Why do we use an interface in Java?
Why do we use an interface in Java?
Here's the answer:
In Java, an interface is a abstract class that contains only constants (public static final fields) and abstract methods. Interfaces are used to define a contract or a blueprint for classes that implement them. This means that any class that implements an interface must provide an implementation for all of the methods in the interface.
Interfaces are often used in Java programming to achieve abstraction, encapsulation, and polymorphism. Here's how they help with these concepts:
Abstraction: An interface can be seen as a blueprint or a template that defines a set of methods without providing any actual implementation. This makes it possible for classes to inherit the methods from the interface without having to know the details of the implementation.Here are some reasons why we use an interface in Java:
To define a contract or blueprint for classes: Interfaces provide a way to define a set of methods without actually implementing them. This makes it possible for multiple classes to implement the same interface, and the system can still interact with those classes using the methods defined by the interface.In summary, using an interface in Java provides benefits such as abstraction, encapsulation, and polymorphism. Interfaces also enable multiple inheritance, allowing classes to inherit behavior from multiple interfaces without the need for explicit implementation.
Would you like me to expand on this topic or explore related concepts?
Use of interface in java w3schools
I'm happy to help!
W3Schools is a popular online platform that provides tutorials and reference materials on web development, including Java. According to W3Schools, Java is a high-level programming language developed by Sun Microsystems (now owned by Oracle Corporation). Here's an overview of how you can use the interface in Java with W3Schools:
Getting Started
To start working with W3Schools and Java, you'll need a few things:
A text editor or Integrated Development Environment (IDE) like Eclipse or NetBeans A basic understanding of HTML, CSS, and JavaScript (if you're new to web development, I recommend starting with some beginner-friendly tutorials)Creating a W3Schools Interface using Java
W3Schools provides an API for interacting with their platform programmatically. You can use Java to create a W3Schools interface by sending HTTP requests to their API endpoints.
Here's a simple example of how you could do this:
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
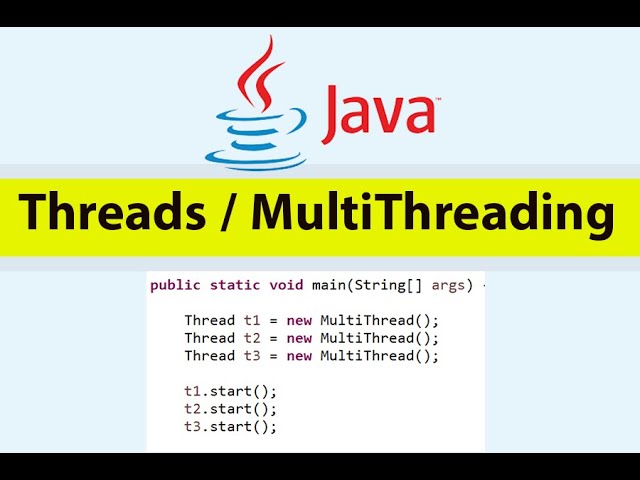
import java.net.HttpURLConnection;
import java.net.URL;
public class Main {
public static void main(String[] args) throws IOException {
// Set your W3Schools API token here:
String apiKey = "YOUR_API_KEY";
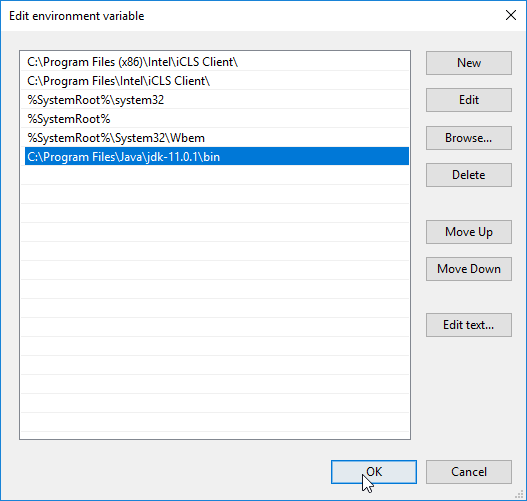
// Set the endpoint URL and query parameters
String url = "https://www.w3schools.com/api/convert.asp";
URL obj = new URL(url);
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
// Add request headers
con.setRequestMethod("POST");
con.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
// Set the query parameters as a POST request body
String query = "input=10&to=Spanish";
con.setDoOutput(true);
java.io.DataOutputStream wr = new java.io.DataOutputStream(con.getOutputStream());
wr.writeBytes(query);
wr.flush();
wr.close();
// Get the response
int status = con.getResponseCode();
if (status == 200) {
BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
con.disconnect();
// Process the response here
System.out.println(response.toString());
} else {
System.out.println("Failed to retrieve data");
}
}
}
This example uses Java's built-in HttpURLConnection
class to send a POST request to W3Schools' API endpoint, convert an integer to Spanish, and print the response.
Tips and Variations
Always check the W3Schools API documentation for the most up-to-date information on available endpoints and query parameters. You can modify the above code to suit your needs, such as changing the request method (e.g., GET, PUT, or DELETE), adding more query parameters, or processing the response differently.Remember that this is just a basic example, and you'll likely need to customize it to fit your specific requirements. W3Schools' API is designed for web development, so it's essential to understand how HTTP requests work in Java (or any other programming language) before attempting more complex interactions.
That's all for now! If you have further questions or would like help with something specific, feel free to ask.