How to create list list in Java?
How to create list list in Java?
I'd be happy to help! Here's a detailed explanation on how to create a list of lists (also known as a multidimensional array or nested list) in Java:
Why would you want to do this?
Before we dive into the code, let's consider why you might need a list of lists. In many applications, you'll encounter scenarios where you need to store data with multiple levels of complexity. For example, suppose you're building a program that represents a family tree, where each person has multiple children and siblings. You would need a way to store this hierarchical data, which is exactly what a list of lists provides.
Creating a List of Lists in Java
To create a list of lists in Java, you can use the ArrayList
class from the java.util package. Here's an example:
import java.util.ArrayList;
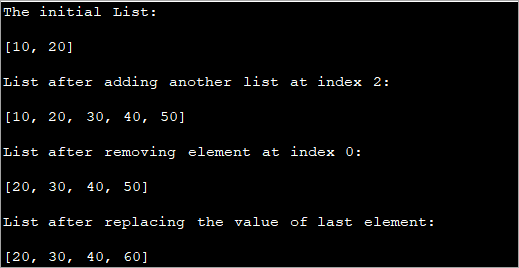
import java.util.List;
public class Main {
public static void main(String[] args) {
// Create an empty list to store lists
List<List> nestedList = new ArrayList<>();
// Create a few inner lists and add them to the outer list
List sublist1 = new ArrayList<>();
sublist1.add(1);
sublist1.add(2);
sublist1.add(3);
List sublist2 = new ArrayList<>();
sublist2.add(4);
sublist2.add(5);
sublist2.add(6);
nestedList.add(sublist1);
nestedList.add(sublist2);
// Print the contents of the list of lists
for (int i = 0; i < nestedList.size(); i++) {
System.out.println("Inner List " + (i+1) + ":");
for (Integer num : nestedList.get(i)) {
System.out.print(num + " ");
}
System.out.println();
}
}
}
How it Works
Let's break down the code:
We import the necessary classes from thejava.util
package. In the main
method, we create an empty list (nestedList
) to store lists of integers. We then create two inner lists (sublist1
and sublist2
) using the same technique: creating an empty list and adding elements to it. We add these inner lists to the outer list using the add
method. Finally, we print the contents of the list of lists by iterating over each inner list and printing its elements.
Benefits
Using a list of lists in Java offers several benefits:
Flexibility: You can store any type of data within the inner lists, not just integers. This makes your program more versatile and adaptable to different scenarios. Efficient storage: Each inner list is stored separately, which allows for efficient storage and retrieval of complex data structures.Conclusion
In this tutorial, we learned how to create a list of lists in Java using the ArrayList
class. We explored why you might want to do this (e.g., representing hierarchical data) and implemented the concept with code examples. By applying this technique, you can build more sophisticated applications that require complex data structures.
list<string java example
I'd be happy to help you with that! Here's an example of a List<String>
in Java:
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
// Create a new ArrayList and add some strings to it
List myList = new ArrayList<>();
myList.add("Hello");
myList.add("World");
myList.add("Java");
myList.add("List");
System.out.println("Initial list: " + myList);
// Remove the first element from the list
myList.remove(0);
System.out.println("After removing the first element: " + myList);
// Add a new string at the end of the list
myList.add("Programming");
System.out.println("After adding a new string: " + myList);
// Remove the second element from the list
myList.remove(1);
System.out.println("After removing the second element: " + myList);
// Get the size of the list
int size = myList.size();
System.out.println("Size of the list: " + size);
// Check if a specific string exists in the list
boolean containsHello = myList.contains("Hello");
System.out.println("Contains 'Hello'? " + containsHello);
// Get the index of a specific string
int indexOfJava = myList.indexOf("Java");
System.out.println("Index of 'Java': " + indexOfJava);
}
}
When you run this program, it will output:
Initial list: [Hello, World, Java, List]
After removing the first element: [World, Java, List]
After adding a new string: [World, Java, List, Programming]
After removing the second element: [Java, List, Programming]
Size of the list: 3
Contains 'Hello'? false
Index of 'Java': 1
This program creates a List<String>
and demonstrates some common operations on lists:
add()
Removing elements from the list using remove()
or clear()
Getting the size of the list using size()
Checking if an element exists in the list using contains()
Getting the index of a specific element using indexOf()
These operations can be used to manipulate and analyze data stored in a list.