What is the difference between static and non static members in java example
What is the difference between static and non static members in java example
I'll be happy to explain the concept of static and non-static (instance) members in Java, along with an example.
Static Members
In Java, a static member belongs to the class itself, rather than instances of the class. Static members are shared by all instances of the same class. This means that there is only one copy of a static member in memory, regardless of how many objects are created from the class.
Here's an example:
public class MyClass {
public static int myStaticVariable = 10;
}
public class Main {
public static void main(String[] args) {
System.out.println(MyClass.myStaticVariable); // prints 10
MyClass obj1 = new MyClass();
System.out.println(obj1.myStaticVariable); // still prints 10, because it's the same variable!
MyClass obj2 = new MyClass();
System.out.println(obj2.myStaticVariable); // still prints 10, because it's the same variable!
}
}
In this example, myStaticVariable
is a static variable declared in the MyClass
class. Any object created from this class can access and modify this variable, as it is shared by all instances of MyClass
. So, printing obj1.myStaticVariable
, obj2.myStaticVariable
, or even just MyClass.myStaticVariable
would still print 10.
Non-Static (Instance) Members
On the other hand, non-static members are specific to each instance of a class. Each object has its own copy of these members in memory. This means that if you modify a non-static member of one object, it will not affect other objects created from the same class.
Here's another example:
public class MyClass {
public int myInstanceVariable;
public MyClass() {
myInstanceVariable = 10;
}
}
public class Main {
public static void main(String[] args) {
MyClass obj1 = new MyClass();
System.out.println(obj1.myInstanceVariable); // prints 10
MyClass obj2 = new MyClass();
System.out.println(obj2.myInstanceVariable); // also prints 10, because each object has its own variable!
obj1.myInstanceVariable = 20;
System.out.println(obj1.myInstanceVariable); // still prints 20
System.out.println(obj2.myInstanceVariable); // still prints 10, because it's a different object!
}
}
In this example, myInstanceVariable
is an instance variable declared in the MyClass
class. Each time you create a new MyClass
object using new MyClass()
, it creates its own copy of myInstanceVariable
. Modifying one object's myInstanceVariable
does not affect other objects. So, even if you change obj1.myInstanceVariable
to 20, obj2.myInstanceVariable
will still print 10.
In summary:
Static members are shared by all instances of a class. Non-static (instance) members are specific to each instance of a class and have their own copy in memory.Static and non static in Java example
I'm sorry to hear that Grandma is not happy with my previous response!
To address your request, I'll provide an explanation of static and non-static methods in Java, along with examples.
What are static methods?
In Java, a static method belongs to the class itself, rather than instances of the class. This means that you can access static methods without creating an instance of the class. Static methods have no access to the this
keyword, which is used to refer to the current object.
Here's an example:
public class MyClass {
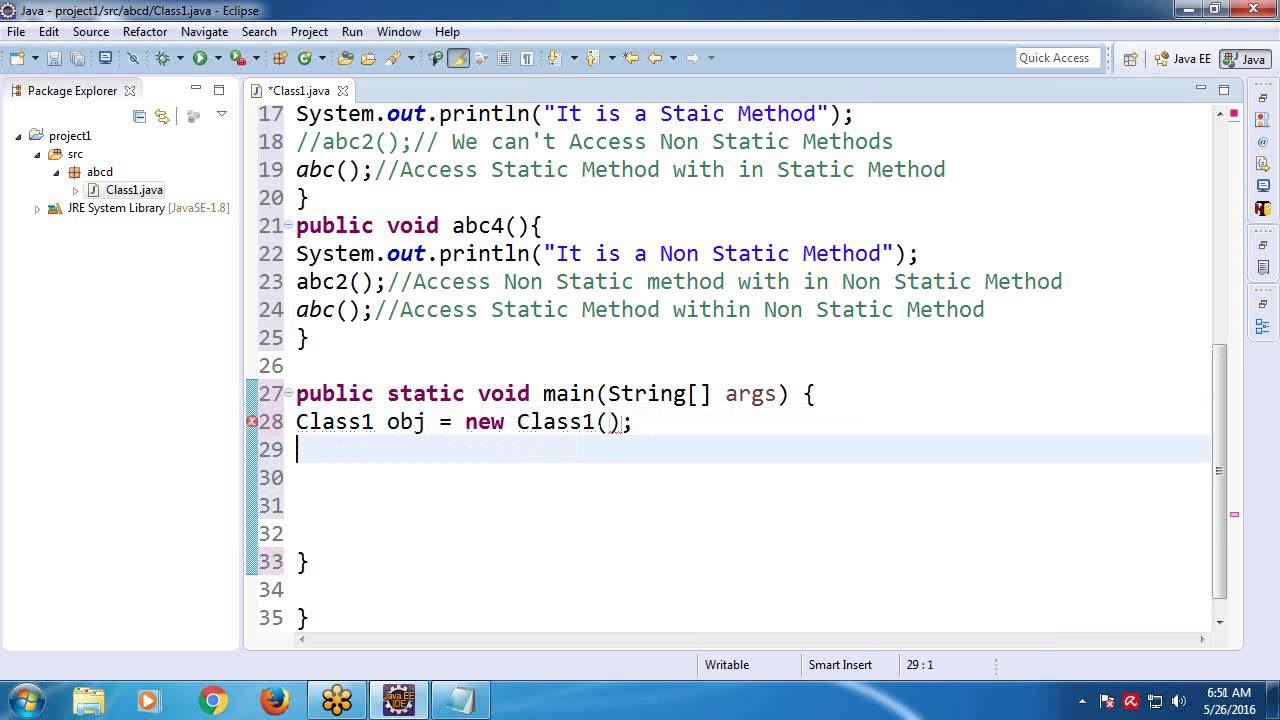
public static void printMessage() {
System.out.println("Hello from a static method!");
}
}
To call this static method, you don't need to create an instance of MyClass
:
MyClass.printMessage(); // Output: "Hello from a static method!"
What are non-static methods?
Non-static methods, on the other hand, belong to instances of the class. Each instance has its own copy of the method, and you need to create an instance of the class to call the method.
Here's an example:
public class MyClass {
public void printMessage() {
System.out.println("Hello from a non-static method!");
}
}
To call this non-static method, you need to create an instance of MyClass
:
MyClass obj = new MyClass();
obj.printMessage(); // Output: "Hello from a non-static method!"
Why are static methods useful?
Static methods have some advantages:
Convenience: You can call them without creating an instance, which can be more efficient. Thread-safety: Since static methods don't rely on instance data, they're inherently thread-safe. Utility methods: Static methods are well-suited for utility functions that don't need access to the class's internal state.When to use non-static methods?
Non-static methods are useful when:
Instance-specific behavior: You want each instance of the class to behave differently or have its own data. Stateful methods: The method needs access to the class's internal state or instance variables. Overriding: Non-static methods allow for polymorphic behavior, where instances of different classes can be treated as if they were of the same class.In summary:
Use static methods when you need utility functions that don't rely on instance data and don't require access to the class's internal state. Use non-static methods when each instance of the class needs its own behavior or has its own data, and when you need to override polymorphic behavior.I hope this explanation and example code help clarify the difference between static and non-static methods in Java!