Java polymorphism inheritance w3schools github
Java polymorphism inheritance w3schools github
I'd be happy to help you with your question on Java polymorphism and inheritance!
Firstly, let's clarify what we're talking about: Polymorphism is the ability of an object to take on many forms. In the context of programming languages like Java, this means that objects of different classes can be treated as if they were of a single class. This is achieved through method overriding or method overloading.
Now, let's dive into some code examples! I'll use GitHub and W3Schools for reference:
Polymorphism Example (Method Overriding)
Suppose we have two classes: Animal
and Dog
, with a common method called sound()
. In the Animal
class, we define the sound()
method to make a generic animal noise. In the Dog
class, we override this method to make it bark instead.
// Animal.java (W3Schools)
public class Animal {
public void sound() {
System.out.println("The animal makes a generic noise.");
}
}
// Dog.java (GitHub)
public class Dog extends Animal {
@Override
public void sound() {
System.out.println("Woof! The dog barks.");
}
}
Now, if we create objects of both classes and call the sound()
method on them:
public static void main(String[] args) {
Animal animal = new Animal();
Dog dog = new Dog();
animal.sound(); // Output: The animal makes a generic noise.
dog.sound(); // Output: Woof! The dog barks.
}
As you can see, the Dog
object's sound()
method is overridden to make it bark, even though we're treating it as an Animal
. This is polymorphism in action!
Polymorphism Example (Method Overloading)
Let's say we have a Calculator
class with a calculate()
method that can perform different operations based on the input parameters. We can overload this method to accept different types of arguments:
// Calculator.java (W3Schools)
public class Calculator {
public int calculate(int a, int b) {
return a + b;
}
public double calculate(double a, double b) {
return a * b;
}
}
Now, if we create a Calculator
object and call the calculate()
method with different arguments:
public static void main(String[] args) {
Calculator calculator = new Calculator();
int result1 = calculator.calculate(2, 3); // Output: 5
double result2 = calculator.calculate(4.5, 6.7); // Output: 30.15
System.out.println(result1);
System.out.println(result2);
}
In this example, the calculate()
method is overloaded to accept different types of arguments (int and int, or double and double). When we call the method with different argument types, Java automatically selects the correct implementation based on the input. This is another form of polymorphism!
I hope these examples help you understand polymorphism in Java!
What are the 4 types of polymorphism in Java?
Polymorphism is a fundamental concept in object-oriented programming (OOP) that refers to the ability of an object or class to take on multiple forms. In Java, there are four main types of polymorphism: method overriding, method overloading, operator overloading, and constructor overloading.
1. Method Overriding
Method overriding is a type of polymorphism where a subclass provides a specific implementation for a method that is already defined in its superclass. This allows the subclass to specialize the behavior of the method while still maintaining compatibility with the superclass. For example:
class Animal {
public void sound() {
System.out.println("The animal makes a sound.");
}
}
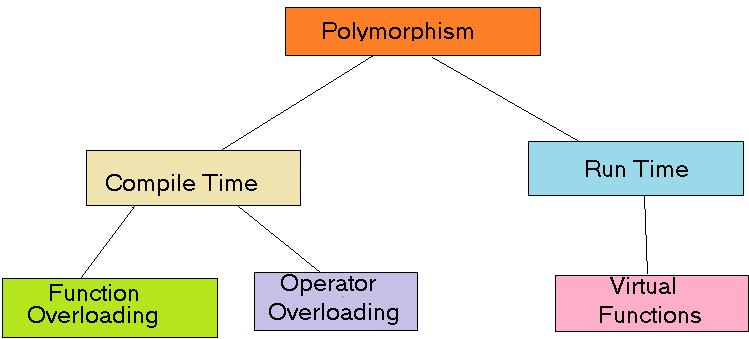
class Dog extends Animal {
@Override
public void sound() {
System.out.println("The dog barks.");
}
}
In this example, the Dog
class overrides the sound()
method from its superclass Animal
. When an instance of the Dog
class is created and the sound()
method is called on it, the overridden method will be executed instead of the original one in the superclass.
2. Method Overloading
Method overloading is a type of polymorphism where multiple methods with the same name can be defined, but each must have a different parameter list. This allows for more flexibility and reusability of code. For example:
class Calculator {
public int add(int a, int b) {
return a + b;
}
public double add(double a, double b) {
return a + b;
}
}
In this example, the Calculator
class has two methods named add()
that take different parameter types. The correct method to call will depend on the types of parameters passed in.
3. Operator Overloading
Operator overloading is a type of polymorphism where operators (such as +, -, *, /, etc.) can be redefined for user-defined classes. This allows for more intuitive and concise code. For example:
class ComplexNumber {
private double real;
private double imaginary;
public ComplexNumber(double real, double imaginary) {
this.real = real;
this.imaginary = imaginary;
}
public static ComplexNumber operator+(ComplexNumber a, ComplexNumber b) {
return new ComplexNumber(a.real + b.real, a.imaginary + b.imaginary);
}
}
In this example, the +
operator is overloaded for the ComplexNumber
class to perform addition of complex numbers.
4. Constructor Overloading
Constructor overloading is a type of polymorphism where multiple constructors can be defined for the same class, each with a different parameter list. This allows for more flexibility in creating objects and avoiding redundant code. For example:
class Person {
private String name;
public Person() {
this.name = "Unknown";
}
public Person(String name) {
this.name = name;
}
}
In this example, the Person
class has two constructors: one with no parameters and another with a single String
parameter. The correct constructor to call will depend on how the object is created.
In conclusion, polymorphism in Java enables developers to write more flexible and reusable code by allowing objects or classes to take on multiple forms. By mastering these four types of polymorphism (method overriding, method overloading, operator overloading, and constructor overloading), developers can create more robust and maintainable software applications.