What does @inject in java do reddit
What does @inject in java do reddit
I'm glad you asked!
@Inject
is a Java annotation that's part of the Google Guice dependency injection framework. In simple terms, it helps manage dependencies between objects or classes.
In traditional Java programming, you'd typically create instances of classes and manually pass dependencies to them. This can lead to tightly coupled code, making your program harder to maintain and extend.
Dependency Injection (DI) is a design pattern that decouples classes by providing their dependencies. Think of it like ordering food at a restaurant: you don't make the pizza yourself; instead, you rely on someone else to deliver it. In this case, "someone else" means other classes that provide the required dependencies.
When you use @Inject
, Guice (the DI framework) takes care of creating instances and injecting them into your class. You don't need to manually instantiate or configure the dependencies.
Here's an example:
Suppose you have a Logger
interface and a specific implementation, ConsoleLogger
. Your main application class (MainApp
) depends on a logger:
public class MainApp {
private Logger logger;
public MainApp(Logger logger) {
this.logger = logger;
}
public void doSomething() {
logger.info("Doing something...");
}
}
Without @Inject
, you'd need to manually create the logger and pass it to your main application class:
public class Main {
public static void main(String[] args) {
Logger logger = new ConsoleLogger();
MainApp mainApp = new MainApp(logger);
mainApp.doSomething();
}
}
This approach leads to tightly coupled code, making it harder to test and maintain.
Using @Inject
, you can decouple your classes:
public class MainApp {
private Logger logger;
@Inject
public MainApp(Logger logger) {
this.logger = logger;
}
public void doSomething() {
logger.info("Doing something...");
}
}
In the Main
class, you don't need to manually create the logger or pass it to your main application class:
public class Main {
@Inject
public static void main(String[] args) {
MainApp mainApp = Guice.createInjector().getInstance(MainApp.class);
mainApp.doSomething();
}
}
Guice takes care of creating the instances and injecting them into your classes. This makes your code more modular, reusable, and easier to test.
In summary, @Inject
is a Java annotation that helps manage dependencies between objects or classes using Guice's Dependency Injection framework. It decouples your code, making it more maintainable and testable.
What does @inject in java do with example
I'm happy to help you with that!
In Java, @Inject
is an annotation used to inject dependencies into a class or method using the Dagger dependency injection framework.
Dagger is a popular library for building loosely coupled systems by managing dependencies between objects. It's widely used in Android development and other Java-based projects.
When you add the @Inject
annotation to a variable, field, constructor parameter, or setter method, it indicates that the value should be injected into that location from a dependency graph defined elsewhere in your code.
Here's an example of using @Inject
with a simple class:
// Define a component (e.g., Application) that provides dependencies
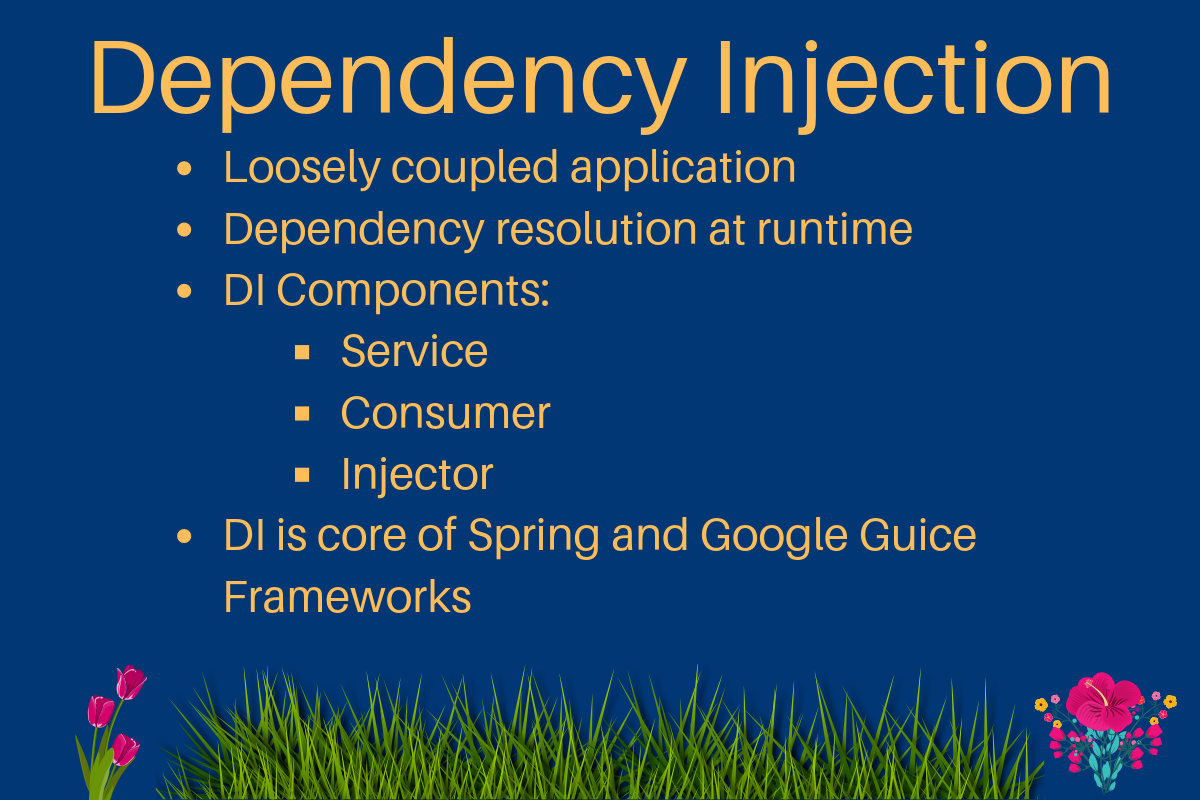
@Component(modules = {MyModule.class})
public interface MyComponent {
// Provide a dependency for the Foo class
Foo foo();
}
// Define the module that provides dependencies
@Module(injects = {Foo.class, Bar.class})
public class MyModule {
// Provide instances of Foo and Bar
@Provides
public Foo provideFoo() {
return new Foo();
}
@Provides
public Bar provideBar() {
return new Bar();
}
}
// Define the Foo class with dependencies
public class Foo {
private Bar bar;
@Inject
public Foo(Bar bar) {
this.bar = bar;
}
public void doSomething() {
// Use the injected bar dependency here
}
}
In this example:
We define aMyComponent
interface that provides dependencies using the @Component
annotation. The MyModule
class is the implementation of MyComponent
, providing instances of Foo
and Bar
. The Foo
class has an injected dependency on Bar
, which is provided by the MyModule
.
When you use a component to create an instance of the Foo
class, Dagger will automatically inject the Bar
dependency into it:
// Create a new instance of Foo using the MyComponent
Foo foo = MyComponent.builder().build().foo();
// Use the injected Foo instance
foo.doSomething();
In this scenario, you would see that the bar
field in the Foo
class is automatically initialized with an instance of Bar
provided by MyModule
.
This example demonstrates how @Inject
works in Java:
@Inject
annotation to indicate which variables or methods should receive injected dependencies. The dependency injection framework (Dagger) automatically resolves and injects the necessary dependencies into your code.
This pattern is widely used in Java-based applications, especially those with complex object graphs and multiple dependencies.
Does this help?