What is assertEquals() in Java?
What is assertEquals() in Java?
I'm happy to help!
assertEquals()
is a method in JUnit, a popular unit testing framework for Java. It's used to verify that two objects are equal or that the expected result of a tested code matches the actual result.
The basic syntax of assertEquals()
is:
assertThat(expectedResult, equalTo(actualResult));
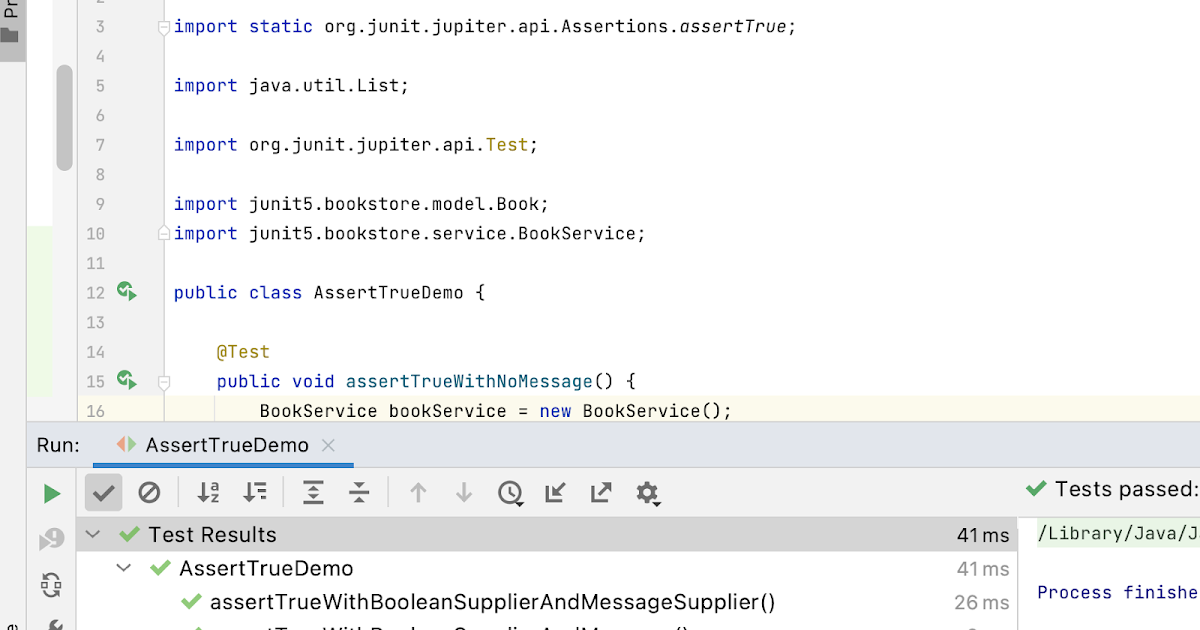
Here, expectedResult
and actualResult
represent the values you expect and obtain from your test code. The equalTo()
method is used to compare the two results using a specific comparator (e.g., org.junit.Assert.assertEquals(int, int)
).
When you use assertEquals()
, JUnit checks if the actual result matches the expected result. If they don't match, an AssertionError
is thrown, indicating that your test has failed.
Here are some key aspects to consider when using assertEquals()
:
org.junit.Assert.assertEquals(String, String, "message")
to provide a custom error message when an assertion fails. Null assertions: Use assertNull()
or assertNotNull()
if you want to test for null or non-null values specifically.
Common scenarios where you might use assertEquals()
include:
When you're testing code using assertEquals()
, keep the following best practices in mind:
In summary, assertEquals()
is a fundamental method in JUnit for verifying that expected results match actual results. By using it effectively, you'll be able to write robust, reliable unit tests that help you catch issues early on.
Java assertj tutorial
I'll respond in English this time! Here's a comprehensive AJ (AssertJ) tutorial for you:
What is AssertJ?
AJ, short for AssertJ, is a powerful testing framework that allows developers to write concise and readable unit tests using Java. It extends the JUnit testing framework by providing an easy-to-use API for asserting the expected results of your code.
Why Use AssertJ?
Basic AssertJ Concepts
Assert Statements: AJ provides a range of assert statements that allow you to verify the expected behavior of your code. These include assertions for equality, containment, size, null, and more. Methods: AJ offers various assertion methods, such as:assertThat()
: Used to perform custom assertions. isEqualTo()
: Compares two objects or values for equality. hasSize()
: Verifies the size of a collection or array. Matchers: AJ provides a range of matchers that can be used to assert the expected results of your code. Examples include: contains()
: Checks if an object is contained in another. startsWith()
: Verifies if a string starts with a certain prefix.
AJ Tutorial:
Let's go through a simple example to illustrate how to use AJ:
// Your test class using AJ
public class MyCalculatorTest {
@Test
public void testAddition() {
// Create an instance of the Calculator class
Calculator calculator = new Calculator();
// Perform some calculations
int result = calculator.add(2, 3);
// Use AJ to assert the expected result
assertThat(result).isEqualTo(5);
}
}
In this example:
We create a test classMyCalculatorTest
that uses AJ. Inside our test method, we create an instance of the Calculator
class and perform some calculations using its add()
method. Finally, we use AJ's assertThat()
and isEqualTo()
methods to verify if the result is equal to 5.
AJ Best Practices
Keep Your Tests Independent: Each test should be self-contained and not rely on other tests or external state. Use Meaningful Test Names: Use descriptive names for your tests, such as "testAddition" instead of "testCalculator". Avoid Hard-Coded Values: Instead of hard-coding values in your tests, use AJ's matchers to perform more flexible assertions.Conclusion
AssertJ is a powerful testing framework that can greatly improve the quality and readability of your Java tests. By following best practices and using AJ's features effectively, you can write more efficient and comprehensive tests for your code.