What are 5 exceptions of java with examples
What are 5 exceptions of java with examples
I'd be happy to provide you with the list of exceptions in Java and some examples!
Java provides many built-in classes for throwing and catching exceptions, which is used to handle runtime errors or exceptional conditions during program execution.
Here are five key exceptions in Java along with their descriptions:
Example:
public class DivideByZero {
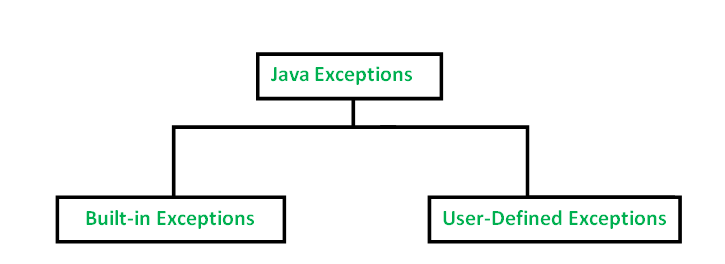
public static void main(String[] args) {
try {
int i = 10 / 0;
System.out.println("i is " + i);
} catch (ArithmeticException e) {
System.out.println("Caught ArithmeticException: " + e.getMessage());
}
}
}
ClassCastException: This exception is thrown when an attempt is made to cast an object to a subclass of which it is not an instance.
Example:
public class ClassCastExceptionDemo {
public static void main(String[] args) {
try {
Object obj = new Integer(10);
String str = (String) obj;
System.out.println(str);
} catch (ClassCastException e) {
System.out.println("Caught ClassCastException: " + e.getMessage());
}
}
}
IndexOutOfBoundsException: This exception is thrown when an index is out of range for the object being accessed.
Example:
public class IndexOutOfBoundsExceptionDemo {
public static void main(String[] args) {
try {
int arr[] = new int[5];
System.out.println(arr[5]);
} catch (IndexOutOfBoundsException e) {
System.out.println("Caught IndexOutOfBoundsException: " + e.getMessage());
}
}
}
IOException: This exception is thrown when there's an input/output error, such as when a file cannot be read or written.
Example:
public class IOExceptionDemo {
public static void main(String[] args) {
try {
FileReader reader = new FileReader("non-existent-file.txt");
} catch (IOException e) {
System.out.println("Caught IOException: " + e.getMessage());
}
}
}
NullPointerException: This exception is thrown when a null reference is dereferenced or used.
Example:
public class NullPointerExceptionDemo {
public static void main(String[] args) {
try {
String str = null;
System.out.println(str.length());
} catch (NullPointerException e) {
System.out.println("Caught NullPointerException: " + e.getMessage());
}
}
}
These are just a few of the many built-in exceptions in Java, and it's essential to understand how and when to use them correctly to ensure robust error handling in your programs.
Please note that I've only provided examples for these five exceptions; there are numerous other types of exceptions available in Java.
What are the exceptions in Java?
In Java, an exception is a runtime error that occurs when a program attempts to perform an operation that cannot be performed, such as dividing by zero or accessing an array out of bounds.
Exceptions can be handled using try-catch blocks, which allow the programmer to catch and handle exceptions in a controlled manner. Here are some types of exceptions:
Checked Exception: Checked exceptions are errors that can be detected at compile time. When a method declares that it may throw a checked exception, all subclasses of that exception must also be declared by any methods that override or overload the original method. If an exception is not caught in a try-catch block, the program will terminate. Examples of checked exceptions includeIOException
and SQLException
. Unchecked Exception: Unchecked exceptions are errors that can only be detected at runtime. These types of exceptions do not require explicit declaration by the compiler. Any method can throw an unchecked exception without declaring it. If an unchecked exception is thrown and not caught, the program will terminate. Examples of unchecked exceptions include ArrayIndexOutOfBoundsException
and ClassCastException
. Error: An error is a serious problem that a reasonable application should not try to catch. Errors are propagated up the call stack until they are either handled or reach the top-level program frame, at which point the VM terminates. RuntimeException: A subclass of the Exception
class that can be thrown during runtime. Examples of runtime exceptions include NullPointerException
, ArithmeticException
, and ArrayIndexOutOfBoundsException
.
checkRead()
) to indicate that a requested action is not allowed for some reason.
In Java, exceptions are handled using try-catch blocks, where:
try block: The code that may throw an exception. catch block: The code that catches and handles the exception.Here's an example of how to use a try-catch block in Java:
public void divide(int numerator, int denominator) {
try {
if (denominator == 0) {
throw new ArithmeticException("Cannot divide by zero");
}
int result = numerator / denominator;
System.out.println("The result is: " + result);
} catch (ArithmeticException e) {
System.out.println("Error: " + e.getMessage());
}
}
In this example, the divide
method attempts to divide two numbers. If the division results in a remainder because the denominator is zero, an ArithmeticException
is thrown and caught by the catch block.