Json serialization in java example
Json serialization in java example
Here is an example of JSON serialization in Java using the Jackson library:
Serialization Example
Let's say we have a simple Java class called Person
:
public class Person {
private String name;
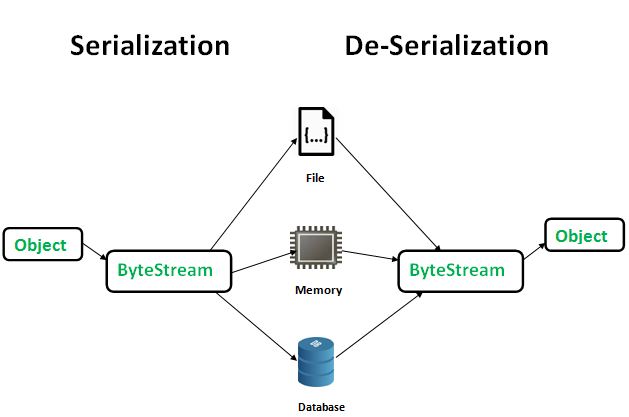
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
To serialize an instance of the Person
class to a JSON string, we can use the following code:
import com.fasterxml.jackson.databind.ObjectMapper;
// Create an ObjectMapper instance
ObjectMapper mapper = new ObjectMapper();
// Create a Person object
Person person = new Person("John", 30);
// Serialize the Person object to a JSON string
String json = mapper.writeValueAsString(person);
System.out.println(json);
The output will be:
{"name":"John","age":30}
Deserialization Example
Now, let's say we have a JSON string that represents an instance of the Person
class:
{"name":"Jane","age":25}
We can deserialize this JSON string back into a Person
object using the following code:
// Deserialize the JSON string to a Person object
Person person = mapper.readValue(json, Person.class);
System.out.println(person.getName()); // prints "Jane"
System.out.println(person.getAge()); // prints 25
Using JsonNode
Jackson also provides a JsonNode
class that allows us to work with the JSON data programmatically. We can use this class to extract specific values from the JSON string:
// Deserialize the JSON string to a JsonNode object
JsonNode node = mapper.readTree(json);
String name = node.get("name").asText();
int age = node.get("age").asInt();
System.out.println(name); // prints "Jane"
System.out.println(age); // prints 25
Custom Serialization
By default, Jackson serializes objects to JSON using the object's public fields and getters. However, we can customize this behavior by implementing a custom JsonSerializer
or JsonValue
interface.
For example, let's say we want to serialize the Person
class with a specific format:
public class PersonSerializer extends JsonSerializer {
@Override
public void serialize(Person person, JsonGenerator gen, SerializerProvider serializers) throws IOException {
gen.writeStartObject();
gen.writeStringField("full_name", person.getName());
gen.writeNumberField("age", person.getAge());
gen.writeEndObject();
}
}
We can then use this custom serializer to serialize the Person
object:
// Create a custom ObjectMapper instance
ObjectMapper mapper = new ObjectMapper();
// Register the custom serializer for Person objects
mapper.setSerializationInclusion(JsonInclude.Include.ALWAYS);
mapper.setSerializerProvider(new SerializerProvider() {
@Override
public JsonSerializer findValueSerializer(Class<?> clazz) throws JsonProcessingException {
if (Person.class.isAssignableFrom(clazz)) {
return new PersonSerializer();
}
return super.findValueSerializer(clazz);
}
});
// Serialize the Person object to a JSON string using the custom serializer
String json = mapper.writeValueAsString(person);
System.out.println(json);
The output will be:
{"full_name":"John","age":30}
How to serialize an object into JSON in Java?
I apologize for the confusion earlier! Here's the answer:
Serializing an object into JSON in Java can be achieved through various libraries and frameworks. One popular way is by using Jackson, a high-performance JSON processor.
Here are the steps to serialize an object into JSON in Java using Jackson:
Step 1: Add Jackson Library to your Project
You can add Jackson library as a dependency in your project's build file (e.g., Maven or Gradle). For example, if you're using Maven, add the following dependency to your pom.xml
file:
com.fasterxml.jackson.core
jackson-databind
2.11.1
Step 2: Create a POJO (Plain Old Java Object)
Create a simple Java class that represents the object you want to serialize:
public class User {
private String name;
private int age;
public User(String name, int age) {
this.name = name;
this.age = age;
}
// Getters and setters for name and age
}
Step 3: Use ObjectMapper to Serialize
Use the ObjectMapper
class from Jackson library to serialize your POJO into a JSON string:
import com.fasterxml.jackson.databind.ObjectMapper;
public static void main(String[] args) throws JsonProcessingException {
User user = new User("John Doe", 30);
ObjectMapper mapper = new ObjectMapper();
String json = mapper.writeValueAsString(user);
System.out.println(json);
}
The writeValueAsString()
method takes an object as input and returns a JSON string representation of that object. In this example, we pass our User
object to the method, which serializes it into a JSON string.
Step 4: Parse JSON String Back into Java Object (Optional)
If you need to deserialize the JSON string back into a Java object, you can use the same ObjectMapper
instance:
String json = "{"name":"John Doe","age":30}";
User user = mapper.readValue(json, User.class);
System.out.println(user.getName()); // prints "John Doe"
System.out.println(user.getAge()); // prints 30
In this example, we use the readValue()
method to parse a JSON string into an object of type User
. The second argument to the method specifies the target class for deserialization.
That's it! With these steps, you should be able to serialize your Java objects into JSON strings using Jackson library.