of each section.By using methods in Java, you can write more efficient, organized, and reusable code that is easy to read and maintain.
W3Schools (a popular online platform for learning web development) also provides a comprehensive guide on Methods in Java, including examples, syntax, and best practices.