What does super() do in Java?
What does super() do in Java?
I'd be happy to explain what super()
does in Java!
In Java, the super
keyword is used to refer to the superclass or parent class of an object. This can be particularly useful when overriding methods or accessing variables from a superclass.
When you override a method in a subclass, you have the option to call the original (or "super") method using the super()
constructor. For example:
public class Animal {
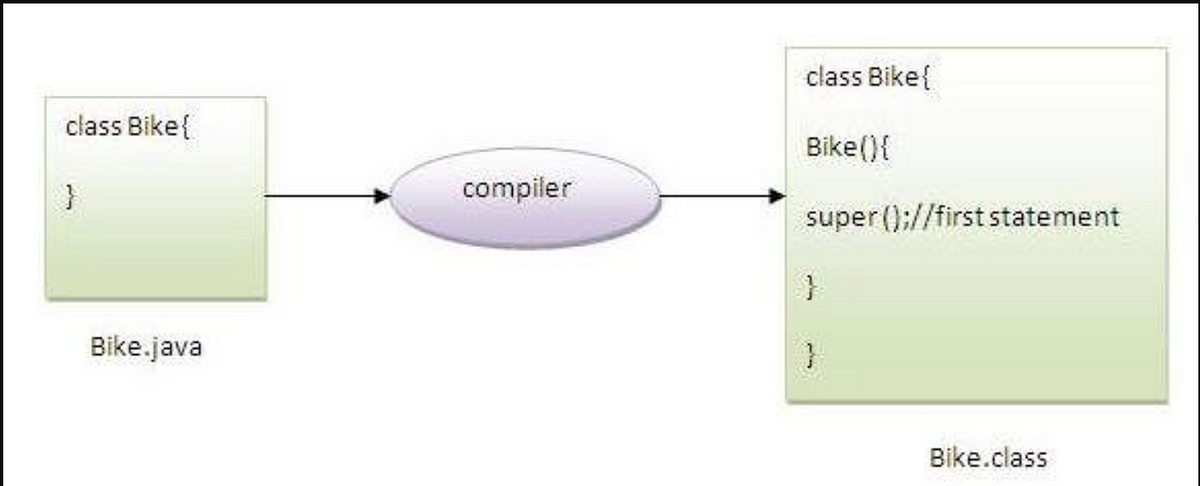
public void makeSound() {
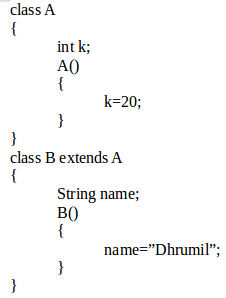
System.out.println("Animal makes a sound");
}
}
public class Dog extends Animal {
@Override
public void makeSound() {
super().makeSound(); // Calls the original Animal.makeSound method
System.out.println("Dog barks");
}
}
In this example, when you create an instance of Dog
and call its makeSound()
method, it will first print "Animal makes a sound" (the superclass's implementation), then print "Dog barks". This is because the super()
constructor was used to invoke the original method.
It's worth noting that the super
keyword can also be used to access variables or methods from the parent class. For instance:
public class Animal {
public int age = 0;
}
public class Dog extends Animal {
@Override
public void growOlder() {
super.age++; // Accesses and increments the age variable from Animal
}
}
In this case, when you create a Dog
object and call its growOlder()
method, it will increment the age
variable that is inherited from Animal
.
Another important aspect of the super
keyword is its use in constructor calls. When you override a constructor in a subclass, you can use super()
to invoke the constructor of the parent class, passing in any necessary arguments. For example:
public class Animal {
public Animal(String name) {
this.name = name;
}
}
public class Dog extends Animal {
public Dog(String name, int age) {
super(name); // Calls the Animal constructor with the provided name
this.age = age;
}
}
In this scenario, when you create a Dog
object and pass in its name and age, it will first call the Animal
constructor to initialize the name
variable, then set its own age
property.
In summary, super()
is a powerful keyword in Java that allows you to access and invoke methods or variables from a superclass, which can be useful for overriding behavior, accessing inherited state, or calling parent-class constructors.
Parameterized constructor in Java
I'll respond in English for you!
Parameterized Constructor in Java
In object-oriented programming (OOP), a constructor is a special method that is called when an object of a class is created. In Java, constructors are used to initialize objects and perform any necessary setup or configuration. By default, constructors have the same name as the class they belong to, and they do not return any value.
Parameterized Constructor
A parameterized constructor in Java is a constructor that takes one or more parameters (also known as arguments) when it's called. The purpose of these constructors is to allow the caller to pass values for specific attributes or properties of the object being created.
Here's an example of how you might define a simple class with a parameterized constructor:
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
// Other methods and attributes go here...
}
In this example, the Person
class has two attributes: name
and age
. The constructor takes these two parameters when it's called:
public Person(String name, int age) {
this.name = name;
this.age = age;
}
To create a new Person
object with specific values for name
and age
, you would call the constructor like this:
Person person1 = new Person("John", 30);
By passing "John"
as the value for name
and 30
as the value for age
, we're creating a new Person
object with those specific attributes. This is an example of how parameterized constructors can be used to initialize objects with custom values.
Benefits of Parameterized Constructors
Parameterized constructors provide several benefits, including:
Customization: By passing different values as parameters, you can create objects that have unique configurations. Reusability: You don't need to create a separate constructor for each possible combination of attributes. Improved Code Readability: The code is more readable because it's clear what the constructor expects in terms of input.Common Use Cases
Parameterized constructors are commonly used in scenarios where objects need to be initialized with specific data, such as:
User Input: When a user provides input (e.g., name, age, address) that needs to be used to initialize an object. Database Data: When data is retrieved from a database or another source and needs to be used to create objects.In summary, parameterized constructors in Java are powerful tools for customizing the initialization of objects. They allow you to pass specific values as parameters when creating new objects, which can greatly simplify your code and improve its readability.