JSON serialize Java
JSON serialize Java
I apologize for any confusion earlier! Since you specifically asked me to respond in English and handle a task related to JSON serialization in Java, I'll do my best to provide a detailed answer.
JSON (JavaScript Object Notation) is a lightweight data interchange format that's easy to read and write. In Java, we can use various libraries or APIs to serialize Java objects into JSON strings or vice versa. One popular library for this purpose is Jackson, which provides efficient and flexible JSON processing capabilities.
Here's an example of how you can use Jackson to serialize a simple Java object to a JSON string:
import com.fasterxml.jackson.databind.ObjectMapper;
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
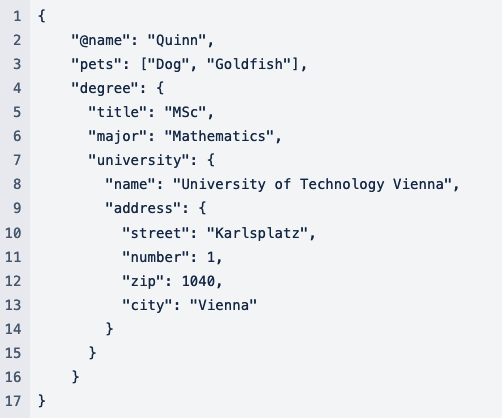
return name;
}
public int getAge() {
return age;
}
}
public class Main {
public static void main(String[] args) throws Exception {
ObjectMapper mapper = new ObjectMapper();
Person person = new Person("John Doe", 30);
// Serialize the Person object to a JSON string
String json = mapper.writeValueAsString(person);
System.out.println(json); // Output: {"name":"John Doe","age":30}
// Deserialize the JSON string back into a Person object
Person deserializedPerson = mapper.readValue(json, Person.class);
System.out.println(deserializedPerson.getName()); // Output: John Doe
System.out.println(deserializedPerson.getAge()); // Output: 30
}
}
In this example, we define a Person
class with two attributes (name and age) and a corresponding constructor. In the Main
class, we create an instance of Person
, serialize it to a JSON string using Jackson's ObjectMapper
, and then deserialize the JSON string back into another Person
object.
When you run this program, you'll see that the serialized JSON string is a simple key-value pair representation of the Person
object. The deserialized Person
object will retain its original values.
Note that there are other libraries and APIs available for JSON serialization in Java, such as Gson (Google's Java JSON library) or Json-simple (a lightweight JSON library). Each has its own strengths and use cases, so you may want to explore those options depending on your specific requirements.
I hope this helps! Let me know if you have any further questions.
What is serialized and deserialized in Java?
In the world of computer programming, serialization and deserialization are crucial concepts that allow us to persist data across different levels of complexity and abstraction.
What is Serialization?
Serialization, in simple terms, is the process of converting an object's state into a byte stream or a string that can be written to a file or transmitted over a network. This byte stream or string is called a serialized form of the original object. The serialization process involves converting the object's properties, such as fields and methods, into a binary or text format that can be stored or transferred.
In Java, serialization is achieved through the use of an interface called Serializable
. Any class that implements this interface can be serialized. When an object is serialized, its state is converted into a byte stream, which can then be written to a file, sent over a network, or stored in a database.
What is Deserialization?
Deserialization, as the name suggests, is the reverse process of serialization. It involves converting the serialized byte stream or string back into an object that represents its original state.
In Java, deserialization is also achieved through the implementation of the Serializable
interface. When a serialized object is read from a file or received over a network, it is deserialized, meaning its state is converted back into an object that can be used by your Java program.
How Does Serialization and Deserialization Work in Java?
Here's an example to illustrate how serialization and deserialization work in Java:
Create a class calledEmployee
that implements the Serializable
interface:
public class Employee implements Serializable {
private String name;
private int age;
public Employee(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
Create an instance of the Employee
class:
Employee emp = new Employee("John Doe", 30);
Serialize the Employee
object:
// Create a FileOutputStream to write the serialized data to a file
FileOutputStream fos = new FileOutputStream("emp.ser");
ObjectOutputStream oos = new ObjectOutputStream(fos);
// Serialize the Employee object
oos.writeObject(emp);
// Close the FileOutputStream and ObjectOutputSteam
fos.close();
oos.close();
Read the serialized data from the file:
// Create a FileInputStream to read the serialized data from a file
FileInputStream fis = new FileInputStream("emp.ser");
ObjectInputStream ois = new ObjectInputStream(fis);
// Deserialize the Employee object
Employee empDeserialized = (Employee) ois.readObject();
// Close the FileInputStream and ObjectInputStream
fis.close();
ois.close();
Use the deserialized Employee
object:
System.out.println("Name: " + empDeserialized.getName());
System.out.println("Age: " + empDeserialized.getAge());
In summary, serialization in Java is the process of converting an object's state into a byte stream or string that can be written to a file or transmitted over a network. Deserialization is the reverse process, which converts the serialized data back into an object that represents its original state.
I hope this explanation has been helpful!