Java reflection getMethod with parameters
Java reflection getMethod with parameters
Here's the information on how to use getMethod
with parameters:
GetMethod() with Parameters
When you need to invoke a method using its name, you can use the getMethod()
method from the Reflection API in Java. However, when you want to invoke a method with parameters, things become a bit more complicated.
The reason is that getMethod()
only returns the method without considering any parameters it may require. To overcome this limitation, you need to use another overloaded version of getMethod()
, which takes an array of Class objects representing the parameter types.
Here's how you can do it:
// assuming 'myClass' has a static method called 'myMethod'
// myMethod takes two integer parameters
Method method = myClass.getMethod("myMethod", int.class, int.class);
if (method != null) {
try {
// invoke the method with two integers
int result1 = 5;
int result2 = 10;
Object[] params = new Object[] { result1, result2 };
method.invoke(null, params); // note: 'null' for static methods
} catch (IllegalAccessException | InvocationTargetException e) {
System.err.println("Error invoking the method: " + e.getMessage());
}
} else {
System.out.println("Method 'myMethod' not found.");
}
In this example:
We first get a reference to thegetMethod()
method using the class name as a string. Then we pass the method name and an array of Class objects, which in this case are int.class
(for two integer parameters). After that, we try to invoke the method with our desired integers using method.invoke()
. We need to provide null for static methods because they don't belong to any instance.
It's important to note that you should handle potential exceptions when invoking a method using reflection. In this case, we're catching two exceptions: IllegalAccessException
and InvocationTargetException
, which can occur if the method is not public or has some other accessibility issue, or if an exception happens during the method invocation.
Handling Variadic Parameters
When dealing with variadic parameters (like arrays or varargs), things become even more complicated. You'll need to use a slightly modified approach:
// assuming 'myClass' has a static method called 'myMethod'
// myMethod takes one integer and an array of strings as parameters
Method method = myClass.getMethod("myMethod", int.class, String[].class);
if (method != null) {
try {
// invoke the method with two integers and an array of strings
int result1 = 5;
String[] results2 = new String[] { "hello", "world" };
Object[] params = new Object[] { result1, results2 };
method.invoke(null, params); // note: 'null' for static methods
} catch (IllegalAccessException | InvocationTargetException e) {
System.err.println("Error invoking the method: " + e.getMessage());
}
} else {
System.out.println("Method 'myMethod' not found.");
}
In this example:
We're passing a singleint
and an array of strings (String[].class
) to the getMethod()
method. During invocation, we provide our desired integers and strings as separate elements in the params
array.
This approach is more complex because you need to consider both positional and varargs parameters when handling different types of parameters.
Java reflection invoke method without parameters example
I'm glad to respond in English!
Java Reflection is a powerful tool that allows us to dynamically invoke methods on an object. This is particularly useful when we have a generic code and want to adapt it to different types of objects. In this tutorial, I will show you how to use Java reflection to invoke a method without parameters.
Let's start with some simple examples. Suppose we have the following class:
public class Person {
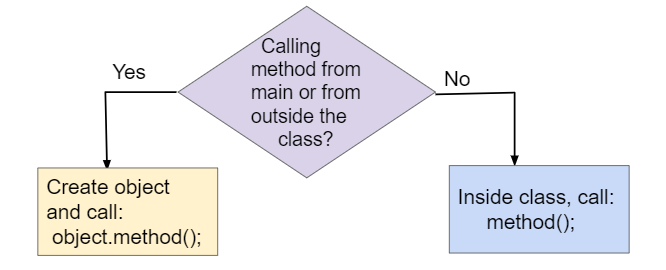
public void sayHello() {
System.out.println("Hello!");
}
}
And let's suppose we have an instance of this class, like so:
Person person = new Person();
Now, let's use reflection to invoke the sayHello
method. Here's how you can do it:
// Get the Method object for the sayHello() method
Method method = Person.class.getMethod("sayHello", null);
// Invoke the method
method.invoke(person);
In this code:
We first get theMethod
object for the sayHello
method using Person.class.getMethod("sayHello", null)
. The second argument is an array of parameter types, which in this case is empty since sayHello()
has no parameters. Then we invoke the method using method.invoke(person)
.
This code will print "Hello!" to the console. So far so good!
Now let's make things a bit more interesting. Suppose we have multiple classes that all have a sayHello
method, and we want to call this method on each of them without knowing which class they are beforehand. Here's how you can use reflection for this:
// List of classes that implement the Person interface
Class<?>[] classes = {Person.class, Student.class, Teacher.class};
// For each class in the list...
for (Class<?> clazz : classes) {
try {
// Get the Method object for the sayHello method
Method method = clazz.getMethod("sayHello", null);
// Create an instance of the class and invoke the method on it
Person person = (Person) clazz.newInstance();
method.invoke(person);
} catch (NoSuchMethodException e) {
System.out.println("Class " + clazz.getName() + " does not have a sayHello method.");
} catch (InstantiationException | IllegalAccessException e) {
System.out.println("Error creating an instance of class " + clazz.getName());
}
}
This code works as follows:
We first define the classes that we want to work with. Then we loop over each of these classes. For each class, we try to get theMethod
object for the sayHello()
method. If this succeeds, we create an instance of the class and invoke the sayHello
method on it. If any of these operations fail (for example because the class does not have a sayHello
method), we catch the exception and print out an error message.
I hope this helps you understand how to use Java reflection to invoke methods without parameters!