How to post http in java example
How to post http in java example
I'd be happy to help you with that!
In Java, you can use the URL
class and its related classes to work with HTTP requests and responses. Here's an example of how to post a simple request using HTTP:
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class HttpPostExample {
public static void main(String[] args) throws Exception {
// URL to POST data to
String url = "http://example.com/endpoint";
// Create a URL object
URL obj = new URL(url);
// Open a connection to that URL
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
// Set the request method as POST
con.setRequestMethod("POST");
// Send post request
con.setDoOutput(true);
java.io.OutputStream os = con.getOutputStream();
String data = "param1=value1¶m2=value2";
byte[] bytes = data.getBytes();
os.write(bytes, 0, bytes.length);
os.close();
// Get the response code
int responseCode = con.getResponseCode();
if (responseCode == 200) {
BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer content = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
content.append(inputLine);
}
in.close();
System.out.println(content.toString());
} else {
System.out.println("Failed to send request. Response code: " + responseCode);
}
}
}
In this example:
We create aURL
object for the endpoint we want to post data to. We open a connection to that URL using HttpURLConnection
. We set the request method to POST and enable output stream to send data. We prepare the data as a string and encode it into bytes, then write those bytes to the output stream. We get the response code from the server. If the response code is 200 (OK), we read the response body using a BufferedReader
and print it.
This example demonstrates how to send a simple POST request with some key-value pairs as data. You can modify this to suit your specific use case.
Please note that this is a basic example and you should consider handling errors, exceptions, and possibly adding authentication or other security measures depending on the specifics of your application.
Now, go ahead and try it out!
How to post http in java using for loop
Here's an example of how you can post HTTP requests in Java using a For Loop:
Let's assume you want to send multiple GET requests to a URL with different parameters using Java. Here is an example code snippet that does exactly that using Apache HttpClient:
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
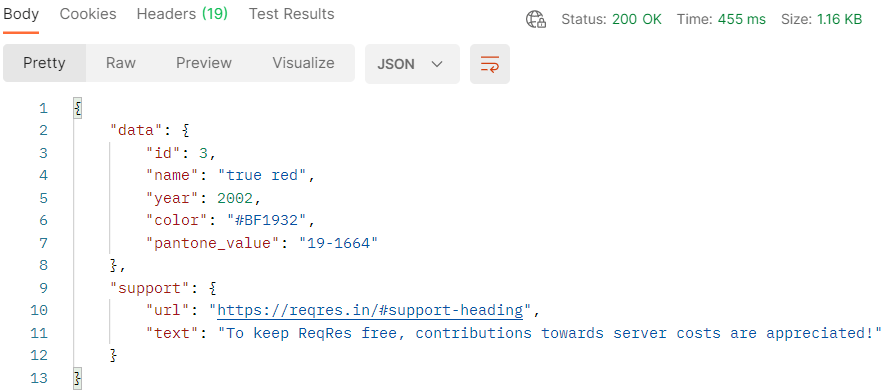
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.HttpClients;
public class HttpExample {
public static void main(String[] args) throws Exception {
// Create an HTTP client
HttpClient httpClient = HttpClients.createDefault();
// Create a list to store the URLs we need to send requests to
String[] urlsToVisit = new String[]{"https://www.example.com/param1=value1", "https://www.example.com/param2=value2", "https://www.example.com/param3=value3"};
// Loop over each URL and send a GET request to it
for (String url : urlsToVisit) {
// Create an HTTP get request
HttpGet httpGet = new HttpGet(url);
// Send the request and get the response
HttpResponse httpResponse = httpClient.execute(httpGet);
HttpEntity entity = httpResponse.getEntity();
// Read the contents of the response
String responseBody = org.apache.http.util.EntityUtils.toString(entity);
// Do something with the response data (e.g., print it)
System.out.println("Response for " + url + ":");
System.out.println(responseBody);
}
// Close the HTTP client when we're done
httpClient.close();
}
}
In this example, you can see that a For Loop is used to send multiple GET requests. Each request is sent using an HttpGet object.
Note: Make sure you have Apache HttpClient added as a dependency in your Java project before running this code.
Also, keep in mind that the above example uses Apache's HttpClient which is widely used and well maintained library. But if you need a more lightweight option then you might want to consider using OkHttp library or even the built-in HttpUrlConnection class available in java.
In any case, it's always good practice to handle exceptions properly when sending HTTP requests.