What is annotation class in Java?
What is annotation class in Java?
I'd be happy to explain what an annotation class is in Java!
In Java, an annotation is a special kind of declaration that can be attached to a class, method, variable, or other construct. Annotations are used to provide additional information about the annotated element without affecting how it works at runtime. In other words, annotations do not change the behavior of your code, but rather provide metadata (data that describes data) about the code.
An annotation class is essentially a Java class that defines custom annotations. Think of an annotation as a label or a tag that you can attach to specific parts of your code, and think of an annotation class as the blueprint for creating those labels.
To create an annotation class in Java, you need to define a class that extends the java.lang.Annotation
interface. The Annotation
interface has two abstract methods: public boolean equals(Object obj)
and public int hashCode()
.
Here is a simple example of an annotation class:
@Target(ElementType.METHOD)
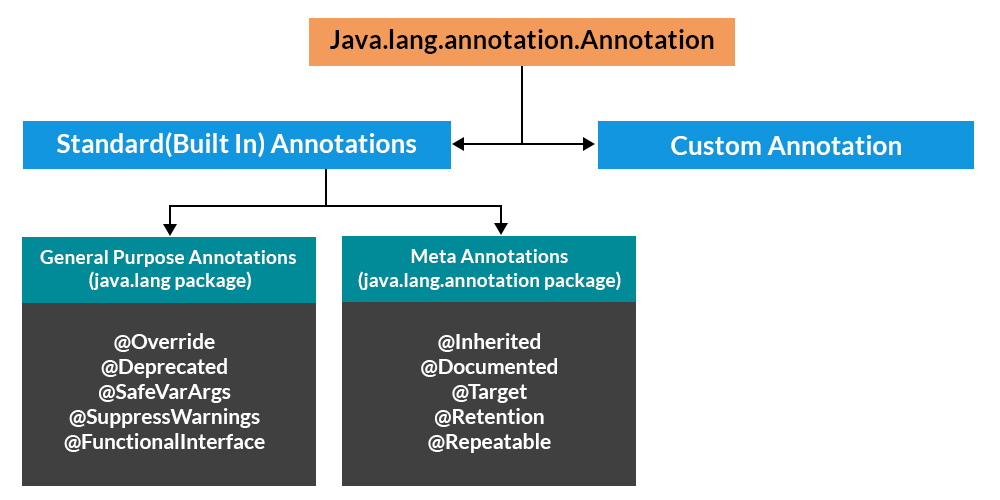
@Retention(RetentionPolicy.RUNTIME)
public @interface MyAnnotation {
String description();
}
In this example, MyAnnotation
is the name of the annotation class. The @Target
and @Retention
annotations specify where (or on what) the MyAnnotation
can be used and how long its effects last. In this case, it can only be used on methods (@Target(ElementType.METHOD)
), and its effects will persist until the program ends (@Retention(RetentionPolicy.RUNTIME)
).
The description()
method is a placeholder for any additional information you might want to provide with your annotation.
Now that we have an annotation class defined, let's attach it to a method:
public class MyClass {
@MyAnnotation(description = "This is my method")
public void myMethod() {
// Method implementation...
}
}
As you can see, the myMethod()
method has been annotated with our custom MyAnnotation
. The description
value we provided will be stored along with the annotation.
Annotations can be retrieved at runtime using reflection. Here's an example:
public class Main {
public static void main(String[] args) {
MyClass myClass = new MyClass();
Method method = myClass.getClass().getMethod("myMethod");
if (method.isAnnotationPresent(MyAnnotation.class)) {
MyAnnotation annotation = method.getAnnotation(MyAnnotation.class);
String description = annotation.description();
System.out.println(description); // Output: This is my method
}
}
}
In this example, we use reflection to get the myMethod()
method and then check if it has our custom MyAnnotation
attached. If it does, we retrieve its description
value and print it.
Annotations are a powerful tool in Java that can help you add meaning to your code without affecting how it runs. They have many uses, such as:
Code analysis: Annotations can be used by tools like IDEs or build scripts to analyze the structure of your code. Dependency injection: Annotations can be used to specify dependencies between components in a system. Data processing: Annotations can be used to provide additional information about data that needs to be processed.In this brief overview, we have seen what an annotation class is in Java and how it can be used to create custom annotations. We have also looked at how these annotations can be retrieved at runtime using reflection.
What is the annotation for POJO class in Java?
I'd be happy to explain what an annotation is and its usage on a POJO (Plain Old Java Object) class in Java.
In Java, an annotation is a form of metadata that can be applied to various elements such as classes, methods, fields, parameters, and even individual lines of code. Annotations are used to provide additional information about a particular piece of code, which can then be used by other parts of the program or even by external tools and frameworks.
An annotation consists of at least three parts:
The @ symbol: This is the standard prefix for all annotations in Java. The name of the annotation: This is the name given to the annotation, such as "@Override" or "@Deprecated". Optional attributes: These are additional values that can be associated with the annotation.For example, consider the following code:
public class MyPojo {
@Autowired
private MyService myService;
}
In this example, the @Autowired
annotation is applied to the myService
field. This annotation indicates that an instance of the MyService
class should be automatically injected into the myService
field when the MyPojo
object is instantiated.
Annotations can be used for a variety of purposes, including:
Providing metadata about a piece of code: For example, an annotation might indicate that a particular method is not intended to be called directly. Configuring frameworks and libraries: Annotations are often used by frameworks like Spring or Hibernate to configure how objects are created, injected, or managed. Generating code: Annotations can be used as input for code generation tools or IDE plugins to generate boilerplate code.Annotations are also used extensively in Java-based frameworks such as Spring, Hibernate, and Vaadin. For example:
In Spring, annotations like@Controller
, @Service
, and @Repository
are used to identify specific classes as controllers, services, or repositories. In Hibernate, annotations like @Entity
, @Table
, and @Column
are used to configure how JPA entities are mapped to database tables and columns.
In the context of a POJO class, an annotation can be used to provide additional information about the class itself. For example:
public class MyPojo {
@NoArgsConstructor
private MyService myService;
}
Here, the @NoArgsConstructor
annotation indicates that a no-argument constructor should be generated for the MyPojo
class.
In summary, annotations are a powerful feature in Java that can provide additional metadata about code, configure frameworks and libraries, or generate boilerplate code. In the context of a POJO class, an annotation can be used to provide information about the class itself, such as its constructor or injection requirements.